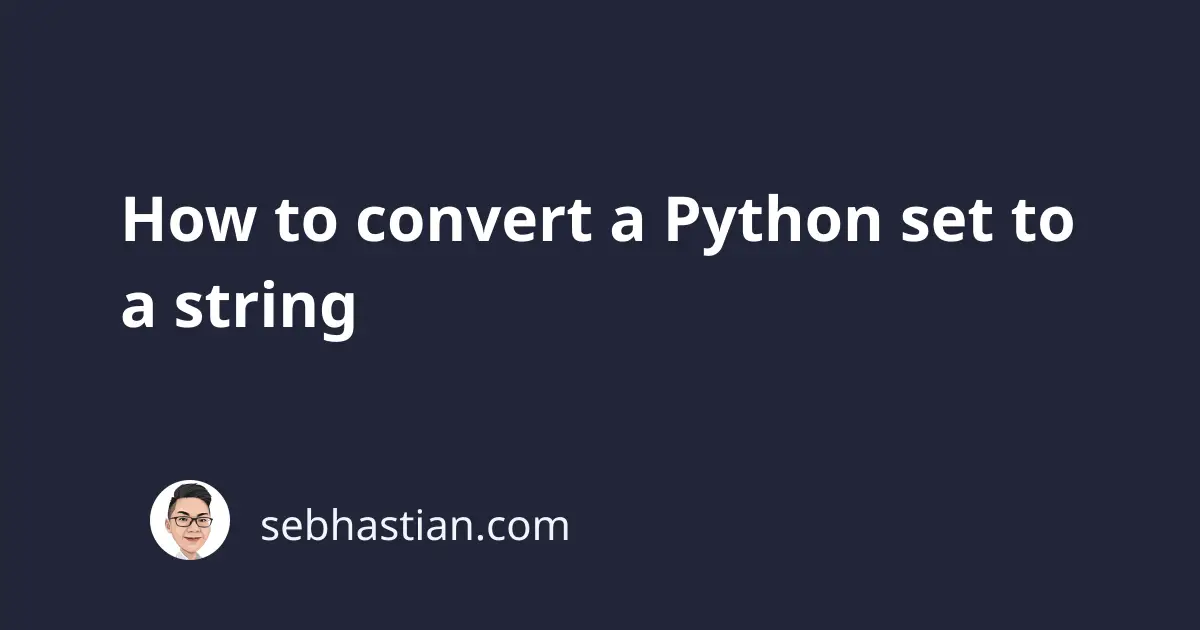
Sometimes, you may need to convert a set object to a string object in Python.
By default, Python allows you to convert a set object to a string using the str()
function as follows:
my_set = {"A", "S", "D"}
my_str = str(my_set)
print(my_str)
But the result will be a string with curly brackets as shown below:
{'S', 'D', 'A'}
Most likely, you want to improve the conversion and remove the curly brackets, the extra quotation mark, and the commas.
There are two methods you can use to better convert a set to a string in Python:
- Using the
str.join()
method - Using a
for
loop
In this tutorial, I will show you how to use both methods in practice.
Convert set to string with the str.join()
method
Since a set is an iterable object, you can use the str.join()
method to join the items in the set as a string.
You can call the join()
method from an empty string as follows:
my_set = {"A", "S", "D"}
my_str = "".join(my_set)
print(my_str)
Output:
ASD
If you want to add some character between the elements, you can specify the character in the string from which you call the join()
method:
my_set = {"A", "S", "D"}
my_str = "-".join(my_set)
print(my_str)
Output:
A-S-D
Keep in mind that a set is an unordered data structure, so the insertion order is not preserved. This is why the string has a different order than the set you defined.
One weakness of this method is that if you have a set of mixed types, then the join()
method will raise an error.
Suppose you have a mixed set as shown below:
my_set = {"A", 1, 2, True, "B"}
my_str = "".join(my_set)
print(my_str)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
my_str = "".join(my_set)
^^^^^^^^^^^^^^^
TypeError: sequence item 0: expected str instance, int found
See how the join()
method expected a str
instance, but int
is found instead.
To overcome this limitation, you need to use a for
loop as explained below.
2. Using a for loop
To convert a set to a string, you can also use a for
loop to iterate over the set and assign each value to a string. Here’s an example:
my_set = {"A", 1, 2.4, "B"}
my_str = ""
for item in my_set:
my_str = my_str + str(item)
print(my_str)
Output:
B2.4A1
One benefit of using this method is that you can convert a set of mixed types to a string by calling the str()
function on each item.
This way, you can convert a set of strings, integers, or floats just fine.
Conclusion
Now you’ve learned how to convert a set to a string in Python. Happy coding! 👍