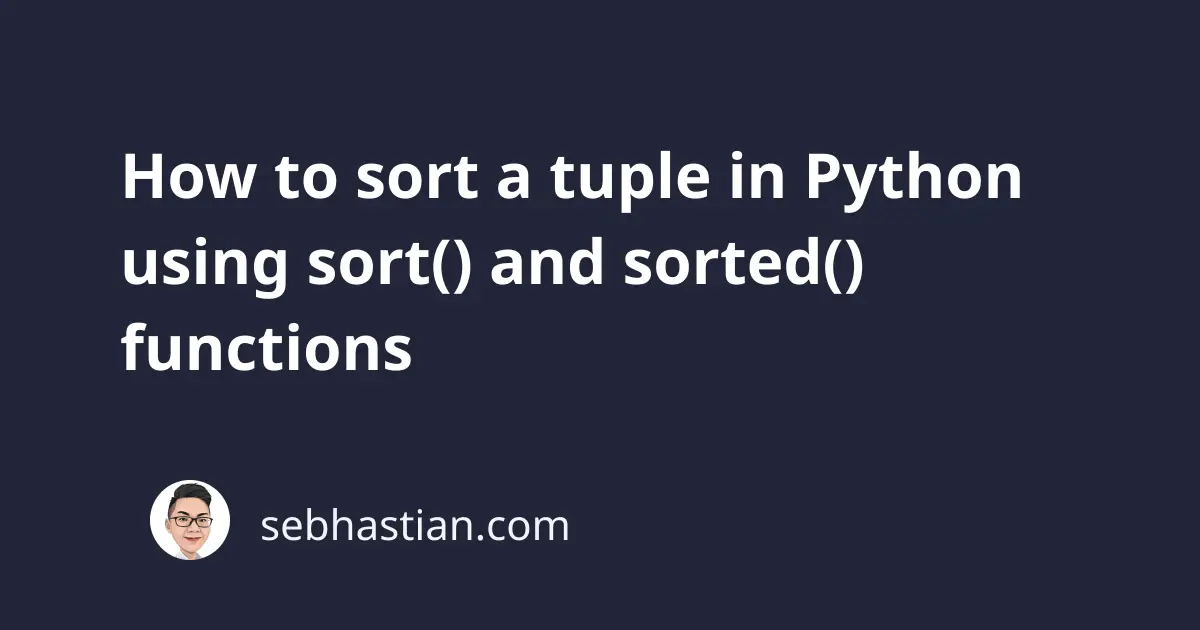
When you need to sort a tuple in Python, there are two methods that you can use:
- Using the
sorted()
function - Using the
list.sort()
method
This tutorial will explain how to use both solutions in practice.
Using the sorted()
function
The Python sorted()
function is used to sort an iterable object based on the values it contained.
This function can be used to sort a tuple as follows:
my_tuple = (5, 8, 1, 0, 6)
new = sorted(my_tuple)
print(new)
Output:
[0, 1, 5, 6, 8]
If you want to sort a tuple in descending order, you can specify a reverse
argument as follows:
my_tuple = (5, 8, 1, 0, 6)
new = sorted(my_tuple, reverse=True)
print(new)
Output:
[8, 6, 5, 1, 0]
This function can also be used to sort a list of many tuples as shown below:
list_of_tuples = [(2, 5, 1), (4, 3, 1), (2, 0, 7)]
new = sorted(list_of_tuples)
print(new)
Output:
[(2, 0, 7), (2, 5, 1), (4, 3, 1)]
As you can see, the tuples are sorted by comparing the elements in the same position sequentially.
This means that Python will compare the elements in position one first, and when the elements are equal, it will compare the elements at the second position, and so forth.
When you have multiple tuples, the elements inside each tuple won’t be sorted. Only the tuples are sorted.
Using the list.sort()
method
As an alternative, you can also use the list.sort()
method to sort a tuple as follows:
my_tuple = (5, 8, 1, 0, 6)
my_list = list(my_tuple)
my_list.sort()
new_tuple = tuple(my_list)
print(new_tuple)
When using the list.sort()
method, you need to convert the tuple into a list, call the sort()
method on the list, and convert the list back to a tuple.
If you try to chain the call to the sort()
method, the result would be None
because sort()
doesn’t return any value.
The following code:
my_tuple = (5, 8, 1, 0, 6)
new_tuple = tuple(list(my_tuple).sort())
print(new_tuple)
Will return a TypeError: 'NoneType' object is not iterable
.
Because this method requires more steps, it’s better to use the sorted()
function.
Now you’ve learned how to sort a tuple in Python. Nice work!