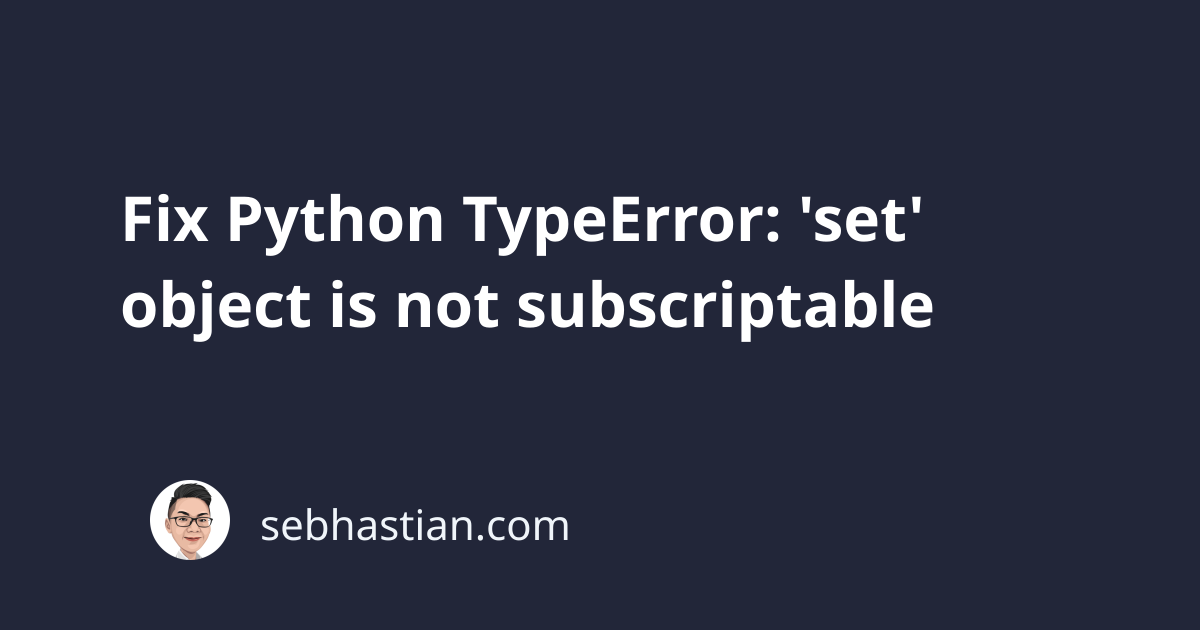
Python shows TypeError: 'set' object is not subscriptable
error when you use the square brackets []
notation to access elements of a set object.
To fix this error, you’ll need to convert the set object to a list or a tuple first.
In Python, sets are unordered collections of unique elements. They are defined using curly braces {}
or the set()
constructor.
A set is not a subscriptable object, so when you try to access its element like this:
s = {1, 2, 3}
print(s[0]) # ❌
Python responds with TypeError: 'set' object is not subscriptable
:
Traceback (most recent call last):
File ...
my_set[0]
TypeError: 'set' object is not subscriptable
Python sets are not subscriptable, meaning they do not support access to elements using square brackets (the subscript notation).
When you need to access an element of a set using an index, you’ll need to convert it to a list or a tuple first as follows:
s = {1, 2, 3}
# Convert a Python set to a list
my_list = list(s)
print(my_list[0]) # 1 ✅
# Convert a Python set to a tuple
my_tuple = tuple(s)
print(my_tuple[0]) # 1 ✅
If you have no code that requires a set and you have access to the code (not imported from a module) then you can declare the variable as a list directly.
Use square brackets to declare a list as shown below:
s = [1, 2, 3] # This is a list
print(s[0]) # 1 ✅
It is important to be aware of the differences between sets and other collection types like lists and tuples so that you use the appropriate methods for working with sets in your code.
Conclusion
The Python TypeError: 'set' object is not subscriptable
error occurs when you attempt to access elements of a set using square brackets.
The error can be fixed by converting the set to a list or tuple first, then access the specific element using square brackets.
Alternatively, you can declare the set as a list if you don’t require a set and you have access to the code.