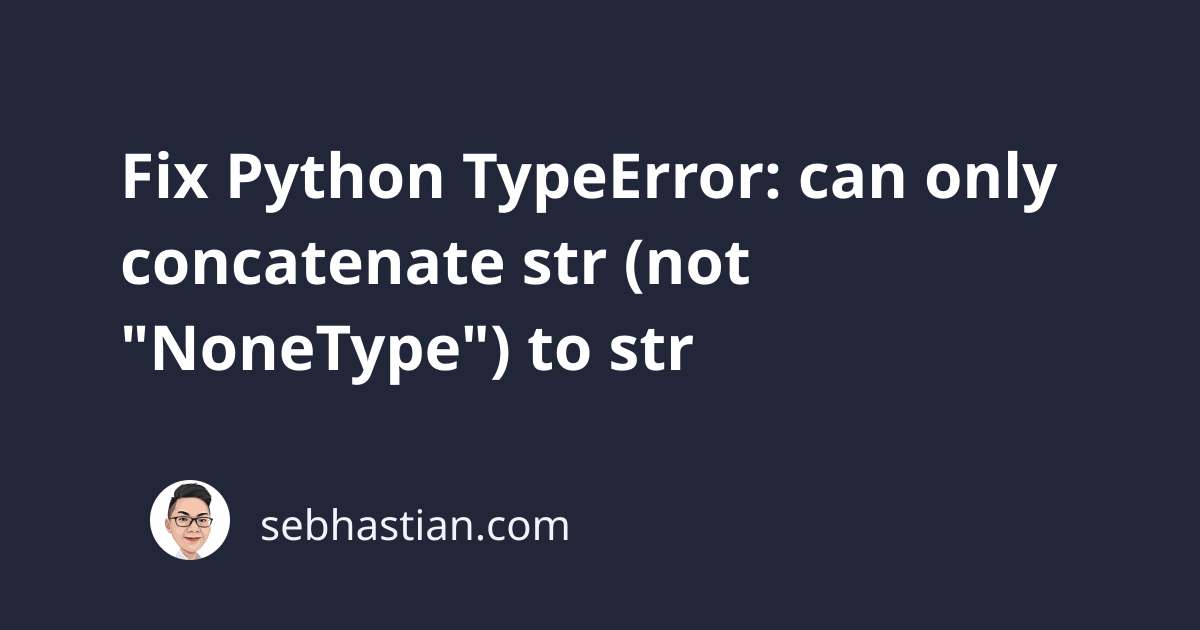
Python shows TypeError: can only concatenate str (not "NoneType") to str
when you try to concatenate a string with a None
value with the +
operator.
To fix this error, you need to avoid concatenating a None
value with a string.
Let’s see an example. Suppose you have the Python code below:
x = None
# ❌ TypeError: can only concatenate str (not "NoneType") to str
print("The value of x is " + x)
Because the x
variable is None
, concatenating it with a string in the print()
function produces the error:
Traceback (most recent call last):
File ...
print("The value of x is " + x)
TypeError: can only concatenate str (not "NoneType") to str
To avoid concatenating a None
with a string, you can first check the value of your variable using an if
statement.
When the variable is not None
, then concatenate the variable to a string as shown below:
x = None
if x is not None:
print("The value of x is " + x)
else:
print("The value of x is None")
Alternatively, you can also provide a default value for the variable when the value is None
as follows:
x = None
if x is None:
x = "Y"
print("The value of x is " + x) # ✅
By adding a default value to replace None
, you ensure that the TypeError: can only concatenate str (not "NoneType") to str
is avoided.
The None
value is a special value in Python that represents the absence of a value or a null value. It is often used to indicate that a variable or a function has no return value.
To avoid the TypeError
, you need to be aware of the circumstances under which the None
value is produced by Python and handle it appropriately in your code.
Several common sources of None
value when running Python code are:
- Assigned
None
to a variable explicitly - Calling a function that returns nothing
- Calling a function that returns only under a certain condition
You’ve seen an example of the first case, so let’s look at the second and third cases.
When you call a function that has no return
statement, the function will return a None
value implicitly.
Consider the code below:
def greet():
pass
result = greet()
print(result) # None
When a function doesn’t have a return
statement defined, it returns None
.
This also applies to Python built-in functions, such as the sort()
method of the list
object.
listings = [5, 3, 1]
# call sort() on list
output = listings.sort()
print(output) # None
print(1 in listings) # True ✅
The sort()
method sorts the original listings
variable without returning anything. When you assign the result to a variable, that variable contains None
.
Finally, you can also get None
when you have a function that has a conditional return
statement.
Consider the greet()
function below:
def greet(name):
if name:
return f"Hello {name}!"
# Call greet with empty string
output = greet("")
print(output) # None
output = greet("Nathan")
print("n" in output) # True ✅
The greet
function only returns a string value when the name
variable evaluates to True
.
Since an empty string evaluates to False
, the first call to the greet
function returns None
.
To avoid having None
returned by your function, you need to add another return
statement as follows:
def greet(name):
if name:
return f"Hello {name}!"
return "Hello Unknown!"
In the code above, the second return
statement will be executed when the name
variable evaluates to False
.
Now you’ve learned several common cases that may cause a None
value to enter your code.
Conclusion
The TypeError: can only concatenate str (not "NoneType") to str
error in Python occurs when you concatenate a None
value with a string value.
To fix this error, you need to avoid concatenating a None
with a string.
You can do this by using an if .. is not None
statement to check if the variable is None
before concatenating it.
You can also use the if .. is None
expression and provide a default value to use when the variable contains None
.