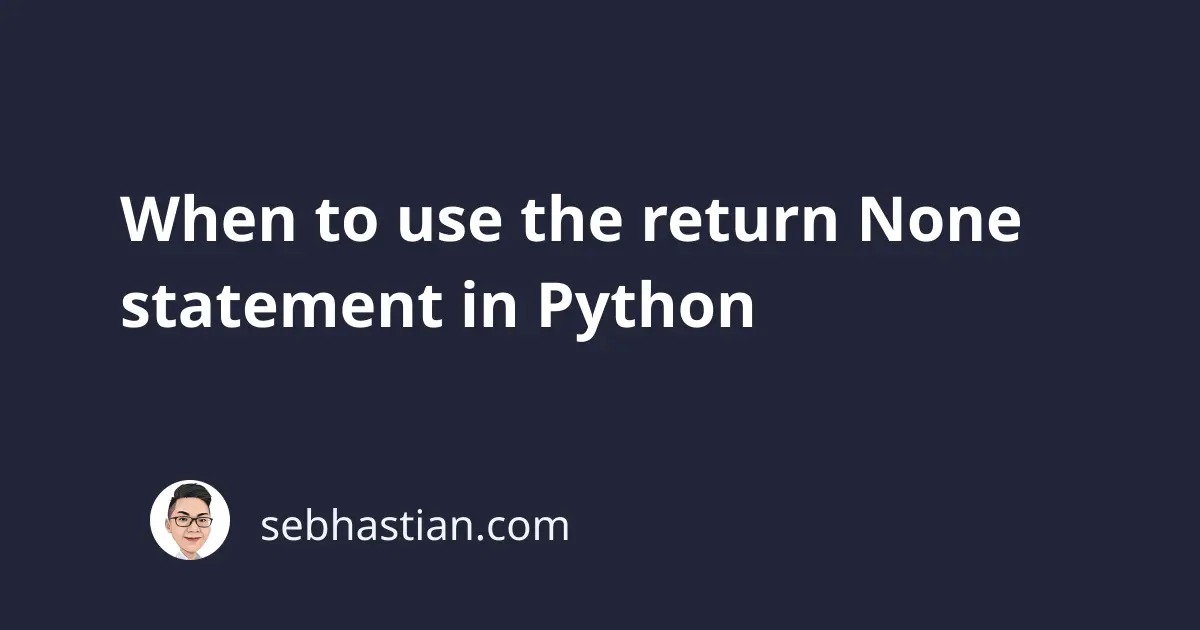
The return
statement in Python is used to end the execution of a function and return a specific value back to the caller.
This return value can then be used for further process by assigning it to a variable. When a function has no return
statement or just the return
keyword without any specific value, then it will return the default value None
.
Consider the following examples:
def a():
return "Hello"
def b():
return
def c():
return None
def d():
x = 9
## Call the functions
print(a()) # Hello
print(b()) # None
print(c()) # None
print(d()) # None
The function a()
in the example returns a specific string value ‘Hello’ back to the caller. Meanwhile, the function b()
just specifies return
without any specific value, so it returns None
.
The c()
function explicitly returns the value None
, and the d()
function doesn’t have a return
statement, so it also returns None
.
Since Python returns None
by default, you might wonder, when do you need to return None
explicitly in your code?
While there’s no difference between implicitly and explicitly returning a None
value, there are times when you might want to provide the return None
statement.
When you put the return None
statement in your code, it’s clear that your function has completed its task without finding any meaningful value to return.
When you didn’t use the return None
statement, the function call result becomes a bit ambiguous. There may be a bug in the function.
For example, suppose you have a function that finds a word from a list as follows:
def find_word(word_list, word):
for w in word_list:
if w == word:
return w
return None
coding_term = ["bug", "system", "shell"]
result = find_word(coding_term, "error")
print(result) # None
Even if we don’t write the return None
statement in the find_word()
function, it will still return None
when there’s no matching word in the list.
But defining the return None
statement means we expect the function to do so. We explicitly tell anyone who reads the code that the function can and will return None
if a certain condition is met.
Because a None
is expected, you can also include code that handles this value in your program as follows:
result = find_word(coding_term, "error")
if result is None:
print("The word is not found in the list!")
And that’s about it. You should explicitly write the return None
statement only when there’s a possibility that your function might return nothing.
One example where the return None
statement is not required is when a function is not expected to return anything, like the greet()
function below:
def greet():
print("Hello!")
result = greet()
print(result) # None
The greet()
function only prints a greeting to the terminal, so there’s no need to specify any return
statement at all.
I hope this tutorial has helped you to understand when you need to use the return None
statement explicitly. Happy coding! 👍