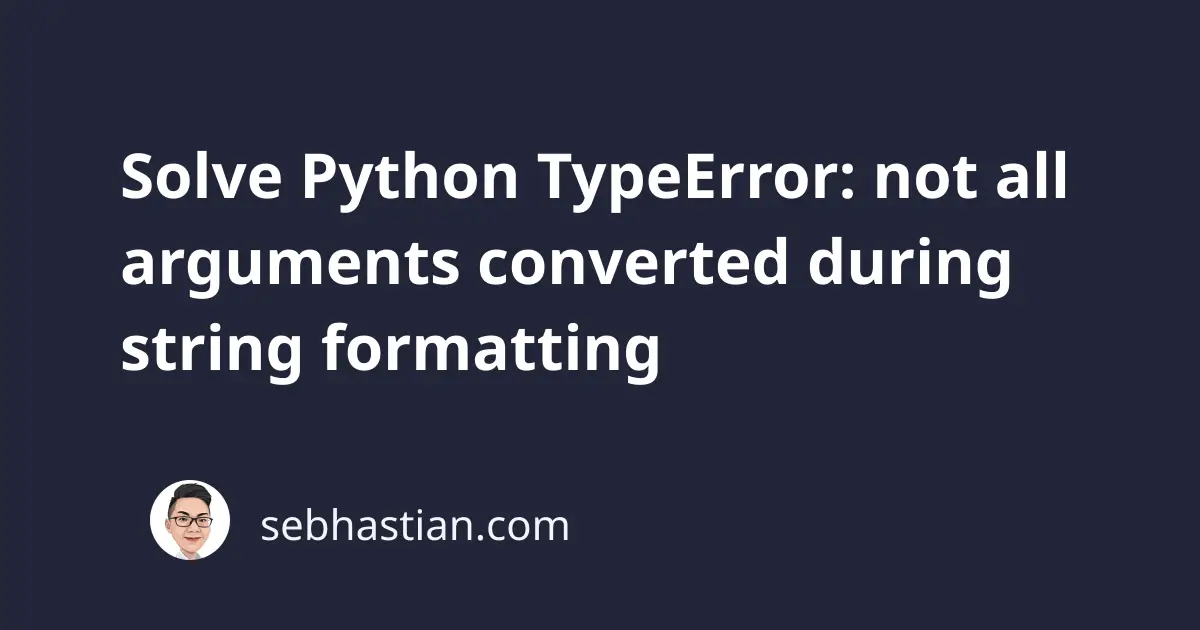
The TypeError: not all arguments converted during string formatting error in Python occurs when you mix the new string placeholders {}
with the old modulo %
operator.
String formatting allows you to insert dynamic values into a string by using special placeholders called format specifiers.
There are two ways to format a string with Python as follows:
# new string formatter using {} and format()
print("Hello, my name is {}.".format("Nathan"))
# old string formatter using %s and % operator
print("Hello, my name is %s." % ("Nathan"))
Both ways to format above still work in Python, but you must not mix them up or the TypeError will occur.
Here’s an example of the formatter that causes the error:
# mix {} with the % operator
print("Hello, my name is {}." % ("Nathan")) # ❗️ TypeError
When you run the code above, Python shows the error:
To fix this error, you need to make sure that you are using the right format:
- The curly brackets format
{}
with theformat()
function - The specifier format
%s
with the modulo%
operator
When the format is right, the error should be fixed.
Case #2: Using modulo with string and int type data
This error also occurs when you use the modulo operator to calculate the remainder of a number.
When the left operand is a string type, Python will throw the same error:
print("55" % 2)
# ❗️ TypeError: not all arguments converted during string formatting
When you have a string type, you need to convert it to an integer using the int()
function:
# 👇 convert string to int first
result = int("55") % 2
print(result) # 1
Conclusion
To conclude, the TypeError: not all arguments converted during string formatting occurs in Python when you use the modulo %
operator with strings in a way that Python can’t understand.
There are two common cases when this error occurs:
- When you mix the new and old string format specifiers
- When you calculate the remainder of a number on a string and int type data
To fix this error, you can follow the steps shown in this article.
I hope this post has been useful to you. 🙏