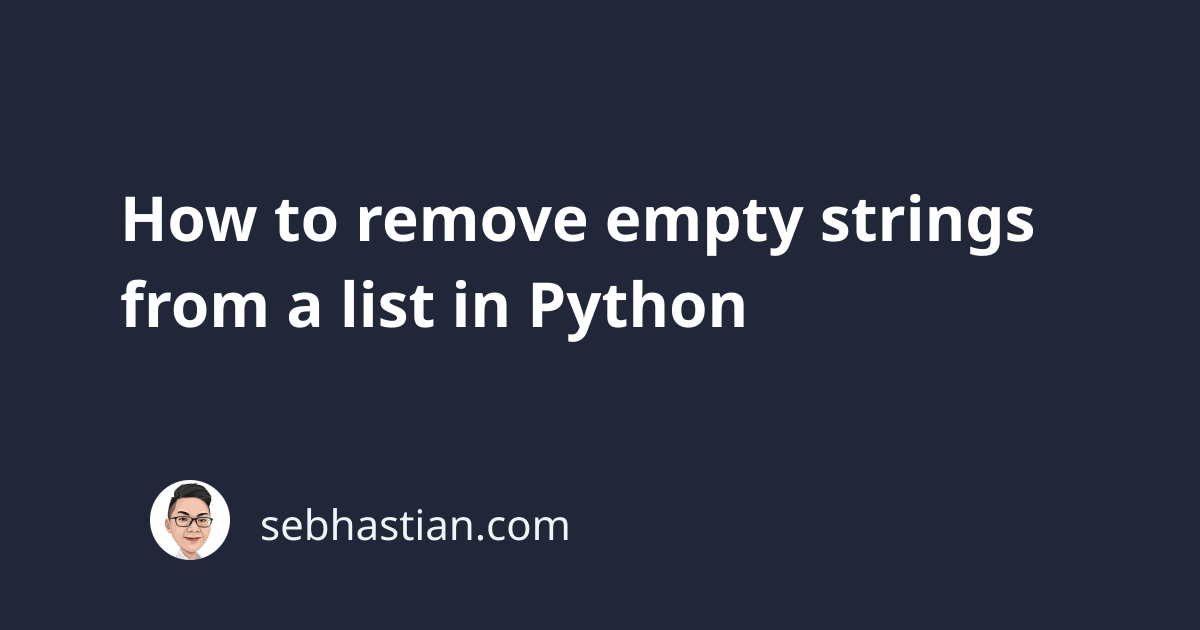
In my experience, there are two easy methods you can use to remove empty strings from a list:
- Use a list comprehension with an
if
conditional statement - Use the
filter()
function
This tutorial will show you how to use both methods in practice.
Using list comprehension to filter empty strings
A list comprehension is a Python technique to create a new list based on an existing list.
In the list comprehension, you can filter the empty strings out by using an if
condition as follows:
my_list = ["a", "", "b", " ", "c", ""]
new_list = [item for item in my_list if item]
print(new_list)
Output:
['a', 'b', ' ', 'c']
In the code above, the item
from my_list
is added to the new_list
only when the if
condition evaluates to True
. Since an empty string evaluates to False
, it will be excluded from the new list.
However, notice that a string containing only whitespace is not excluded because it evaluates to True
as well.
If you want to remove a string that contains whitespace too, you need to call the strip()
method on the item
object like this:
my_list = ["a", "", "b", " ", "c", ""]
new_list = [item for item in my_list if item.strip()]
print(new_list)
Output:
['a', 'b', 'c']
This time, the whitespace string is removed from the new list.
2. Using the filter() function to remove empty strings
Empty strings in a list can also be removed by calling the filter()
function on your list.
When using the filter()
function, you need to pass str.strip
as the first argument and the list as the second argument. See the example below:
my_list = ["a", "", "b", " ", "c", ""]
new_list = list(filter(str.strip, my_list))
print(new_list)
Output:
['a', 'b', 'c']
The filter()
function will apply the function you passed as the first argument to the item in the sequence you passed as the second argument.
When the result is True
, then the item is added to the filter object. Once done, you need to call the list()
function that converts the filter object to a list.
The result is the same as the list comprehension, but some people prefer using the filter()
function because it’s easier to see the intention of the code.
Another SO user also commented that using filter()
is the right way in Python.
As always, you’re free to use the method you liked best. Personally, I still use list comprehension because I’m used to reading it.
I hope this tutorial helps. Happy coding!