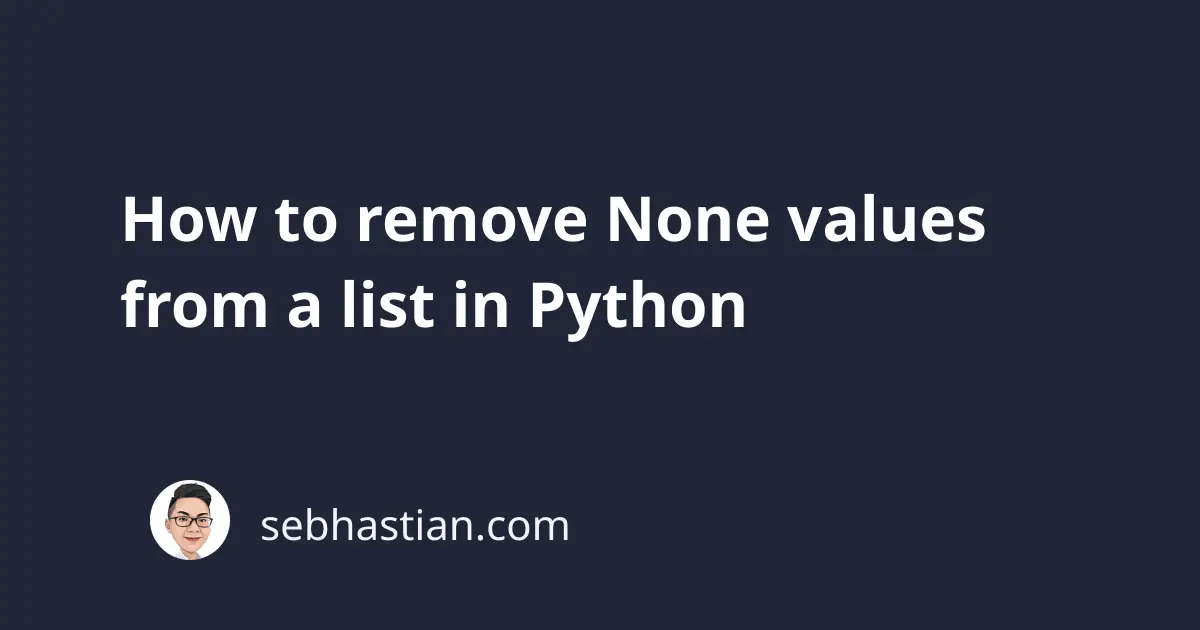
There are three efficient ways you can remove None
values from a list in Python:
- Using the
filter()
function - Using a list comprehension
- Using a loop (
for
orwhile
)
This tutorial will show you how to use the solutions above in practice.
1. Using the filter()
function
Python provides the filter()
function, which you can use to filter items from a list. To remove all None
values from a list, you need to specify None
as the first argument of the filter()
function.
The function returns a filter
object, so you also need to call the list()
function to convert the object into a list object:
original = [0, 2, '', 'Jack', None, 9, None]
new = list(filter(None, original))
print(new)
Output:
[2, 'Jack', 9]
Keep in mind that the filter()
function will return all items that evaluate to True
. Values that evaluate to False
like 0
and an empty string are also excluded from the new list.
Depending on the result you want to achieve, this might not be something you want.
To remove only None
values explicitly, you need to use the other methods.
2. Using a list comprehension
The list comprehension syntax can be used to remove the None
values from your list.
In the list comprehension, you need to specify to return the value if it’s not a None
as follows:
original = [0, 2, '', 'Jack', None, 9, None]
new = [x for x in original if x is not None]
print(new)
Output:
[0, 2, '', 'Jack', 9]
The list comprehension allows you to filter out None
values from the list, but it might be difficult to read a list comprehension once you have a complex condition.
If you also want to perform other tasks while removing the None
values, you might consider using a loop.
Remove None values using a loop
The for
loop is the most straightforward way to remove None
values from a list.
Inside the for
loop, append the item to the new list when the value is not None
like this:
original = [0, 2, '', 'Jack', None, 9, None]
new = []
for item in original:
if item is not None:
new.append(item)
print(new)
Output:
[0, 2, '', 'Jack', 9]
The for
loop works in a similar way to the list comprehension because Python will iterate over the list and evaluate the items one at a time.
The advantage of using a for
loop is that you can add additional operations without sacrificing readability.
For example, suppose you need to convert any string value in the list into its length attribute. You can add the condition to the if
block as follows:
original = [0, 2, '', 'Jack', None, 9, None]
new = []
for item in original:
if item is not None:
if type(item) == str:
item = len(item)
new.append(item)
print(new)
Output:
[0, 2, 0, 4, 9]
As you can see, the empty string is replaced with 0
, while Jack
is replaced with 4
.
Here’s the same code again using a list comprehension:
original = [0, 2, "", "Jack", None, 9, None]
new = [
len(item) if type(item) == str
else item for item in original if item is not None
]
print(new) # [0, 2, 0, 4, 9]
Whoa! Don’t know about you, but I find this example to be quite confusing.
List comprehension is useful because it allows you to replace a for
loop with a one-liner, but it becomes difficult to read when you have multiple conditions.
Some people might place the condition in a separate function like this:
original = [0, 2, "", "Jack", None, 9, None]
def str_to_len(item):
if type(item) == str:
item = len(item)
return item
new = [str_to_len(item) for item in original if item is not None]
print(new)
While the list comprehension is less confusing, you shouldn’t create a function for one-time use. For me, using a for
loop is still easier to read and understand than this.
You can also use a while
loop to replace the for
loop as follows:
original = [0, 2, "", "Jack", None, 9, None]
new = []
item_index = 0
while item_index < len(original):
item = original[item_index]
if item is not None:
if type(item) == str:
item = len(item)
new.append(item)
item_index += 1
print(new) # [0, 2, 0, 4, 9]
But the for
loop syntax is still the most intuitive and easy to understand for me. 😃
Conclusion
This tutorial has shown you how to remove None
values from a list. Depending on your requirements, you can use the filter()
function, the list comprehension, or a for
loop.
I hope this tutorial is useful. Happy coding! 👍