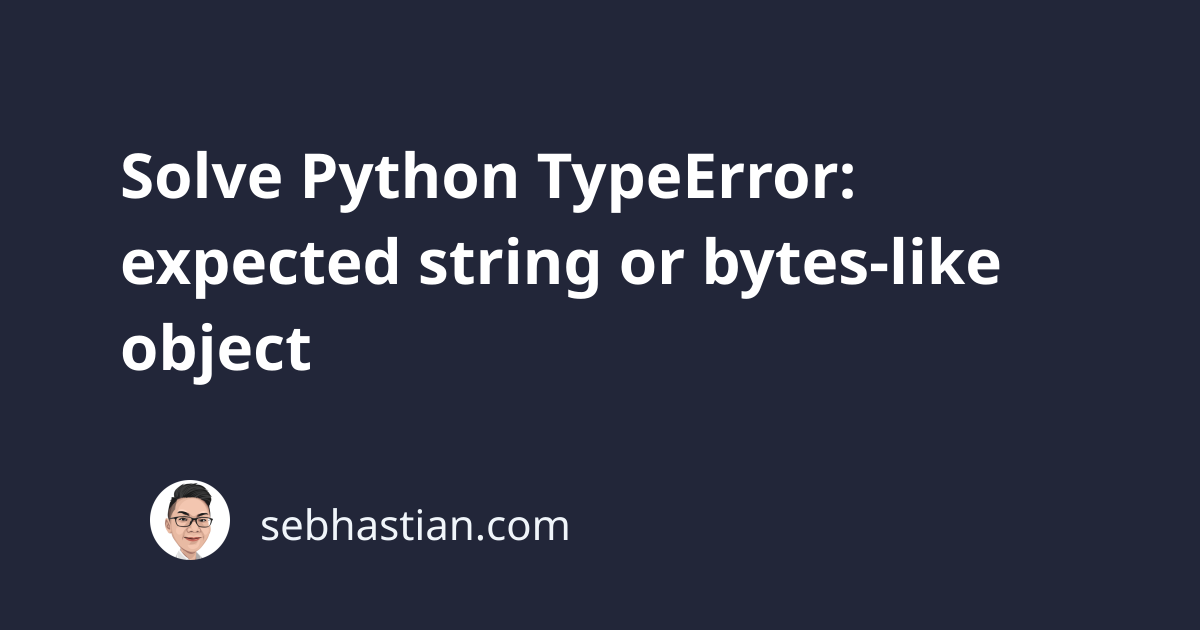
Python TypeError: expected string or bytes-like object commonly occurs when you pass a non-string argument to a function that expects a string.
To solve this error, you need to make sure you are passing a string or bytes-like object as the argument of that function.
Two functions that commonly cause this error are:
re.sub()
re.findall()
Let’s see how to solve the error for both functions in this article.
Solve re.sub() causing TypeError: expected string or bytes-like object
The re.sub()
function in Python is used to replace a pattern
in a string
with a replacement
value.
Here’s the function signature:
re.sub(pattern, replacement, string)
Python expects a string as the third argument of the function.
When you run the following code:
import re
result = re.sub("world", "there", 1234) # ❗️TypeError
Python responds with an error as shown below:
To solve this TypeError, you need to convert the third argument of the function to a string using the str()
function:
result = re.sub("world", "there", str(1234)) # ✅
print(result) # 1234
This solution also works when you pass a list as the third argument of the re.sub()
function.
Suppose you want to replace all number values in a list with an X
as shown below:
import re
list = [0, "A", 2, "C", 3, "Z"]
list_as_string = re.sub("[0-9]+", "X", list) # ❗️
print(str)
The call to sub()
function will cause an error because you are passing a list.
To solve this error, you need to convert the list to a string with the str()
function.
From there, you can convert the string back to a list with the strip()
and split()
functions if you need it.
Consider the example below:
import re
list = [0, "A", 2, "C", 3, "Z"]
# 👇 Convert list to string with str()
list_as_string = re.sub("[0-9]+", "X", str(list))
# The following code converts the string back to list
# 👇 Remove quotation mark from the string
list_as_string = re.sub("'", "", list_as_string)
# 👇 use strip() and split() to create a new list
new_list = list_as_string.strip('][').split(', ')
print(new_list)
# ['X', 'A', 'X', 'C', 'X', 'Z']
And that’s how you solve the error when using the re.sub()
function.
Solve re.findall() causing TypeError: expected string or bytes-like object
Python also raises this error when you call the re.findall()
function with a non-string value as its second argument.
To solve this error, you need to convert the second argument of the function to a string as shown below:
import re
list = [0, "A", 2, "C", 3, "Z"]
# Convert list to string with str()
matches = re.findall("[0-9]+", str(list))
print(matches)
# ['0', '2', '3']
The re.findall()
function already returns a list, so you can print the result as a list directly.
Conclusion
To conclude, the Python TypeError: expected string or bytes-like object error occurs when you try to pass an object of non-string type as an argument to a function that expects a string or bytes-like object.
To solve this error, you need to make sure that you are passing the correct type of object as an argument to the function.
Two functions that commonly cause this error are the re.sub()
and re.findall()
functions from the re
module.
In some cases, you may need to convert the object to a string using the str()
function when passing it as an argument.