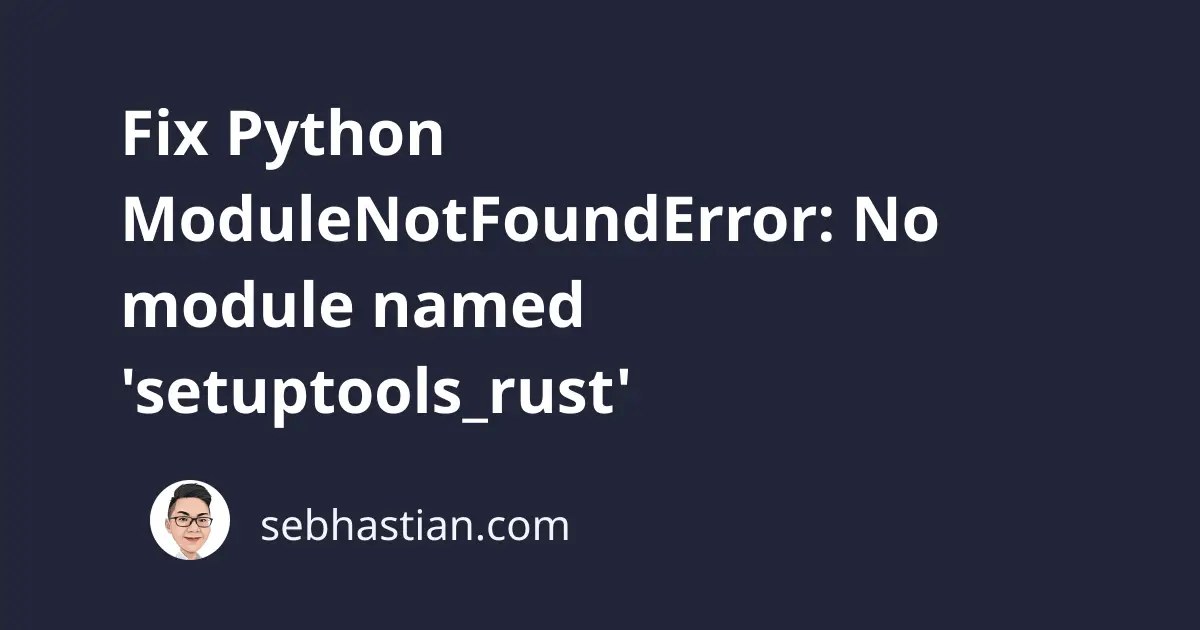
Python shows the ModuleNotFoundError: No module named 'setuptools_rust'
message when it cannot find the package setuptools_rust
in your Python environment.
To fix this error, you need to install the package explicitly using pip
. This tutorial will show you how to resolve this error.
Installing setuptools_rust
Suppose you add the following import
statement to your code:
import setuptools_rust
And you see the following error message:
This means Python can’t find the setuptools_rust
package under the current Python environment.
The solution is to install the package using pip
, which is the package installer for Python.
If you don’t have pip
installed, follow the pip installation guide to make it available on your computer.
Next, use one of the following commands to get setuptools_rust
on your computer or server:
# Upgrade pip to the latest version first
pip install --upgrade pip
# Use pip to install setuptools_rust
pip install setuptools_rust
# Or pip3
pip3 install setuptools_rust
# If pip isn't available in PATH
python -m pip install setuptools_rust
# Or with python3
python3 -m pip install setuptools_rust
# For Windows without pip in PATH
py -m pip install setuptools_rust
# for Anaconda
conda install -c anaconda setuptools_rust
# for Jupyter Notebook
!pip install setuptools_rust
For Linux-based OS like CentOS, you may need to use the sudo
command to install the package:
sudo pip3 install --upgrade pip
sudo pip3 install setuptools-rust
# or
sudo pip install --upgrade pip
sudo pip install setuptools-rust
Once you installed setuptools-rust
successfully, you should be able to run the code with the import
statement with no error.
If that doesn’t work, then you might have one of the following scenarios happen:
- You have multiple versions of Python installed on your system, and you are using a different version of Python than the one where
setuptools-rust
is installed. - You might have
setuptools-rust
installed in a virtual environment, and you are not activating the virtual environment before running your code. - Your IDE uses a different version of Python from the one that has
setuptools-rust
Let’s see how to fix these errors.
Issue with multiple versions of Python
If you have multiple versions of Python installed on your system, you need to make sure that you are using the specific version where the setuptools-rust
package is installed.
You can test this by running the which -a python
or which -a python3
command from the terminal:
$ which -a python3
/opt/homebrew/bin/python3
/usr/bin/python3
In the example above, there are two versions of Python installed on /opt/homebrew/bin/python3
and /usr/bin/python3
.
Suppose you run the following steps in your project:
- Install
setuptools-rust
withpip
using/usr/bin/
Python version - Install Python using Homebrew, now you have Python in
/opt/homebrew/
- Then add
import setuptools-rust
in your code
The steps above will cause the error because setuptools-rust
is installed in /usr/bin/
, and your code is probably executed using Python from /opt/homebrew/
path.
To solve this error, you need to run pip install setuptools-rust
command again so that the package is installed and accessible by the new Python version.
Next, you can also have the package installed in a virtual environment.
Issue with Python virtual environment
Python venv
package allows you to create a virtual environment where you can install different versions of packages required by your project.
If you are installing setuptools-rust
inside a virtual environment, then the module won’t be accessible outside of that environment.
You can see if a virtual environment is activated or not by looking at your command prompt.
When a virtual environment is activated, the name of that environment will be shown inside parentheses as shown below:
In the picture above, the name of the virtual environment (base)
appears when the Conda virtual environment is activated.
Python IDE like Anaconda and PyCharm usually create their own virtual environment when you create a Python project with them.
To solve this, you can either:
- Turn off the virtual environment so that
pip
installs to your computer - Install the required package in that virtual environment with
pip
You can choose the solution that works for your project.
When your virtual environment is created by Conda, run the conda deactivate
command. Otherwise, running the deactivate
command should work.
For more detail on creating, running, and deactivating Python virtual environment, you can read this venv introduction article.
Issue with IDE using a different Python version
Finally, the IDE from where you run your Python code may use a different Python version when you have multiple versions installed.
For example, you can check the Python interpreter used in VSCode by opening the command palette (CTRL + Shift + P
for Windows and ⌘ + Shift + P
for Mac) then run the Python: Select Interpreter
command.
You should see all available Python versions listed as follows:
You need to use the same version where you installed setuptools-rust
so that the module can be found when you run the code from VSCode.
Once done, you should be able to import setuptools-rust
into your code.
Conclusion
In summary, the ModuleNotFoundError: No module named 'setuptools-rust'
error occurs when the setuptools-rust
packages are not available in your Python environment.
To fix this error, you need to install setuptools-rust
using pip.
If you already have the module installed, make sure you are using the correct version of Python, deactivate the virtual environment if you have one, and check for the Python version used by your IDE.
By following these steps, you should be able to import setuptools-rust
modules in your code successfully.