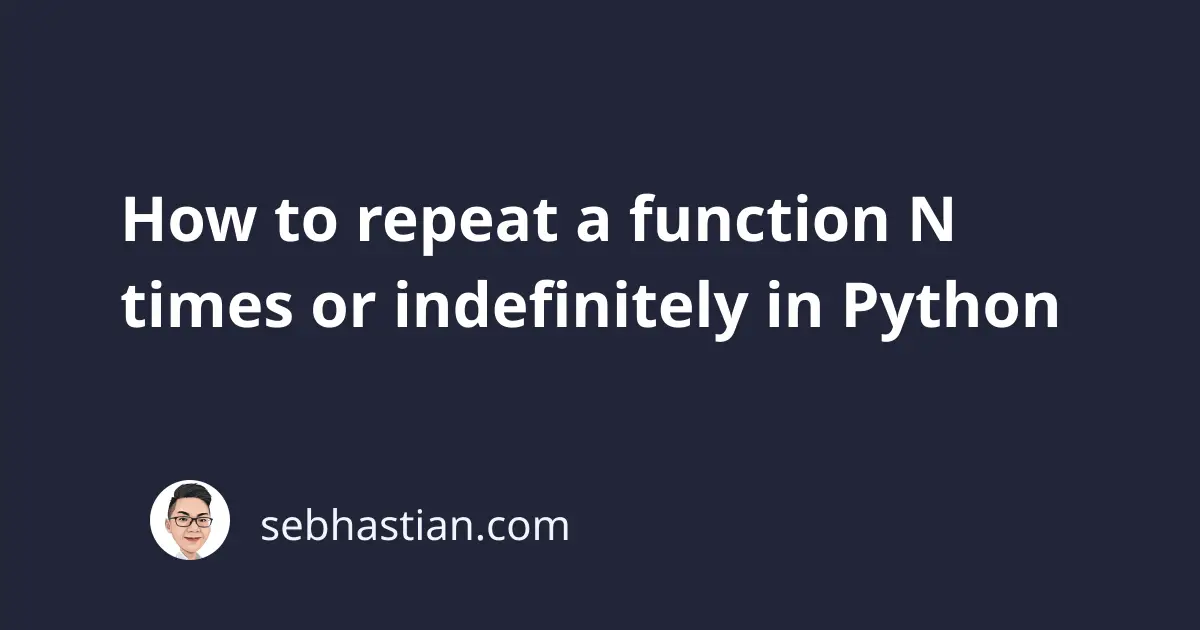
Sometimes, you might require a program that runs a function multiple times.
There are two ways you can repeat a function in Python:
- Use the
for
loop to repeat a function N times - Use the
while
loop to repeat a function indefinitely
This tutorial will show you examples of how to repeat a function in Python.
Repeat a function N times in Python
To repeat a function N times in Python, you need to use the for
loop and range
function.
Suppose you have a function that asks for a number and then multiple that number by 2
. You want to repeat this function ten times.
Here’s how you do it:
def askUser():
val = int(input("Input a number: "))
print(f"The result is: {val * 2}")
n = 10
for i in range(n):
askUser()
print("End of process!")
The syntax for i in range(n)
will repeat the code inside the for
block N times.
You only need to modify the value of n
to make the for
loop repeat as many times as you want.
Repeat a function indefinitely in Python
To repeat a function indefinitely in Python, you need to use the while
loop and call the function in that loop.
To stop the function, you need to write an if
statement with a condition that stops the loop.
For example, the following code asks the user for a number indefinitely until the user enters the word exit
:
def askUser():
val = input("Input a number. To stop, enter 'exit': ")
if val == 'exit':
return val
else:
val = int(val)
print(f"The result is: {val * 2}")
while True:
res = askUser()
if res == 'exit':
break
print("End of process!")
In the code above, the askUser
function will ask for a number indefinitely until the user enters exit
.
This is done by adding an if-else
statement inside the function. If the input equals the word exit
, the function returns that word.
In the while
loop, a break
is executed when the askUser
function returns a string equal to exit
. You can modify the condition for stopping the loop that fits your need.
And that’s how you repeat a function call indefinitely in Python.