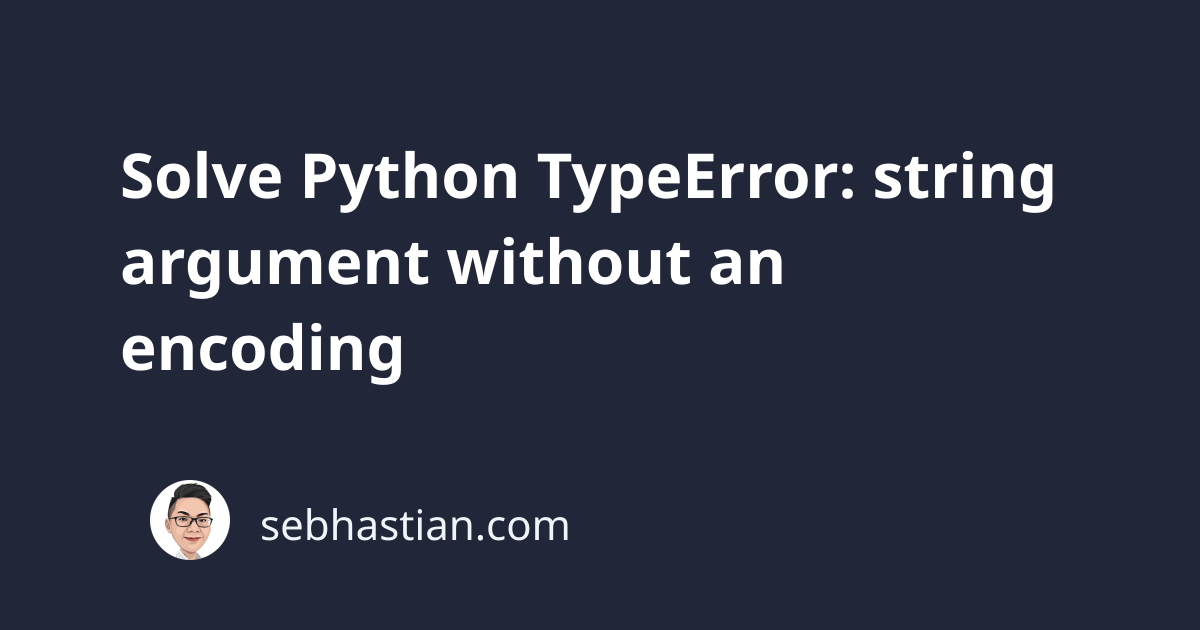
Python shows TypeError: string argument without an encoding
when you pass a string argument to a function or method that expects an encoded string, but you have not specified the encoding
for that string.
To solve this error, you need to specify the encoding of the string argument.
This usually happens when you create a bytes
object using the bytes()
function.
Suppose you run the following code:
b_object = bytes("Hello")
The bytes()
function is called to convert the string Hello
into a bytes object, but you didn’t specify the encoding
of that string.
This causes Python to respond with an error:
Traceback (most recent call last):
File ...
b_object = bytes("Hello")
TypeError: string argument without an encoding
You need to pass the encoding of the string as follows:
b_object = bytes("Hello", "utf-8") # ✅
print(b_object) # b'Hello'
The same error also occurs when you create an array of bytes using the bytearray()
function:
b_array = bytearray("Hello") # ❗️TypeError
b_array = bytearray("Hello", "utf-8") # ✅
print(b_array) # bytearray(b'Hello')
You can also specify the encoding
as a named argument like this:
b_object = bytes("Hello", encoding="utf-8")
b_array = bytearray("Hello", encoding="utf-8")
You need to specify the encoding only when you create a bytes object from a string.
When you pass another value type like an integer, you don’t need to specify the encoding:
b_obj = bytes(1)
print(b_obj) # b'\x00'
b_array = bytearray(2)
print(b_array) # bytearray(b'\x00\x00')
This error happens only in Python 3 because the bytes
class was updated to always request an encoding
argument for a string.
In Python 2, the bytes()
function works inconsistently as follows:
res = bytes(u"abc") # ✅
res = bytes(u"\xff") # ❗️UnicodeEncodeError
To help you avoid the error shown above, Python 3 changed the way the bytes()
and bytearray()
functions work.
They now require an encoding to be specified when you pass a string.
Conclusion
The Python TypeError: string argument without an encoding
occurs when you pass a string argument to a function or method that requires the encoding to be specified.
To solve this error, pass the encoding
value to the function as an argument.