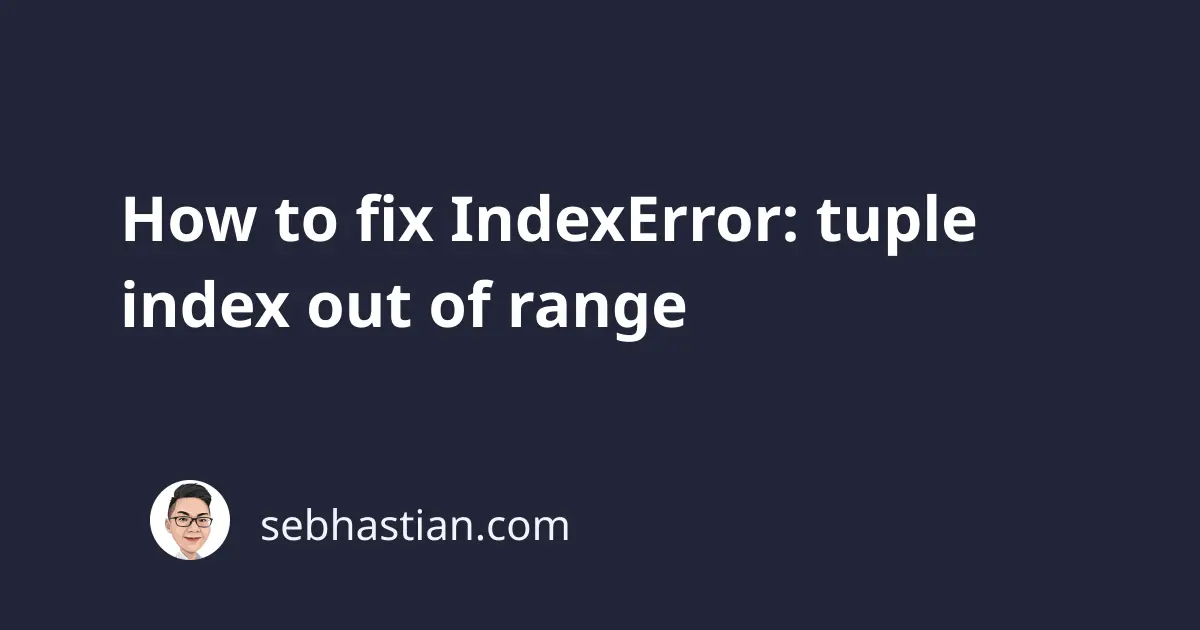
When you’re working with tuples in Python, you might see the following error:
IndexError: tuple index out of range
This error usually occurs when you try to access an index that doesn’t exist. It could be that you count the index wrong as a tuple index starts from zero.
Let’s see an example that causes this error and how to fix it.
How to reproduce this error
Suppose you have a tuple that contains the names of cities as follows:
cities = ("London", "Kuala Lumpur", "Wellington")
Now, suppose you want to print the name Wellington
to the terminal with print()
, so you access the tuple index as follows:
print(cities[3])
But instead of printing the city name, Python responds with this error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(cities[3])
IndexError: tuple index out of range
You receive an error because index 3
doesn’t exist in the cities
tuple.
A tuple index starts from 0
, so the available index in cities
are:
0
forLondon
1
forKuala Lumpur
2
forWellington
When you specify any other number as the index you want to access, you’ll get the tuple index out of range error.
How to fix this error
To resolve this error, make sure you’re accessing an index that exists in your tuple.
The correct index number to access Wellington
is 2
, so this is the correct code:
print(cities[2]) # Wellington
If you use a for
loop and range
to iterate over a tuple, make sure that the range numbers you specified are inside the range of available index numbers.
Failing to do so will trigger this error as well. Suppose you try to access the second and third items of the cities
tuple like this:
cities = ("London", "Kuala Lumpur", "Wellington")
for i in range(1, 4):
print(cities[i])
The range
syntax above produce numbers 1
, 2
, and 3
, so it’s the equivalent of this code:
print(cities[1])
print(cities[2])
print(cities[3])
Because cities
index only goes up to 2
, Python shows this error with the last print
statement:
Kuala Lumpur
Wellington
Traceback (most recent call last):
File "main.py", line 4, in <module>
print(cities[i])
IndexError: tuple index out of range
To avoid this error, make sure you’re specifying a range
that’s within the tuple index. Revise the code as follows:
cities = ("London", "Kuala Lumpur", "Wellington")
for i in range(1, 3):
print(cities[i])
Now you won’t see the error in the output:
Kuala Lumpur
Wellington
The last two items are successfully printed. The code is free from error.
Conclusion
The IndexError: tuple index out of range
occurs when you access a tuple using an index number that’s outside of the available range. To fix this error, make sure your tuple has an item at the index number you specified.
I hope this tutorial helps. See you again in other articles! 👍