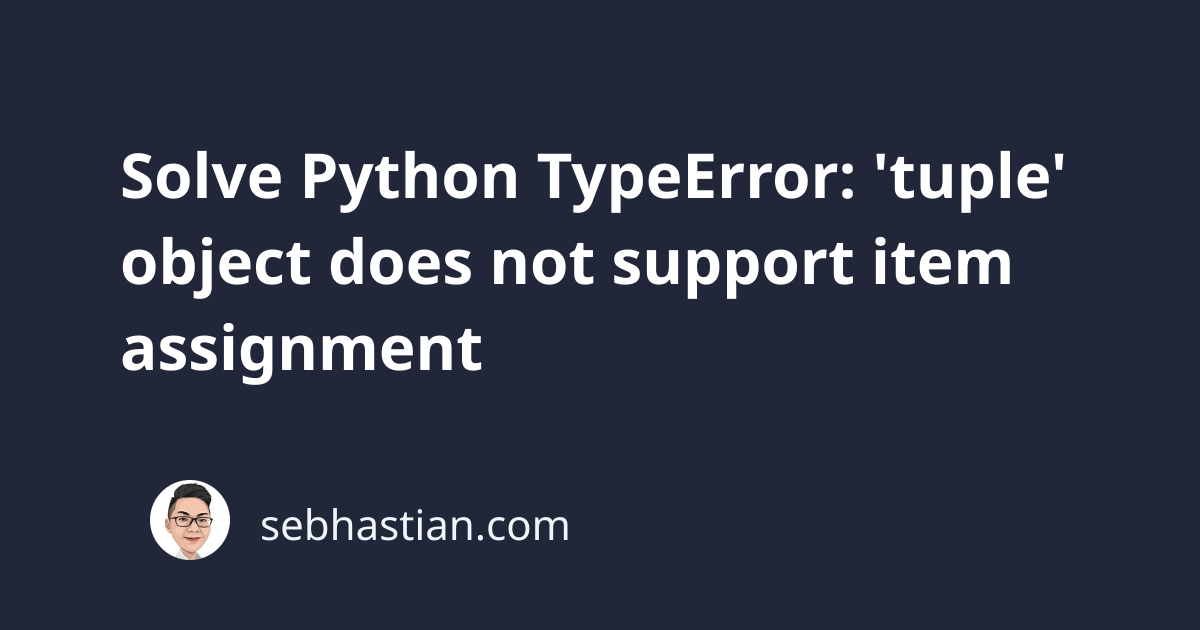
In Python, tuples are immutable sequences that cannot be modified once they are created. This means that you cannot change, add, or delete elements from a tuple.
When you try to modify a tuple using the square brackets and the assignment operator, you will get the “TypeError: ’tuple’ object does not support item assignment” error.
Consider the example below:
fruits = ("Orange", "Apple", "Banana")
fruits[0] = "Mango"
The above code tries to change the first element of the tuple from “Orange” to “Mango”.
But since a tuple is immutable, Python will respond with the following error:
python3 /script.py
Traceback (most recent call last):
File "/script.py", line 3, in <module>
fruits[0] = "Mango"
~~~~~~^^^
TypeError: 'tuple' object does not support item assignment
There are two solutions you can use to edit a tuple in Python.
Solution #1: Change the tuple to list first
When you need to modify the elements of a tuple, you can convert the tuple to a list first using the list()
function.
Lists are mutable sequences that allow you to change, add, and delete elements.
Once you have made the changes to the list, you can convert it back to a tuple using the tuple()
function:
fruits = ("Orange", "Apple", "Banana")
# 1. Convert tuple to list
fruits_list = list(fruits)
# 2.Modify the list
fruits_list[0] = "Mango"
# 3. Convert list back to tuple
fruits = tuple(fruits_list)
print(fruits) # ("Mango", "Apple", "Banana")
By converting a tuple into a list, you can modify its elements. Once done, convert it back to a tuple.
Solution #2: Create a new tuple
When you only need to modify a single element of a tuple, you can create a new tuple with the modified element.
To access a range of elements from a tuple, you can use the slice operator.
For example, the following code creates a new tuple by adding a slice of elements from an existing tuple:
fruits = ("Orange", "Apple", "Banana")
# Create a new tuple with the modified element
fruits = ("Mango",) + fruits[1:]
print(fruits) # ("Mango", "Apple", "Banana")
The code fruits[1:]
means you are slicing the fruits
tuple to return the second element to the last.
Creating a new tuple is more efficient than converting the entire tuple to a list and back as it requires only one line of code.
But this solution doesn’t work when you need a complex modification.
Conclusion
The Python TypeError: tuple object does not support item assignment issue occurs when you try to modify a tuple using the square brackets (i.e., []
) and the assignment operator (i.e., =
).
A tuple is immutable, so you need a creative way to change, add, or remove its elements.
This tutorial shows you two easy solutions on how to change the tuple object element(s) and avoid the TypeError.
Thanks for reading. I hope this helps! 🙏