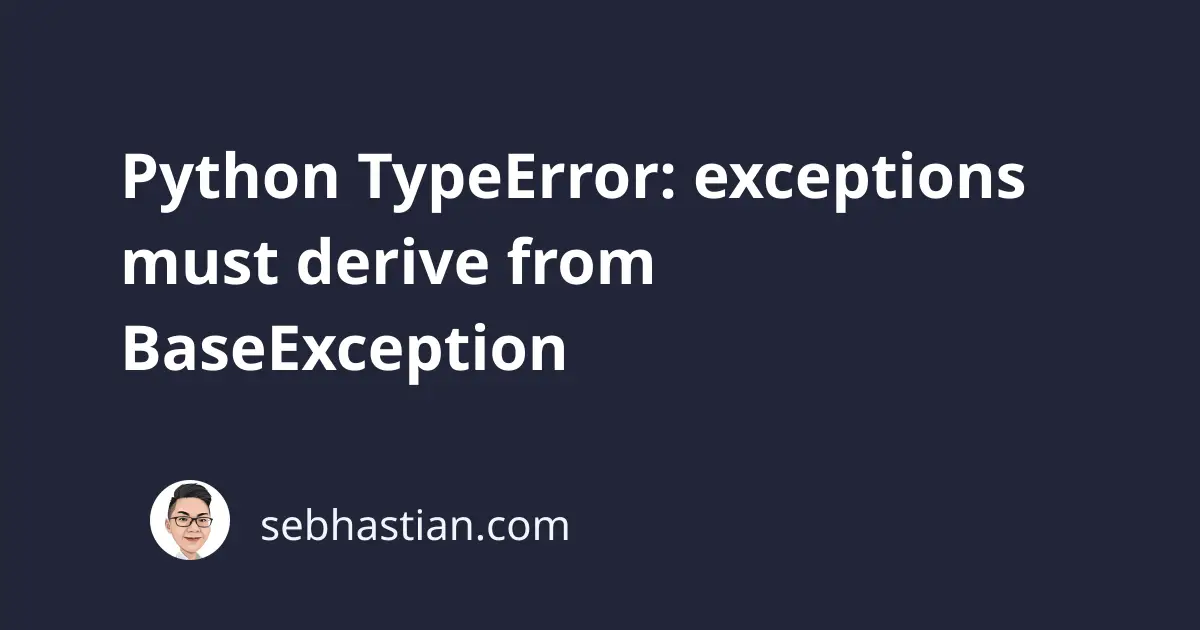
Python TypeError: exceptions must derive from BaseException
is a common error that may occur when you work with exceptions.
This tutorial helps you understand why this error happens and how to resolve it.
Why TypeError: exceptions must derive from BaseException occurs
The TypeError: exceptions must derive from BaseException
occurs when you try to raise an error without passing a class that inherits from the BaseException
class.
For example, suppose you want to raise an error when the x
variable is not a string like this:
x = 9
if type(x) is not str:
raise "Error! x must be a string" # ❌
Because the raise
keyword above is not followed with a class that inherits from the BaseException
class, Python will show the following error message:
Traceback (most recent call last):
File ...
raise "Error! x must be a string"
TypeError: exceptions must derive from BaseException
To fix this error, you need to pass a built-in exception or your custom exception class.
How to fix TypeError: exceptions must derive from BaseException
Depending on the nature of your error message, you can choose from a wide selection of exception classes provided by Python.
When you have a wrong type as in the above example, you would use the TypeError
class like this:
x = 9
if type(x) is not str:
raise TypeError("Error! x must be a string") # ✅
Other common exception classes are AssertionError
, ValueError
, or RuntimeError
.
You can use any exception class as shown in this Exception hierarchy.
You can even define your own custom exception class in Python. To do so, you need to create a class that extends from the Exception
class.
Suppose you want to create an exception class named MyException
. Here’s how you do it:
class MyException(Exception):
def __init__(self, message):
self.message = message
def __str__(self):
return self.message
Once defined, you can use the class when raising an error as follows:
class MyException(Exception):
def __init__(self, message):
self.message = message
def __str__(self):
return self.message
x = 9
if type(x) is not str:
raise MyException("Error! x must be a string") # ✅
But creating your own exception classes will increase the complexity of your code.
I’d recommend you only create a customized exception class when the built-in ones don’t cover the error you need to raise.
Should I use Exception or BaseException?
Python developers are recommended to create a custom exception class using the Exception
class instead of BaseException
.
This is because the BaseException
class include errors that must not be caught by the try ... except Exception
block, such as SystemExit
.
When you create your own custom exceptions, you want those exceptions to be caught and show a traceback to help you identify the error.
This is the reason why you’re recommended to use the Exception
class.
Conclusion
To conclude, the TypeError: exceptions must derive from BaseException
occurs when you try to raise
an error without defining the exception class.
To fix this error, you need to pass a built-in or custom exception class that’s relevant to the error message you want to raise.
Good work resolving this error! See you in other tutorials 👍