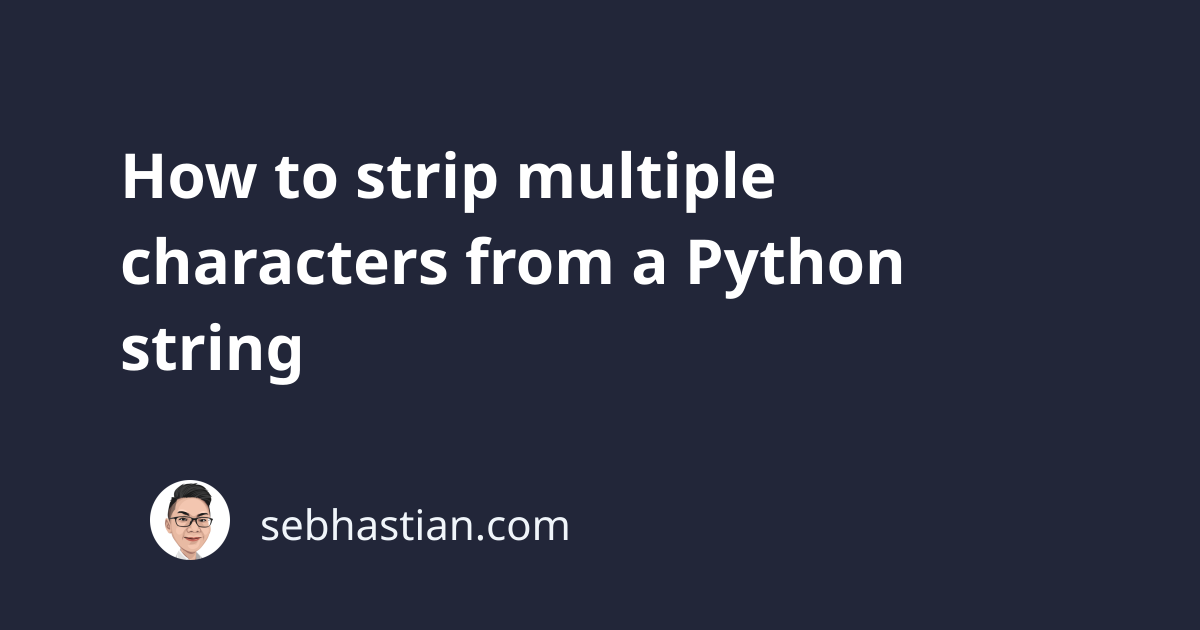
When you need to strip multiple characters from a string in Python, you can use either the str.strip()
or re.sub()
method.
The strip()
function is used to remove leading and trailing characters from a string. It returns a new string so the original string is preserved.
Consider the example below:
url = "/-(posts|)(index|)(pages)-/"
new_url = url.strip("/-()")
print(new_url)
Output:
posts|)(index|)(pages
As you can see, the strip()
method from the str
object remove any leading and trailing characters that match the symbols you passed into the method.
If you need to remove the characters in the middle of the string as well, you need to use the sub()
method from the regular expression (re
) module like this:
import re
url = "/-(posts|)(index|)(pages)-/"
new_url = re.sub("[/\-()]", "", url)
print(new_url)
Output:
posts|index|pages
You need to specify a set of characters using the square brackets []
and escape the -
symbol with the backslash \
.
The regular expression sub()
method will replace the pattern we specified as the first argument of the method.
Here’s another example:
import re
my_str = "{name: John},(age: 28)"
new_str = re.sub("[{}()]", "", my_str)
print(new_str)
Output:
name: John,age: 28
Because we specified an empty string as the second argument, any matching pattern is removed from the string.
And that’s how you strip multiple characters from a Python string. I hope this tutorial is helpful. Happy coding! 🙌