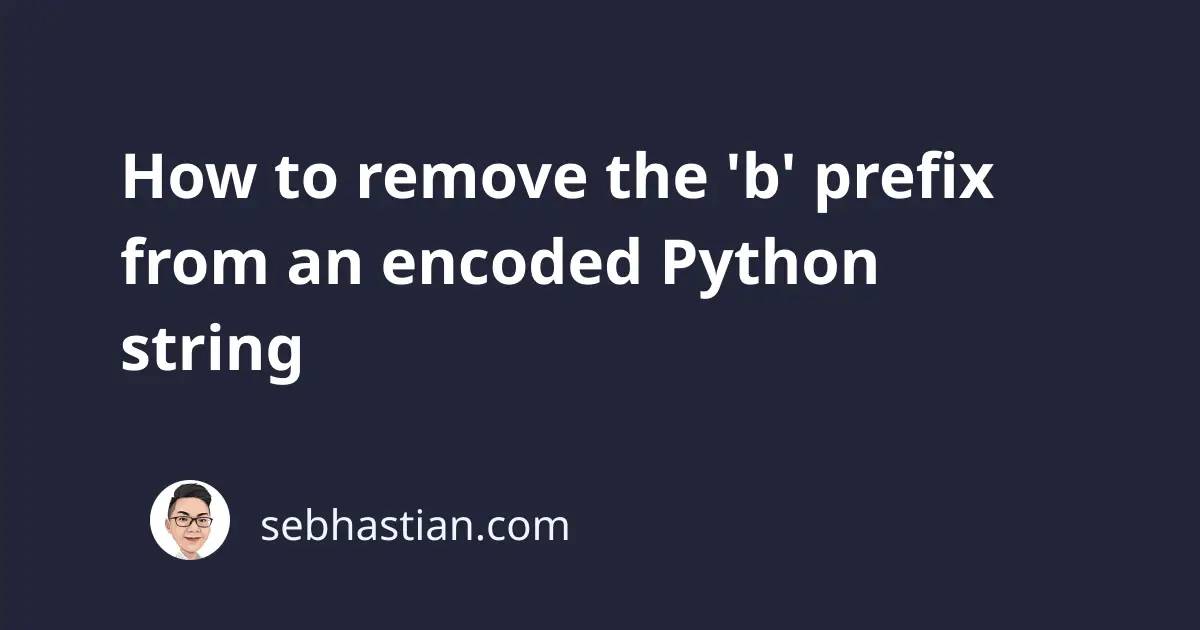
When you print a Python bytes object, you will see a b
prefix in front of the string.
text = b"secret API key"
print(text)
The code above gives the following output:
b'secret API key'
As you can see, there’s a b
prefix in front of the string. This is because the string is actually an encoded bytes object.
To remove the b
prefix from the string, you need to call the decode()
method on the object as shown below:
text = b"secret API key"
plain_string = text.decode()
print(text)
print(plain_string)
Output:
b'secret API key'
secret API key
The bytes object is decoded using the ‘UTF-8’ format by default, so you get the string representation of the bytes object.
As an alternative, you can also use the str()
function to convert the bytes object into a string object as follows:
text = b"secret API key"
plain_string = str(text, encoding='utf-8')
print(text)
print(plain_string)
Output:
b'secret API key'
secret API key
But when you use the str()
function, you need to specify the encoding
argument or you’ll get the same bytes string.
This is the same as decoding the bytes object, so I’d recommend you use the decode()
method because it’s shorter and faster.
I hope this tutorial is helpful. See you in other tutorials!