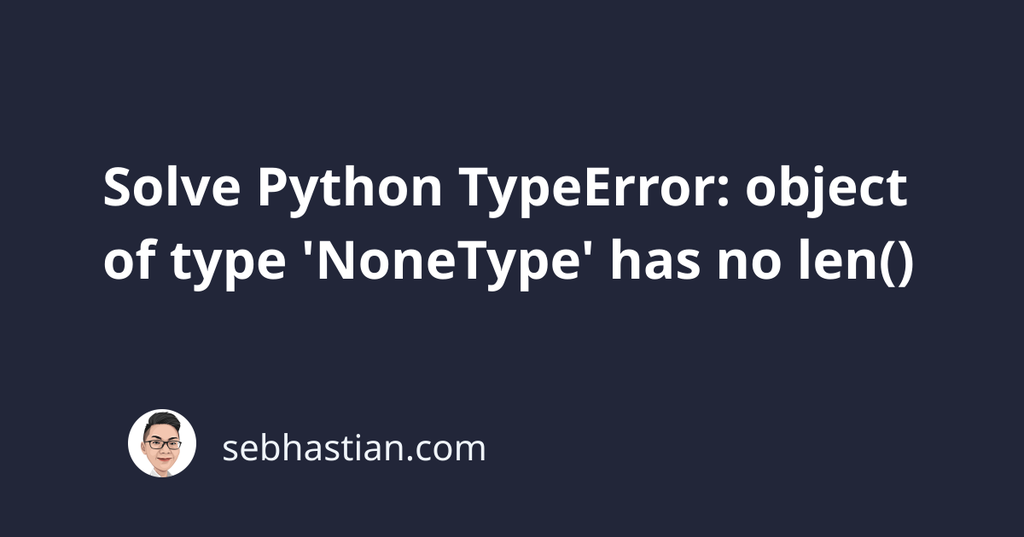
The “TypeError: object of type ‘NoneType’ has no len()” error in Python occurs when you try to call the len
function on an object of type NoneType.
NoneType
is a special type in Python that represents the absence of a value or a null
value. It is an object of its own type, and it is an instance of the None
class.
The len()
function is used to return the length of a sequence, such as a string, list, or tuple. But the function can’t be called on objects of type NoneType
, because they do not have a length.
Several built-in functions in Python returns None
by default. Here’s an example:
result = print("Hello")
print(result) # None
print(len(result)) # ❗️TypeError: 'NoneType' has no len()
The print
function in Python doesn’t return anything, so the result
variable in the code above will contain a None
value.
The code will show the TypeError as follows:
To fix or avoid this error from occurring, you need to make sure that you are not trying to call len()
on an object of type None
.
You can do this by checking the type of the object before calling len()
on it. You can use an if .. is ..
statement as shown below:
x = None
if x is None:
print("x is NoneType so it has no len()")
else:
print(len(x))
The None
value can be set explicitly as in the example above, or you can get it from functions that you called in your code.
Another common case of calling len()
on a NoneType object is when you assign the return value of Python built-in functions, like sort()
and shuffle()
.
Both sort()
and shuffle()
mutate the original list object, so these functions return None
:
import random
list_a = ["z", "x", "c"]
result = random.shuffle(list_a)
if result is None:
print("result is NoneType, it has no len()") # ✅
else:
print(len(result))
The code above will print “result is NoneType, it has no len()”.
You will also get the same TypeError when you call a custom function that has no return
statement.
Suppose you have a function that sorts a list of dictionaries by name key as shown below:
def sort_by_name(users):
users.sort(key=lambda x: x["name"])
The function above calls the sort()
function, but there’s no return value.
When you call the function, the return will be None
as follows:
users = [
{"name": "Nathan", "age": 28},
{"name": "Susan", "age": 27},
{"name": "Eric", "age": 31},
{"name": "Miley", "age": 22},
]
new_users = sort_by_name(users)
print(len(new_users)) # ❗️TypeError: 'NoneType' has no len()
To solve the error, you need to return the users
variable in the sort_by_name
function like this:
def sort_by_name(users):
users.sort(key=lambda x: x["name"])
return users
With the return
statement, the sorted list will be available for you to assign to the new_users
variable. This enables the len
function to get the length of the list.
And that’s how you fix the Python TypeError: object of type NoneType has no len() issue. Keep up the good work! 👍