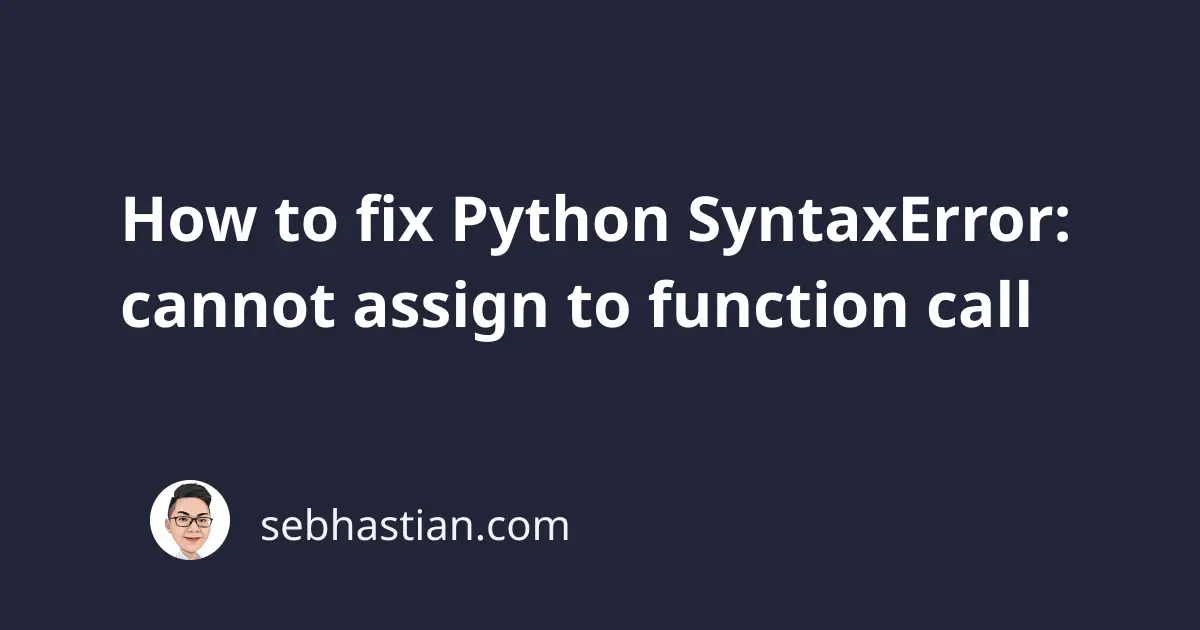
Python shows SyntaxError: cannot assign to function call
error when you assign a value to the result of a function call.
Here’s an example code that triggers this error:
def add(a, b):
return a + b
result = add(3, 4)
add(3, 4) = 5 # ❌ SyntaxError
In the code above, the number 5
is assigned to the result of calling the add()
function.
Python responds with the error below:
add(3, 4) = 5
^^^^^^^^^
SyntaxError: cannot assign to function call here.
Maybe you meant '==' instead of '='?
In Python, a function call is an expression that returns a value. It cannot be used as the target of an assignment.
To fix this error, you need to avoid assigning a value to a function call result.
When you want to declare a variable, you need to specify the variable name on the left side of the operator and the value on the right side of the operator:
# Assign value 5 to the variable x
x = 5
You can also assign the function call result to a variable as follows:
x = add(3, 4)
print(x) # 7
If you want to compare the result of a function call to a value, you need to use the equality comparison operator ==
.
The following code example is valid because it checks whether the result of add()
function equals to 5
:
# Checks whether add(3, 4) equals to 5
x = 5
add(3, 4) == x # ✅
A single equal operator =
assigns the right side of the operator to the left side, while double equals ==
compares if the left side is equal to the right side.
You can even assign the result of the comparison to a variable like this:
result = add(3, 4) == x # ✅
print(result) # False
Finally, you can also encounter this error when working with Python iterable objects (like a list, dict, or tuple)
You can’t reassign or compare a list element as long as you used the parentheses as shown below:
numbers = [1, 2, 3]
numbers(2) = 5 # ❌ SyntaxError
numbers(2) == 5 # ❌ TypeError
When you use parentheses with the equality comparison operator, Python responds with TypeError: 'list' object is not callable
.
Whether you want to reassign or compare a list element, you need to use the square brackets like this:
numbers = [1, 2, 3]
numbers[2] = 5 # ✅
print(numbers) # [1, 2, 5]
print(numbers[2] == 5) # True
And that’s how you fix Python SyntaxError: cannot assign to function call
error.