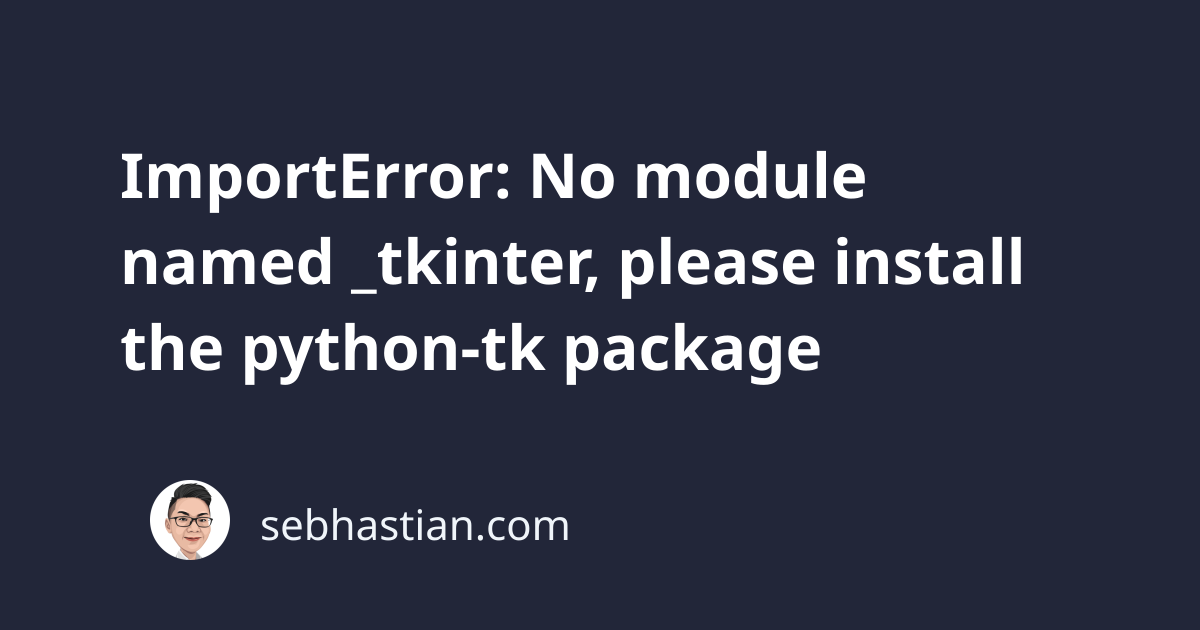
When running Python on Linux-based OSes, you may encounter an error that says:
ImportError: No module named _tkinter, please install the python-tk package
This error happens because the Tkinter package can’t be found on your computer system.
There are several solutions you can try to fix this error, based on the Operating System you used:
Fix No module named _tkinter on Linux
To fix this error on Linux-based OS, you need to install python-tk
on your system.
Run the following command from the terminal:
# For Python 2:
sudo apt-get install python-tk
# For Python 3:
sudo apt-get install python3-tk
# CentOS alternative:
sudo yum install tkinter
# Fedora alternative:
sudo dnf install python3-tkinter
Once the Tkinter package was installed, you can import the package into your code.
Fix No module named _tkinter on macOS
If you see this error while running Python on macOS, then you need to install python-tk
with homebrew:
brew install python-tk
Once you installed the package, you should be able to import tkinter
in your code:
import tkinter
tkinter._test()
Fix No module named _tkinter on Windows
This error shouldn’t happen in Windows because Tkinter was bundled with Python.
But if you see this error on Windows, then try installing the tk
package with pip
:
pip install tk
# or
pip3 install tk
If that doesn’t work, run Python .exe
installer and select the Modify menu.
You need to make sure that the tcl/tk feature is selected as follows:
Finish the installation setup and you should be able to use the Tkinter package now.
Make sure that the import statement is correct
If you still see this error after installing Tkinter, then you may have an import
statement that’s incompatible with your Python version.
In Python version 2, you need to import Tkinter
with a capital T
. In Python 3, you import the package will all lowercase as import tkinter
.
You can use a try-except
block to make your code runs in both Python 2 and 3 as follows:
try:
# For Python 2
import Tkinter as tk
except ImportError:
# For Python 3
import tkinter as tk
tk._test()
The code above instructs Python to import the tkinter
package when the Tkinter
package was not found.
Don’t forget to add the same alias so that the code you write still works no matter which library was imported.
Conclusion
The error ImportError: No module named _tkinter
occurred because the Tkinter package was not found on your computer system.
Several Linux-based distributions separate the Tkinter package from the standard libraries included in Python, so you need to install it manually.
Once Tkinter is installed, this error should be resolved.
I hope this article was helpful. Happy coding! 👍