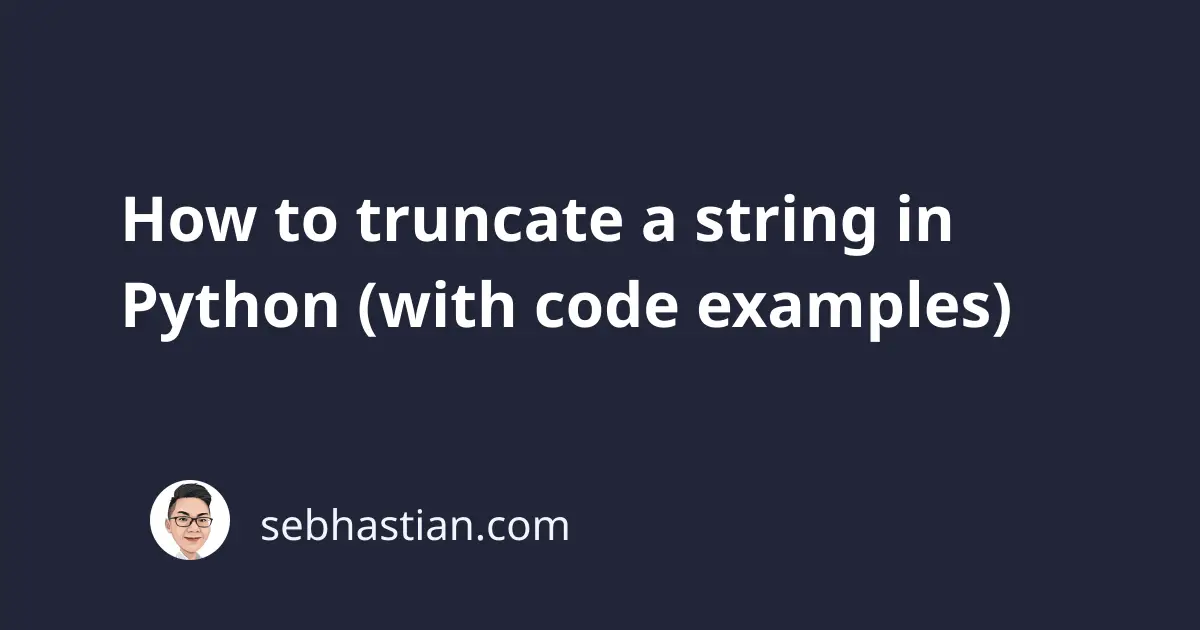
Truncating a string can be useful when you have a limited space to display a long text.
There are several ways you can truncate a string in Python:
This article will show you how to use the methods above to truncate a Python string.
Let’s start with the slicing method.
Use slicing notation
String slicing is a way to select parts of a string by specifying the start
and end
indices of the slice.
The basic syntax of string slicing is str[start:end]
where:
start
is the index of the first character to include in the sliceend
is the index to stop slice.
To truncate a string, you can pass only the end
part of the syntax.
For example, here’s how to get the first 10 characters from a text:
text = "The weather is very nice"
short_text = text[:10]
print(short_text) # The weathe
As you can see, the text[:10]
syntax cuts the first 10 characters of “The weather is very nice” text and return it.
When truncating a string, you might want to add an ellipsis (…) to the end of the short text.
You can do so by using the concatenation operator as follows:
text = "The weather is very nice"
short_text = text[:10] + "..."
print(short_text) # The weathe...
You can also use the ternary operator to truncate a string only when its length is greater than the allowed size:
text = "The weather is very nice"
# Cut the text only when greater than 10
summary = text[:10] + '...' if len(text) > 10 else text
print(summary) # The weathe...
And that’s how you truncate a string with string slicing.
Next, let’s see how to use string formatting syntax to truncate a string.
Use string formatting
The string formatting syntax can be used to truncate a string because you can add the max length of characters in the replacement field.
For example, to truncate the same string text to 10 characters, you can use the following code:
text = "The weather is very nice"
short_text = "{:.10}".format(text)
print(short_text) # The weathe
The replacement field {:.10}
in the code above means that the maximum length of the field is 10 characters.
As a result, the text
value was truncated to fit the rule.
Just like with string slicing, you can use the ternary operator to truncate the string only when the length is longer than allowed:
text = "The weather is very nice"
# Cut the text only when greater than 10
summary = "{:.10}".format(text) + '...' if len(text) > 10 else text
print(summary) # The weathe...
Finally, you can use the textwrap.shorten()
method to truncate a string. Let’s learn about it next.
Using the textwrap shorten() method
The Python textwrap
module has a method named shorten()
that you can use to truncate a string.
The shorten()
method takes three parameters:
text
: the string to be truncatedwidth
: the maximum width of the truncated stringplaceholder
: a string to be appended at the end of the truncated string (default is “[…]”)
Here’s an example of how to use the shorten()
method:
import textwrap
text = "The weather is very nice"
summary = textwrap.shorten(text, width=10, placeholder="...")
print(summary) # The...
The shorten()
method takes into account the placeholder
length to the specified width
argument.
This means that when your width
is ten characters long, the method will truncate the text to make space for the ellipsis symbol.
Finally, the method also removes the entire word instead of the characters so that the result is still readable.
As a result, the truncated text can be shorter than the specified width
.
For more complex cases, you can visit the textwrap.shorten documentation
Conclusion
You’ve just learned three different ways to truncate a string in Python.
String slicing and formatting are useful when you have a simple text. When you have a complex case, you can use the textwrap.shorten()
method.
You can use the method you liked best. 👍