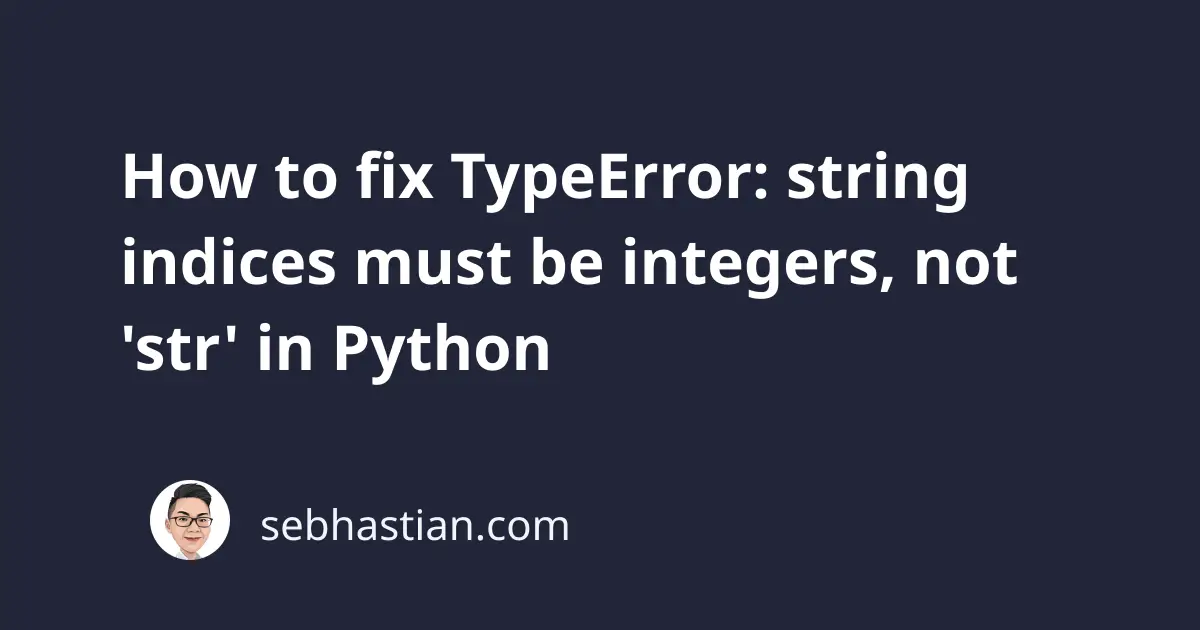
When programming with Python, you might encounter the following error:
TypeError: string indices must be integers, not 'str'
This error occurs when you attempt to access a character in a string using a string.
In this tutorial, I will show you an example that causes this error and how to fix it in practice.
How to reproduce this error
In Python, items of iterable objects such as lists, tuples, or strings can be accessed by using their index numbers.
The index number starts from 0 and increases by 1 for each subsequent item in the object.
For example, here’s how to access the first character in a string:
# Declare a string variable
my_str = "Good Morning!"
# Print the first character in the string
print(my_str[0]) # G
The code above will print G
because it’s the first character of the string my_str
.
The error occurs when you try to access the first character using a string as follows:
my_str = "Good Morning!"
print(my_str['a']) # ❌
Output:
Traceback (most recent call last):
File ...
print(my_str['a'])
TypeError: string indices must be integers, not 'str'
To fix this error, make sure you’re passing an integer inside the square brackets.
How to fix this error
The following three scenarios may cause this error:
- Slicing a string with a wrong syntax
- Accessing a dictionary in a wrong way
- Accessing a JSON string like a JSON object
The following examples show how to resolve this error in each scenario.
1. Slicing a string with a wrong syntax
Aside from passing the index number of a character, you can also extract several characters from a string by using the slicing syntax.
The slicing syntax is as follows:
string_object[start_index:end_index]
The start_index
is the starting position of the slice and end_index
is the ending position of the slice. The two are separated using a colon (:
) operator.
When start_index
is not specified, it is assumed to be 0. If end_index
is not specified, it is assumed to be the length of the string itself.
The start_index
is included in the output, but the end_index
is excluded.
Here are some examples of slicing a string in Python:
my_str = "Good Morning!"
# return 'Morning'
print(my_str[5:12])
# return 'Good'
print(my_str[:4])
# return 'ood'
print(my_str[1:4])
# return 'ning!'
print(my_str[8:])
But when you pass a wrong slice syntax, then Python will give you a TypeError
:
my_str = "Good Morning!"
# ❌ Use a comma to separate the integers
print(my_str[5,12])
# ❌ Use a dot to separate the integers
print(my_str[5.12])
# ❌ Use a colon but enclosed in quotes
print(my_str['5:12'])
To avoid the error, make sure that the slice syntax has the correct format.
2. Accessing a dictionary in a wrong way
Suppose you have a dictionary object as shown below:
data = {
"name": "Nathan",
"age" : 29
}
Now let’s say you want to access the values and print them out using a for
loop:
for item in data:
print(item['name']) # ❌
The code above will cause a TypeError
because the for
loop will return the dictionary key in each iteration.
Here’s the right way for accessing a dictionary with a for
loop:
data = {
"name": "Nathan",
"age" : 29,
"location": "Japan"
}
for item in data:
print(item, data[item])
Output:
name Nathan
age 29
location Japan
Because the for
loop returns the keys, you can use it to access the dictionary values with the square brackets notation.
3. Accessing a JSON string like a JSON object
In Python, a JSON object is similar to a dictionary object, so you can access its values using the keys.
But at times, you might mistake a string for a JSON object:
json_str = '{"name": "Nathan","age" : 29}'
print(json_str['name']) # ❌
The json_str
object is an encoded JSON string, so trying to access the name
value as shown above will cause a TypeError
.
To fix this, you need to import the json
library and use the json.loads()
method:
import json
json_str = '{"name": "Nathan","age" : 29}'
json_obj = json.loads(json_str)
print(json_obj['name']) # Nathan
print(json_obj['age']) # 29
The loads()
method converts a JSON string into a JSON object, which enables you to access the values like a dictionary.
Keep in mind that you don’t need to call the json.dumps()
method.
The dumps()
method is used to convert an object to a JSON string. But you already have a string here, so there’s no need to call it 🤷🏻
Conclusion
And there you go! Now you know three common scenarios where you may cause the TypeError: string indices must be integers, not 'str'
in Python.
This error happens whenever you try to access a string using a string and not index numbers. It can also occur when you’re working with dictionaries and JSON objects.
The steps in this article should help you resolve the error. Happy coding! 🙌