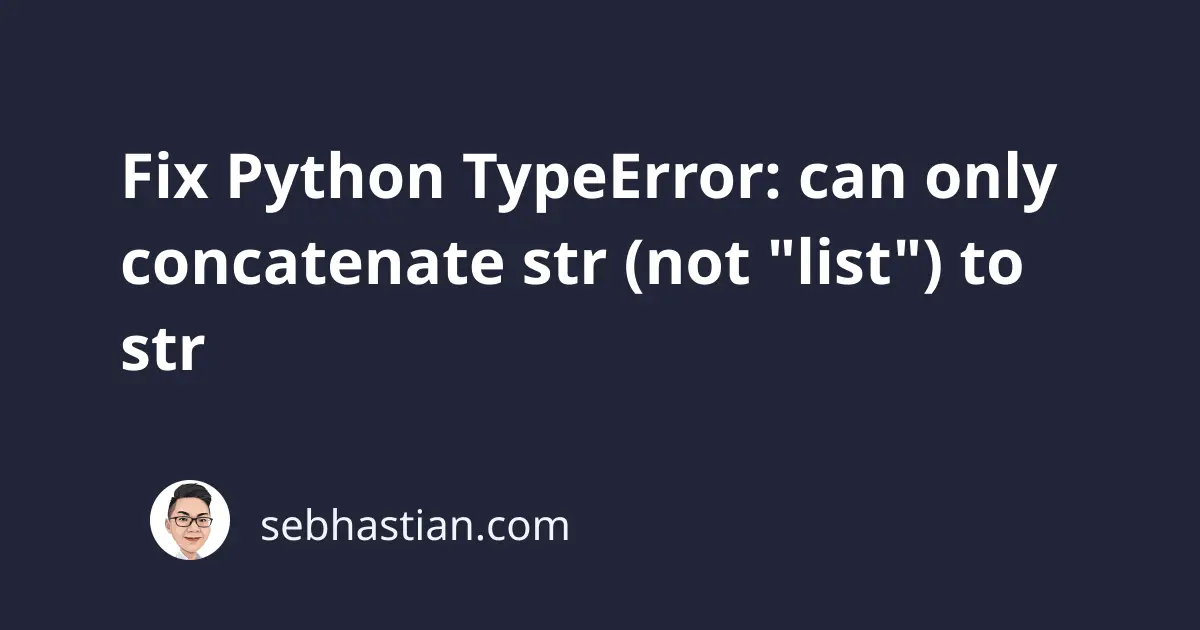
Python shows TypeError: can only concatenate str (not "list") to str
when you try to concatenate a string and a list using the +
operator.
To solve this error, you need to convert the list into a string so that the concatenation can happen.
Python +
operator only allows you to concatenate data of the same type (i.e. a string with a string, a list with a list)
The example below tries to concatenate a string and a list in the print()
function:
items = [1, 2, 3]
# ❌ TypeError: can only concatenate str (not "list") to str
print("The items are " + items)
Because Python doesn’t allow concatenating different types of values, it responds with the following error:
Traceback (most recent call last):
File ...
print("The items are " + items)
TypeError: can only concatenate str (not "list") to str
To fix this error, you need to convert the list to a string before concatenating it.
You can do this using Python’s built-in str()
function, as shown in the example below:
items = [1, 2, 3]
# convert list to string before concatenating
print("The items are " + str(items))
Alternatively, you can pass the items
list as the second argument to the print()
function as follows:
items = [1, 2, 3]
print("The items are", items) # ✅
The print()
function automatically converts the items
list to a string when you pass it as an extra argument.
You can also use the string format()
method or f-strings to insert the values of the list items into the string.
Consider the examples below:
items = [1, 2, 3]
# use string formatting to insert list items into string
print("The items are {}".format(", ".join(str(i) for i in items)))
# use f-strings to insert list items into string
print(f"The items are {', '.join(str(i) for i in items)}")
In the examples above, the str()
function is used to convert each list item to a string, and the join()
method is used to join the list items into a single string separated by a comma.
The resulting string is then inserted into the main string using string formatting or f-strings.
Keep in mind that the +
operator can only be used to concatenate two strings in Python.
If you want to combine other types of objects, such as lists, you need to convert them to strings first.
Here are other errors related to concatenating a string with other types:
I hope this article helps you write better Python code. 🙏