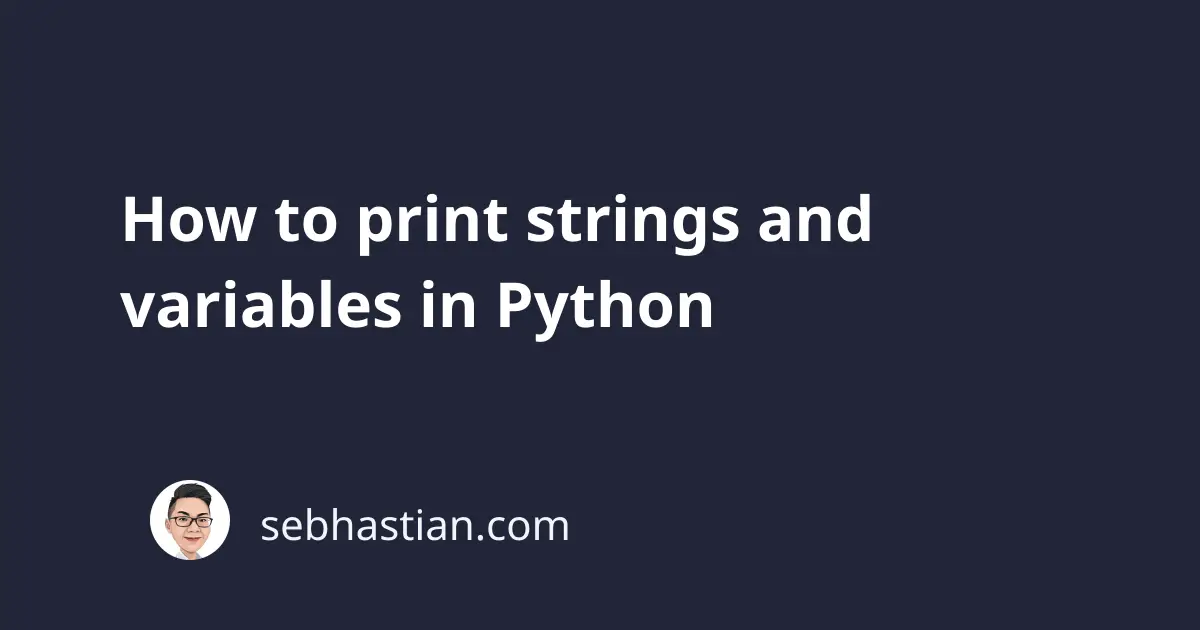
The print()
function in Python is used to generate text output to the terminal.
Most of the time, you need to print some strings together with variable values in Python. This is useful when you need to print some words next to a variable’s value.
In my experience, I found there are four methods you can use to print strings and variables together in one line:
- Using commas to print strings and variables
- Concatenate strings and variables with
+
- Using the f-string format
- Using the
str.format()
method
This article will show you how to use the methods above in practice.
1. Use commas to print strings and variables
The print()
function can accept as many strings or variables as you need in one line. All you need to do is separate each item with a comma.
This example shows how to include a string before a variable:
first_name = "Nathan"
print("Hello", first_name)
Output:
Hello Nathan
If you need to print more than one variable, you can do so by adding the second variable separated by a comma:
first_name = "Nathan"
last_name = "Sebhastian"
print("Hello", first_name, last_name)
Output:
Hello Nathan Sebhastian
You can even add another string to the print()
function:
first_name = "Nathan"
last_name = "Sebhastian"
print("Hello", first_name, last_name, ". How do you do?")
Output:
Hello Nathan Sebhastian . How do you do?
But one weakness of this method is it’s difficult to tell the print()
function when to add a punctuation mark after a variable.
This is because Python prints each element separated by a space.
If you want an easier way to include punctuation marks, you can use concatenation to print strings and variables.
2. Using concatenation (+) to print strings and variables
To print strings and variables with more freedom to format them, you can use the concatenation +
symbol to join both strings and variables.
Here’s an example of concatenating one variable with strings:
first_name = "Nathan"
print("Hello " + first_name + "! How are you?")
Output:
Hello Nathan! How are you?
When using concatenation, you need to add spaces between your strings and variables, or they will be printed without spaces.
While concatenation is useful and gives you more freedom on how to format your text output, it’s not the most recommended way because you need to add two +
symbols and spaces before and after a variable.
This can be confusing for some people. Let’s see how the next method can make things easier.
3. Using the f-string format
The f-string format is my favorite method for printing strings and variables in Python because it’s fast and efficient.
The f-string format allows you to embed any variable inside your string as shown below:
name = "Jack"
topic = "Python"
print(f"{name} is learning {topic} today")
Output:
Jack is learning Python today
As you can see, the f-string format comes in handy when you need to print many variables in one string.
To use the f-string format, you need to add a literal f
character before the string you want to print.
Then, you need to write the name of the variable you want to print inside a set of curly brackets like {name}
and {topic}
variables shown above.
When compared with the other methods, writing the f-string format feels intuitive and fast for me.
4. Using the str.format() method
The str.format()
method is also a good way to print strings and variables in Python, but I prefer it less than the f-string format because the syntax is ugly.
To use the str.format()
method, you need to include braces in the string to substitute for the variables as follows:
name = "Jack"
topic = "Python"
print("{} is learning {} today".format(name, topic))
Output:
Jack is learning Python today
When using the str.format()
method, you need to add curly brackets {}
inside the string to mark the location where you want to insert a variable.
Then, you put the variable in the string by calling the format()
method and passing the variables to that method.
Python reads the variables from left to right, so the first variable you passed to the method will be inserted in the left-most curly brackets.
The reason I prefer the f-string format is that the variables can be embedded directly inside the curly brackets.
You don’t need to include empty curly brackets in the string like when you’re using this method.
Conclusion
Now you’ve learned four different ways to print strings and variables in Python.
Personally, I love using the f-string format because it’s intuitive and easy to read. But feel free to use the method you liked best. There’s no right or wrong way as long as you get to print the text you need.
I hope this tutorial helps. Happy coding! 🙌