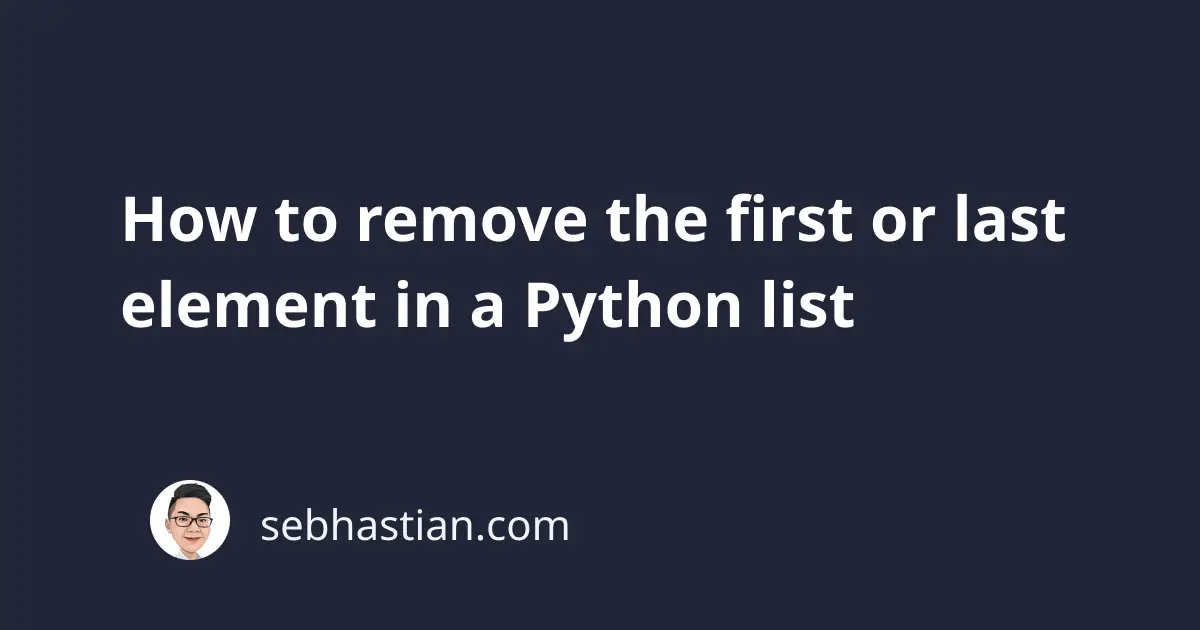
This tutorial will help you learn how to remove the first or last element in a list in practice.
To remove the first element in a Python list, you can use the list.pop()
method and pass 0
as an argument for the method:
my_list = [1, 2, 3, 4, 5]
removed_element = my_list.pop(0)
print(my_list) # [2, 3, 4, 5]
print(removed_element) # 1
To remove the last element in a list, you need to call the pop()
method without passing any argument:
my_list = [1, 2, 3, 4, 5]
removed_element = my_list.pop()
print(my_list) # [1, 2, 3, 4]
print(removed_element) # 5
If you want to remove the first N elements in a list, you can use the list slicing syntax and pass N as the start argument for the slice.
For example, here’s how to remove the first 3 elements in a list:
my_list = [1, 2, 3, 4, 5]
new_list = my_list[3:]
print(my_list) # [1, 2, 3, 4, 5]
print(new_list) # [4, 5]
On the other hand, if you need to remove the last N elements in a list, you need to pass N+1 as the end argument for the slice (after the colon :
symbol).
The example below shows how to remove the last 2 elements from a list:
my_list = [1, 2, 3, 4, 5]
new_list = my_list[:3]
print(my_list) # [1, 2, 3, 4, 5]
print(new_list) # [1, 2, 3]
Here, the elements [4, 5]
are removed from the list.
And that’s how you remove the first or last element, whether one or many, in a list.
I hope this tutorial is useful. Happy coding! 🙌