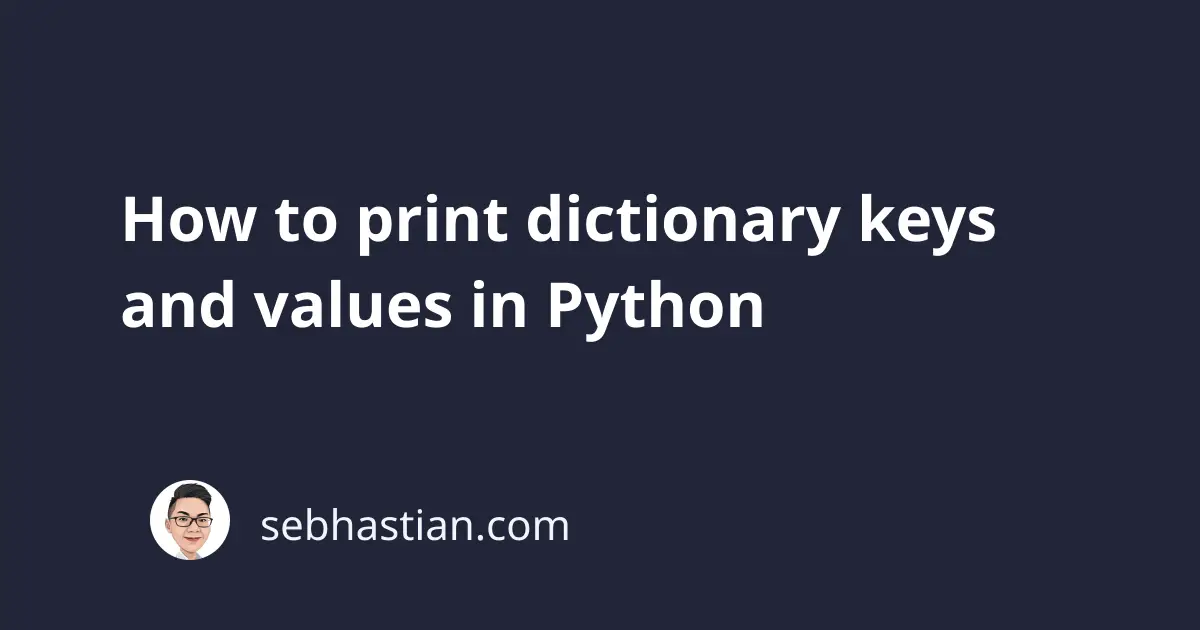
There are two methods you can use to print a dictionary keys and values in Python:
- Using the
items()
method and afor
loop - Using just a
for
loop
In this tutorial, I will show you how to use both options in practice.
1. Using the items() and a for loop
The dictionary object has a method called items()
that returns a list containing the key-value tuples.
You can then use a for
loop to iterate over the list and print each tuple as shown below:
my_dict = {
"name": "Nathan",
"age": 29,
"role": "Programmer"
}
for key, value in my_dict.items():
print(f"{key} : {value}")
Output:
name : Nathan
age : 29
role : Programmer
This approach is convenient and intuitive, but the dict.items()
method is only available in Python 3.
If you need to support Python 2, you need to use the more traditional approach.
2. Print dictionary keys and values using a for
loop
The for
loop alone can be used to print a dictionary keys and values.
You need to iterate over the dictionary object and print each key-value pair as follows:
my_dict = {
"name": "Nathan",
"age": 29,
"role": "Programmer"
}
for key in my_dict:
print(f"{key} : {my_dict[key]}")
In Python, using a for
loop with a dictionary object returns only the key, so you can access the value in the loop using the traditional dict[key]
syntax.
This approach is easy to write and available for all Python versions.
Conclusion
Printing a dictionary keys and values in Python is an easy task that you can do in two ways.
You can use the items()
method and a for
loop, or you can just use the for
loop as shown in this tutorial.
I hope this tutorial helps. See you in other tutorials! 👋