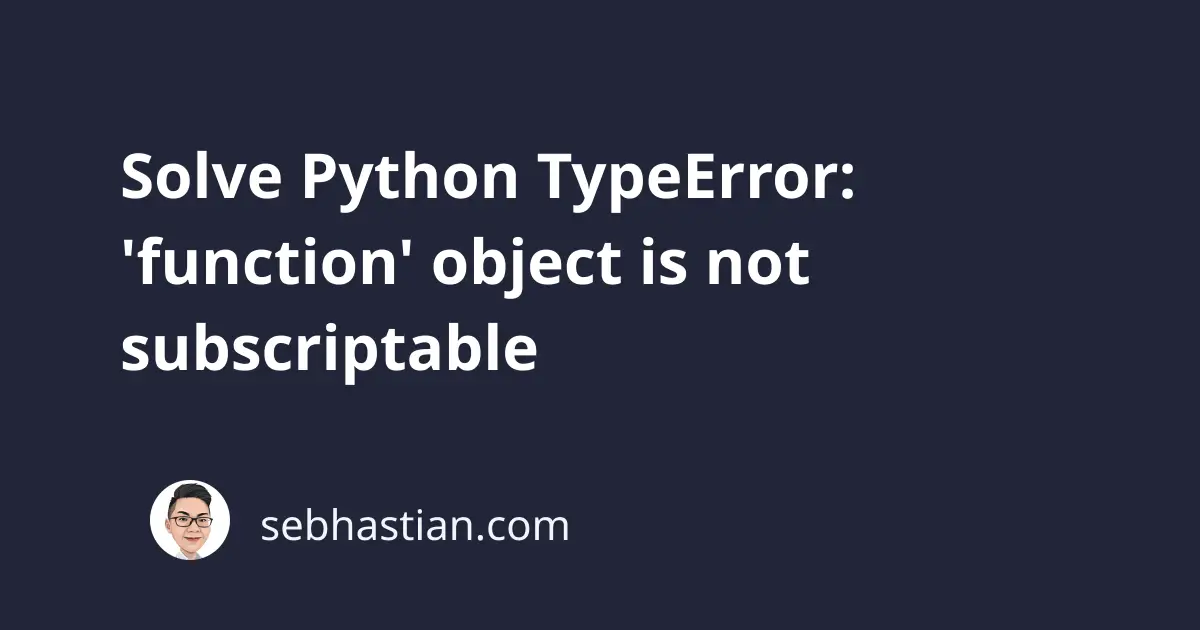
Python message TypeError: ‘function’ object is not subscriptable means that you are trying to call a function using square brackets.
To solve this error, you need to make sure that you are calling a function using the round brackets ()
There are two common cases where this error can happen:
This tutorial shows you how to fix the two cases above.
Case #1 Calling a function using square brackets
In Python, square brackets are used for accessing an element contained in a string, a list, a tuple, or a key in a dictionary.
The following code shows how to access an element of a string:
str = "Nathan"
# Access str element at index 0
print(str[0]) # N
# Access str element at index 2
print(str[2]) # t
When you use the square brackets to call a function, Python responds with the TypeError message.
Consider the example below:
def study(subject):
print("Learning", subject)
study['Python']
# 👆 TypeError: 'function' object is not subscriptable
The code above defines a function named study
that takes a subject
as an argument and prints a message.
When we try to call the function with the square brackets notation, we get the message TypeError: ‘function’ object is not subscriptable as shown below:
To solve this TypeError, you need to replace the square brackets with round brackets like this:
study('Python') # Learning Python
The same rule applies when your function has multiple arguments.
You need to pass the arguments inside round brackets and not square brackets as follows:
def add(a, b):
return a + b
result = add[3, 2] # ❗️TypeError
result = add(3, 2) # ✅
Your code should run once you replaced the square brackets with round brackets as shown above.
Case #2 mistaken a list for a function
Another common case where this TypeError happens is when you create a function with the same name as a list.
The code below has a list and a function named study
:
study = ['Python', 'Java']
def study(subject):
print("Learning", subject)
print(study[1]) # ❗️TypeError
When you define a function with the same name after defining a variable, that variable will refer to the function instead of the variable.
Because the study
variable in the code above is a function, Python responds with the same error.
To fix this issue, you need to rename either the list or the function so they don’t collide:
# 👇 Rename the list
study_list = ['Python', 'Java']
def study(subject):
print("Learning", subject)
print(study_list[1]) # Java
The code above renamed the list name to study_list
. You are free to adjust your code as required.
Conclusion
The Python message TypeError: ‘function’ object is not subscriptable happens when you use square brackets []
beside a function name to call that function.
This is not allowed in Python because functions are not subscriptable objects. They do not have elements that you can access with an index like strings, lists, or tuples.
To solve this error, use parentheses ()
when calling a function and passing arguments to that function. Use the code examples in this article to help you. 👍