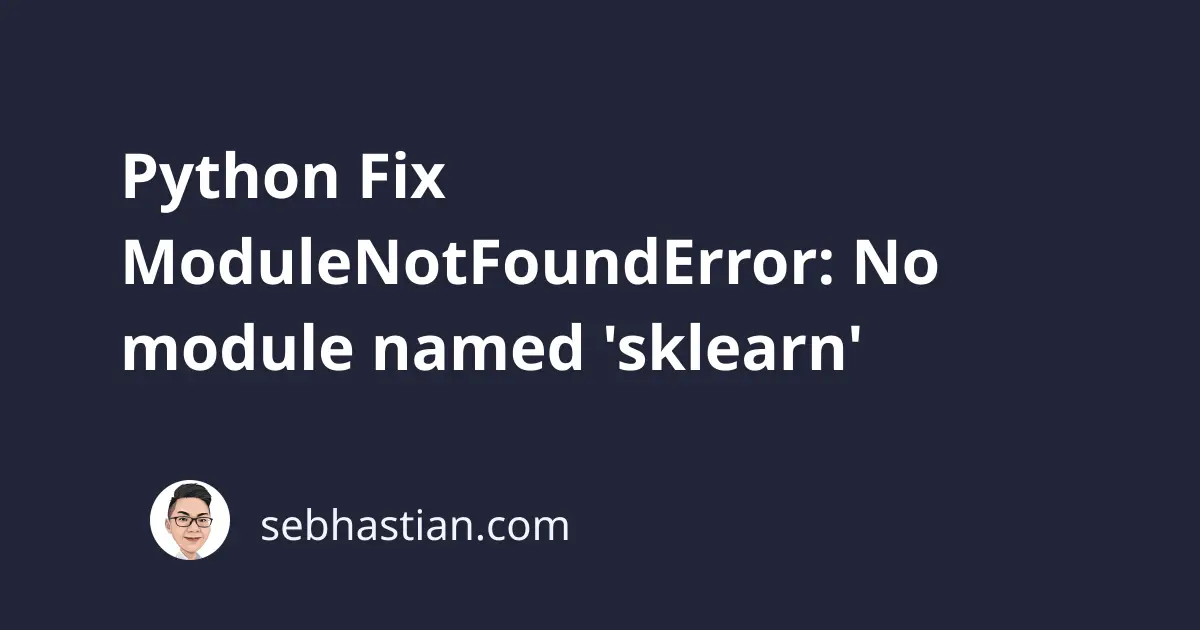
When running Python code, sometimes you will see an error saying ModuleNotFoundError: No module named 'sklearn'
.
This error means that Python can’t find the sklearn
module. You may not have the sklearn
module installed, or the module is installed in a different environment.
The example error log is as follows:
Traceback (most recent call last):
File ...
import sklearn
ModuleNotFoundError: No module named 'sklearn'
To fix this error, you need to install the sklearn
package using pip
or conda
.
First, let’s see how to install sklearn
with pip
. You need to run one of the following commands:
# Use pip to install scikit-learn
pip install scikit-learn
# Or pip3
pip3 install scikit-learn
# If pip isn't available in PATH
python -m pip install scikit-learn
# Or with python3
python3 -m pip install scikit-learn
# For Windows without pip in PATH
py -m pip install scikit-learn
Once the installation has finished, you should be able to import the sklearn
module in your code successfully.
When you are running Python from Jupyter Notebook, add the !
operator before pip
like this:
!pip install -U scikit-learn
# or
!conda install -c anaconda scikit-learn
If you installed Python from Anaconda distribution, then you might want to install the sklearn
package from conda with the following command:
conda install scikit-learn
If you still see the error, it means the Python interpreter still can’t find the sklearn
module.
One of the following scenarios may happen in your case:
- You have multiple versions of Python installed on your system, and you are using a different version of Python than the one where
scikit-learn
is installed. - You might have
scikit-learn
installed in a virtual environment, and you are not activating the virtual environment before running your code.
Let’s see how to fix these errors.
Case#1 - You have multiple versions of Python
If you have multiple versions of Python installed on your system, you need to make sure that you are using the specific version where the scikit-learn
package is installed.
You can test this by running the which -a python
or which -a python3
command from the terminal:
$ which -a python
/opt/homebrew/bin/python
/usr/bin/python
In the example above, there are two versions of Python installed on /opt/homebrew/bin/python
and /usr/bin/python
.
Suppose you run the following steps in your project:
- Install
scikit-learn
withpip
using/usr/bin/
Python version - Install Python using Homebrew, now you have Python in
/opt/homebrew/
- Then add
import scikit-learn
in your code
The steps above will cause the error because scikit-learn
is installed in /usr/bin/
, and your code is probably executed using Python from /opt/homebrew/
path.
To solve this error, you need to run pip install scikit-learn
command again so that the package is installed and accessible by the new Python version.
Finally, keep in mind that you can also have pip
and pip3
available on your computer.
The pip
command usually installs module for Python 2, while pip3
installs for Python 3. Make sure that you are using the right command for your situation.
Next, you can also have the package installed in a virtual environment.
Case#2 - You are using Python virtual environment
Python venv
package allows you to create a virtual environment where you can install different versions of packages required by your project.
If you are installing scikit-learn
inside a virtual environment, then the module won’t be accessible outside of that environment.
Even if you never run the venv
package, Python Anaconda distribution usually creates a virtual enviroment automatically when you install it on your computer.
You can see if a virtual environment is activated or not by looking at your command prompt.
When a virtual environment is activated, the name of that environment will be shown inside parentheses as shown below:
In the picture above, the name of the virtual environment (base)
appears when the Conda virtual environment is activated.
Usually, a Conda virtual environment already has the scikit-learn
module active. You can check this in the Anaconda Environments tab as shown below:
If you see scikit-learn
installed but the environment is not activated, then run the command below to activate it:
conda activate <env>
# For base env:
conda activate base
Once the base
environment is activated, you should be able to import sklearn
module.
Conclusion
To conclude, the ModuleNotFoundError: No module named 'scikit-learn'
error occurs when the scikit-learn
package is not available in your Python environment.
To fix this error, you need to install scikit-learn
using pip
or conda
.
If you already have the module installed, make sure you are using the correct version of Python, or activate the virtual environment if you have one.
By following these steps, you should be able to import scikit-learn
modules in your code successfully.