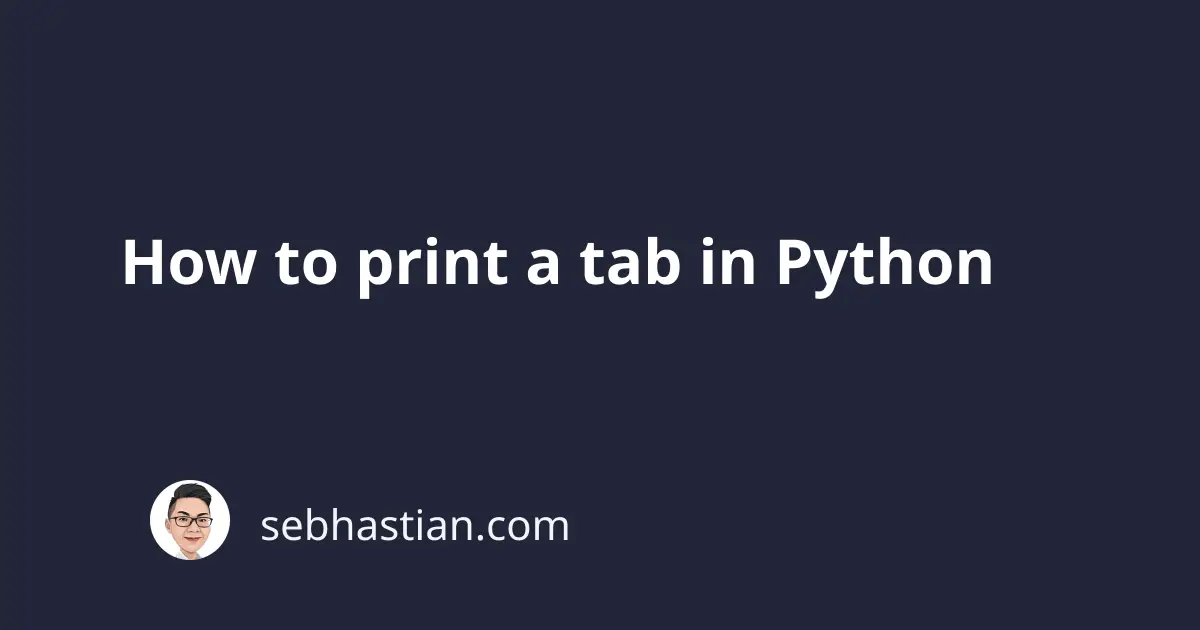
If you want to print a tab in Python, you need to use the \t
escape sequence in your string.
This tutorial shows how you can print tabs in Python for different situations.
Print tabs in a Python string
To print a tab in a Python string, you need to add the \t
escape sequence in the middle of each word in your string.
Here are some examples:
print("Hello\tWorld")
print("Python\tis\tawesome!")
print("1\t2\t3\t4")
Output:
Hello World
Python is awesome!
1 2 3 4
You don’t need to add any white spaces between the words you want to separate using a tab.
Print tabs in a Python list
When you have a list data, you can use the \t
and print
function to insert a tab between the list elements.
See the example below:
p_list = [1, 2, 3]
print(str(p_list[0]) + "\t" + str(p_list[1]) + "\t" + str(p_list[2]))
Alternatively, you can use a for
loop to print a list in one line.
You need to add the sep
(separator) and end
arguments to your print
function as follows:
p_list = [1, 2, 3]
for item in p_list:
print(str(item)+"\t", sep=" ,", end="")
The output will be:
1 2 3
Print tabs in a formatted Python string
When you code a formatted Python string, you can include the \t
character inside the string:
print(f"1\t2\t3")
Output:
1 2 3
But keep in mind that you can’t include a backslash in an f-string expression.
If you try to add a tab between two variables as follows:
name = "Nathan"
age = 29
print(f"{name + '\t' + str(age)}")
Python will show an error:
File ...
print(f"{name + '\t' + str(age)}")
^
SyntaxError: f-string expression part cannot include a backslash
To fix this error, you need to separate the expressions and keep the \t
character outside of the curly brackets.
Here’s one way to fix it:
print(f"{name}\t{str(age)}")
# Output:
# Nathan 29
Sometimes, people also fix this error by putting the \t
character in a variable.
If you put the tab character in a variable, you can use it in the f-string expression part like this:
name = "Nathan"
age = 29
tab = '\t'
print(f"{name + tab + str(age)}") # ✅
Personally, I prefer to put the \t
escape sequence outside of the expression, but feel free to use a variable if you want to.
And that’s how you print a tab in Python. Easy peasy! 👍