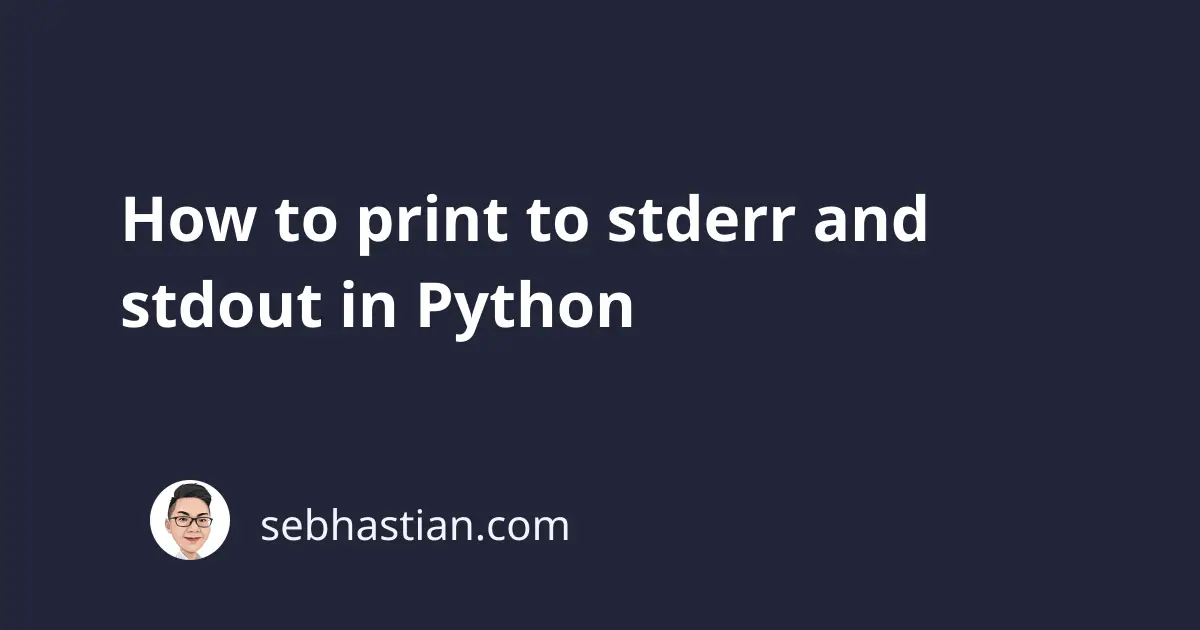
Just like in other programming languages, Python has multiple streams that you can use to receive input and send output.
The stdin or standard input stream is used when you use the input()
function to prompt the user for an input.
The stdout or standard output stream is used when you call the print()
function to print text in the console.
The stderr or standard error stream will be used whenever an exception or error occurs while running your code.
To better maintain and debug your Python program, you can print customized error messages to the stderr. This tutorial will show you some examples to print to the stdout and stderr streams in Python.
First, let’s see how you can print to the stdout stream.
1. Print to stdout
To print to the stdout stream, you simply call the print()
function and specify the text you want to print as its arguments.
Here’s an example:
print("Hello World!", "Good day!", "Thank you!")
Output:
Hello World! Good day! Thank you!
You can also call the sys.stdout.write()
function as follows:
import sys
sys.stdout.write("Hello World!")
sys.stdout.write("Good day!")
sys.stdout.write("Thank you!")
Output:
Hello World!Good day!Thank you!
The sys.stdout.write()
function doesn’t add a new line or space after writing the output, which differs from the print()
function.
Unless you have a unique requirement, I recommend you use the print()
function because you can print multiple outputs in one call.
2. Print to stderr with print()
By default, the print()
function will send the text output to the stdout stream.
To change the stream to stderr, you need to specify the file
argument when calling the print()
function.
Set the file
argument to sys.stderr
like this:
import sys
print("Hello Error!", file=sys.stderr)
Output:
Hello Error!
Notice that there’s no difference when you run the code from the terminal.
3. Using sys.stderr.write() function
The sys.stderr.write()
function is also used to write output to the stderr stream, with a small difference when you run it in the interactive mode.
Consider the output below:
>>> import sys
>>> print("Hello Error!", file=sys.stderr)
Hello Error!
>>> sys.stderr.write("Hello Error!")
12
Hello Error!>>>
The sys.stderr.write()
function prints the number of letters in your output, and it doesn’t print a new line after the text.
4. Use the logging module to print to stderr
The logging
module in Python is used to log events that occur when running your program to the stderr stream.
You need to import the module and call the warning()
function as follows:
import logging
logging.basicConfig(format='%(message)s')
log = logging.getLogger(__name__)
log.warning('Hello Error!')
Using the log.warning()
function will send the output to stderr stream.
Conclusion
Now you’ve learned how the stdout and stderr stream can be used to print outputs in Python.
The standard error stream is created to be independent of the standard output stream. Using the stderr stream allows you to log the events in your program before it reaches an error that stops the program.
I hope this tutorial is helpful. See you in other tutorials! 👍