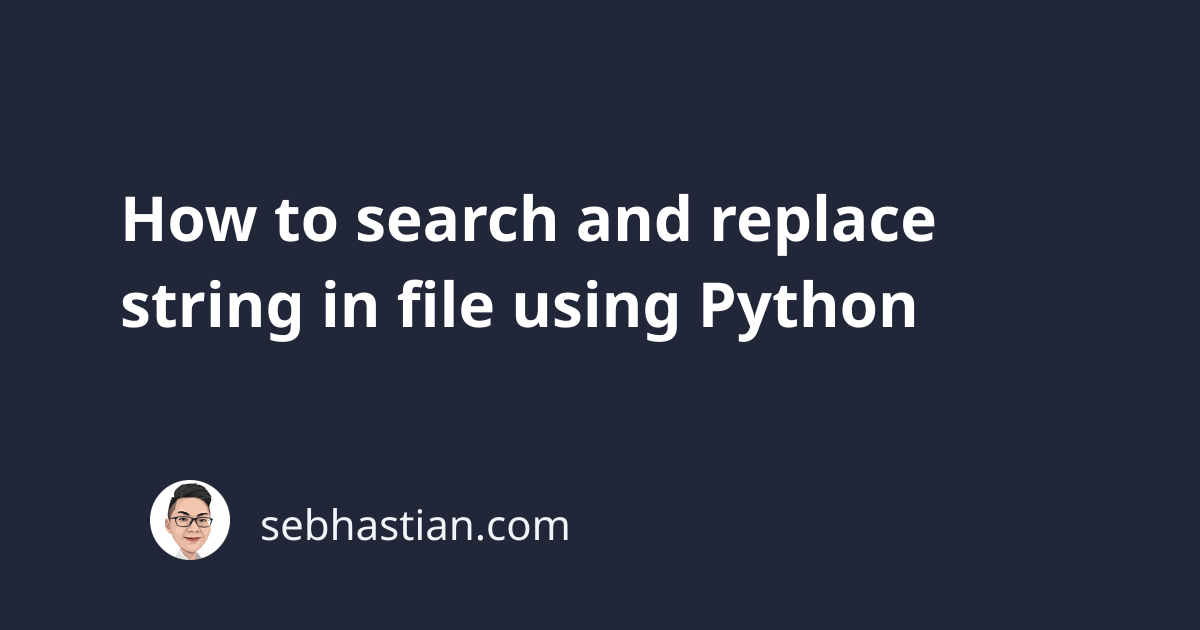
Sometimes, you want to replace a specific string in your file contents with another text.
This tutorial shows how you can use Python to programmatically search and replace strings in a file.
1. Search and replace a string in Python
Suppose we have a text file named example.txt
with the following contents:
Hello, World!
How are you today, World?
Let’s say we want to replace the string World
with a person’s name.
First, You need to use the open()
function to open the file and read its contents.
Then, you can use the replace()
string method to replace a specific text. Here’s how to replace World
with John
:
with open("example.txt") as file:
contents = file.read()
contents = contents.replace("World", "John")
Next, open the same example.txt
again, but this time you need to write the contents
to the file instead of reading:
# Specify `w` to open the file in write mode
with open("example.txt", "w") as file:
file.write(contents)
print("String replace done")
When you run the code above, the string in the example.txt
would be replaced as follows:
Hello, John!
How are you today, John?
As you can see, the string World
is replaced successfully.
If you want to save the old file, you can specify a different file name the second time you call the open()
function as follows:
with open("new_example.txt", "w") as file:
This way, the new content will be saved as new_example.txt
, preserving the old example.txt
file on your disk.
2. Use the open() function in r+ mode
Alternatively, you can also open the file in r+
mode so that you can read and write in one process.
Here’s how to read and write by calling the open()
function once:
with open("example.txt", "r+") as file:
contents = file.read()
contents = contents.replace("World", "John")
# Reset file cursor to the top of the file
file.seek(0)
# Writing the new content to the file
file.write(contents)
# Truncate to clean up the file
file.truncate()
print("String replace done")
First, the r+
mode allows you to read and write to the file you opened.
The seek()
method is used to manually position the file handler. You can reset the handler to the top of the file by passing 0
.
Next, you write the content with replaced string to the file with write()
.
To ensure that any text from before you write the new content is deleted, execute the truncate()
method after writing.
You’ll get the same result as the first option while using the open()
function only once, but you can’t preserve the old file as this solution always writes to the same file.
3. Search and replace a string in a big file
When you have a large file that’s a hundred MB in size, then reading the entire file content at once and replacing the string would be slow.
Instead of reading the whole content using the file.read()
method, use the file.readlines()
method to create a list containing the file contents per line.
Then, you can use a for
loop to iterate over the list and replace the string for each line:
with open("example.txt", "r+") as file:
new_content = ""
for content in file.readlines():
new_content += content.replace("World", "John")
file.seek(0)
file.write(new_content)
file.truncate()
print("String replace done")
Because modern computers come with large memories, you may never need to use the readlines()
method.
But if you find the process slow because your text file is big, then try using this method to optimize the process.
4. Replace multiple strings in one file.
If you have more than one string to replace in your file, then you can create a custom function from the solution above.
Let’s create a function named file_replace()
as follows:
def file_replace(file_name, old, new):
with open(file_name, "r+") as file:
contents = file.read()
contents = contents.replace(old, new)
file.seek(0)
file.write(contents)
file.truncate()
The file_replace()
function accepts three parameters:
file_name
- The name of the file you want to openold
- The string to search fornew
- The string to replace the old string
Whenever you need to replace a string within a file, you just call the function like this:
# 1. Open example.txt and replace World with Jane
file_replace("example.txt", "World", "Jane")
# 2. Open example.txt and replace today with yesterday
file_replace("example.txt", "today", "yesterday")
print("String replace done")
You can call the file_replace()
function as many times as you need.
Conclusion
To replace a string within a file in Python, you need to perform the following steps:
- Use the
open()
function to open, read and write to the file - Use the
str.replace()
method to replace the old string with the new - Write the updated content to the file again
Depending on the result you want to achieve and the size of your file, you can choose to call the open()
function twice so that you can preserve the old file.
You can also optimize the reading of a big file by using the readlines()
and for
loop.
I hope this tutorial is helpful. Feel free to use the code in your project! 👍