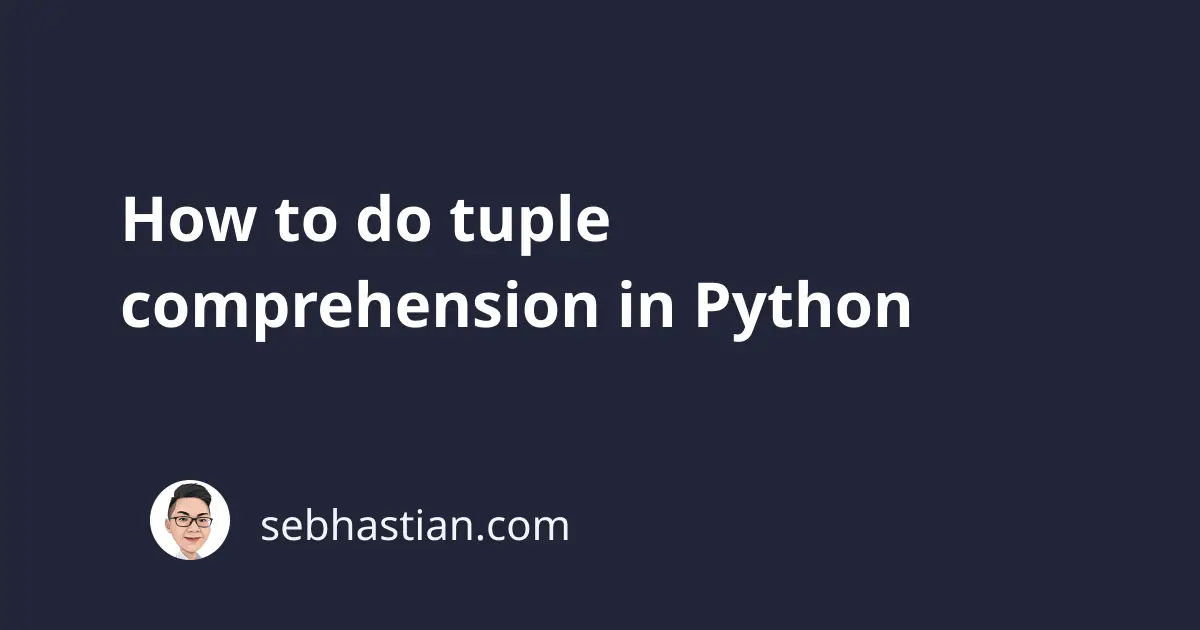
To do a tuple comprehension in Python, you can use the generator function to create a generator object and transform it into a tuple using the tuple()
function.
Here’s an example of performing a tuple comprehension:
my_tuple = tuple(x for x in range(4))
print(my_tuple)
Output:
(0, 1, 2, 3)
The list comprehension syntax uses the square brackets []
like the literal used for creating a list, but the round brackets ()
were used to create a generator in Python.
A generator produces a generic iterator object which you can pass to the tuple()
function to convert it to a tuple.
While using tuple()
and generator is not a true tuple comprehension syntax, it’s sufficient for the rare case where you need to produce a tuple using a syntax similar to a list comprehension.
Another way you can create a tuple comprehension is to just use a list comprehension and convert the result into a tuple:
my_tuple = tuple([x for x in range(4)])
print(my_tuple)
The code above converts the result of the list comprehension into a tuple.
In the end, tuples and lists are the same except that items in a tuple can’t be modified. You can create a tuple from a list as well, so there’s no need to define a new syntax for tuple comprehension.
I hope this article helps. Happy coding! 👍