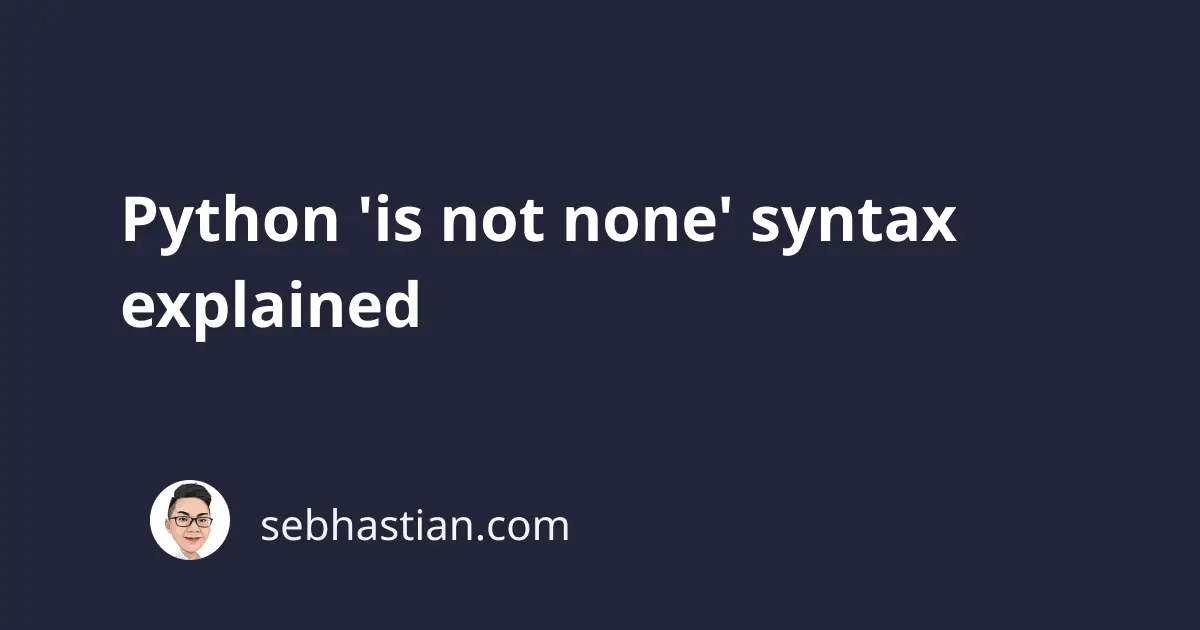
In Python, the is not None
syntax is used to check if a variable or expression is not equal to None.
The None
keyword in Python represents the absence of a value or a null value.
The is not
operator returns True
when the left-hand operator is not equal to the right-hand operator:
x = None
if x is not None:
print("x is not None")
else:
print("x is None") # ✅
In the above example, the variable x
is assigned the value of None
. The if
statement checks if x
is not equal to None
by using the is not
operator.
In this case, the code will print x is None
because x
is assigned the value of None
.
You can also use the equality comparison operator !=
to replace the is not
operator as follows:
x = None
if x != None:
print("x is not None")
else:
print("x is None") # ✅
Since None
is a singleton object, it is possible to use the is not
operator to check if a variable is None
instead of using the !=
operator.
The is
or is not
operator checks for object identity, which is faster than the ==
or !=
operator that checks for the equality of the object values.
What’s more, the ==
result is always True
when is
returns True
(but not the opposite)
Let’s see an example that shows the differences:
x = [1, 2, 3]
y = [1, 2, 3]
print(x == y) # True
print(x is y) # False
When you check if the value of x
and y
is equal using the ==
operator, Python returns True
because they have the same value of [1, 2, 3]
.
But when you check if the object identity of x
is the same as y
, Python returns False
because they are different objects, stored at different memory points.
You can use the id()
function to check for the identification number that points to the object in memory as follows:
x = [1, 2, 3]
y = [1, 2, 3]
print(id(x)) # 4341386624
print(id(y)) # 4342199296
The ID numbers may be different when you run the code above, but the x
and y
numbers will never be the same.
On the other hand, the ID numbers for the None
object is always the same:
x = None
y = None
print(id(x)) # 4375967240
print(id(y)) # 4375967240
It’s recommended to use the is not
operator instead of !=
operator when checking for None
.
Next, you can also use the is not None
operator in a function that returns a value only if the input is not None:
def add_numbers(x, y):
if x is not None and y is not None:
return x + y
else:
return None
result = add_numbers(2, 3)
print(result) # 5
result = add_numbers(None, 3)
print(result) # None
In this example, the function add_numbers
takes two arguments and returns the sum of the two numbers if both arguments are not None
. Otherwise, it returns None
.
Finally, it’s also possible to use the None
keyword in the condition of a ternary operator as follows:
x = None
result = "x is not None" if x is not None else "x is None"
print(result) # x is None
Conclusion
To conclude, the is not None
syntax is a way to check if a variable or expression is not equal to None
in Python.
Combined with the if
statement, this logic can be useful in situations where you have a function that requires a certain variable to be not None
.
It can also be used when you want to return a value only if the input is not None
. It’s recommended to use the is
operator instead of the ==
operator when checking for None
.