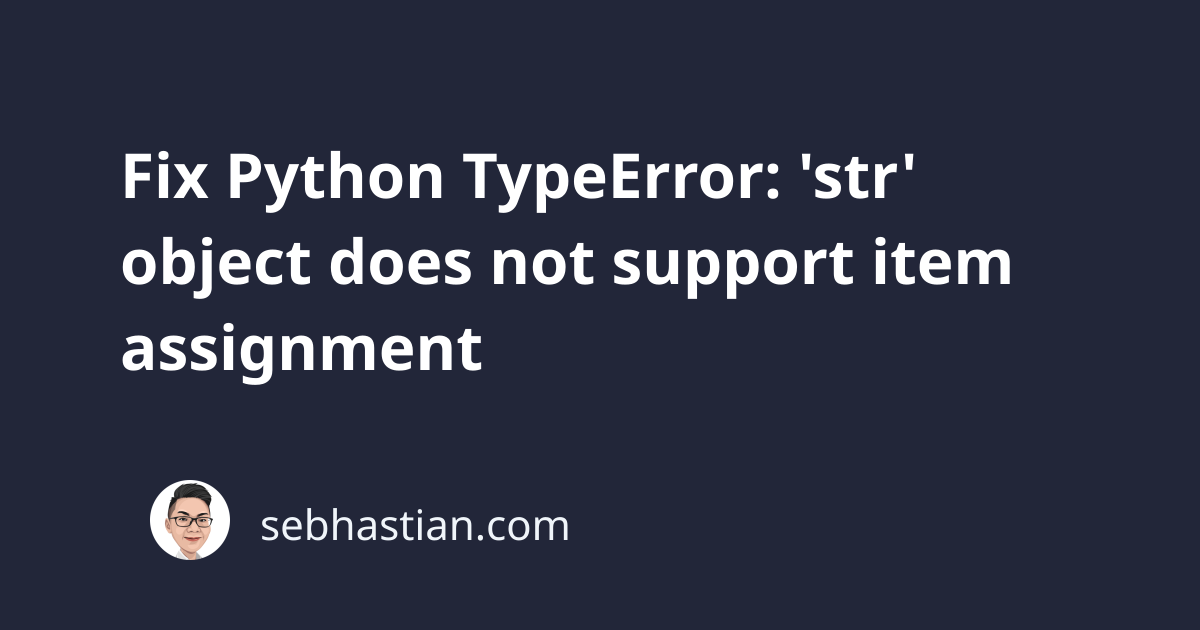
Python shows TypeError: 'str' object does not support item assignment
error when you try to access and modify a string object using the square brackets ([]
) notation.
To solve this error, use the replace()
method to modify the string instead.
This error occurs because a string in Python is immutable, meaning you can’t change its value after it has been defined.
For example, suppose you want to replace the first character in your string as follows:
greet = "Hello, world!"
greet[0] = 'J'
The code above attempts to replace the letter H
with J
by adding the index operator [0]
.
But because assigning a new value to a string is not possible, Python responds with the following error:
Traceback (most recent call last):
File ...
greet[0] = 'J'
TypeError: 'str' object does not support item assignment
To fix this error, you can create a new string with the desired modifications, instead of trying to modify the original string.
This can be done by calling the replace()
method from the string. See the example below:
old_str = 'Hello, world!'
# Replace 'H' with 'J'
new_str = old_str.replace('H', 'J')
print(new_str) # Jello, world!
The replace()
method allows you to replace all occurrences of a substring in your string.
This method accepts 3 parameters:
old
- the substring you want to replacenew
- the replacement forold
valuecount
- how many times to replaceold
(optional)
By default, the replace()
method replaces all occurrences of the old
string:
old_str = 'Hello, world!'
# Replace 'l' with 'x'
new_str = old_str.replace('l', 'x')
print(new_str) # Hexxo, worxd!
You can control how many times the replacement occurs by passing the third count
parameter.
The code below replaces only the first occurrence of the old
value:
old_str = 'Hello, world!'
# Replace 'l' with 'x'
new_str = old_str.replace('l', 'x', 1)
print(new_str) # Hexlo, world!
And that’s how you can modify a string using the replace()
method.
If you want more control over the modification, you can use a list.
Convert the string to a list first, then access the element you need to change as shown below:
greet = "Hello, world!"
# Convert the string into a list
str_as_list = list(greet)
# Replace 'H' with 'J'
str_as_list[0] = 'J'
# Merge the list as string with join()
new_string = "".join(str_as_list)
print(new_string) # Jello, world!
After you modify the list element, merge the list back as a string by using the join()
method.
This solution gives you more control as you can select the character you want to replace. You can replace the first, middle, or last occurrence of a specific character.
Another way you can modify a string is to use the string slicing and concatenation method.
Consider the two examples below:
old_str = "Hello, world!"
# Slice the old_str from index 1 to finish
# Add the letter 'J' in front of the string
new_str = 'J' + old_str[1:]
print(new_str) # Jello, world!
# Change the letter 'w' to 'x'
# slice the old_str twice and concatenate them
new_str = string = old_str[:7] + 'x' + old_str[8:]
print(new_str) # Hello, xorld!
In both examples, the string slicing operator is used to extract substrings of the old_str
variable.
In the first example, the slice operator is used to extract the substring starting from index 1 to the end of the string with old_str[1:]
and concatenates it with the character ‘J’ .
In the second example, the slice operator is used to extract the substring before index 7 with old_str[:7]
and the substring after index 8 with old_str[8:]
syntax.
Both substrings are joined together while putting the character x
in the middle.
The examples show how you can use slicing to extract substrings and concatenate them to create new strings.
But using slicing and concatenation can be more confusing than using a list, so I would recommend you use a list unless you have a strong reason.
Conclusion
The Python error TypeError: 'str' object does not support item assignment
occurs when you try to modify a string object using the subscript or index operator assignment.
This error happens because strings in Python are immutable and can’t be modified.
The solution is to create a new string with the required modifications. There are three ways you can do it:
- Use
replace()
method - Convert the string to a list, apply the modifications, merge the list back to a string
- Use string slicing and concatenation to create a new string
Now you’ve learned how to modify a string in Python. Nice work!