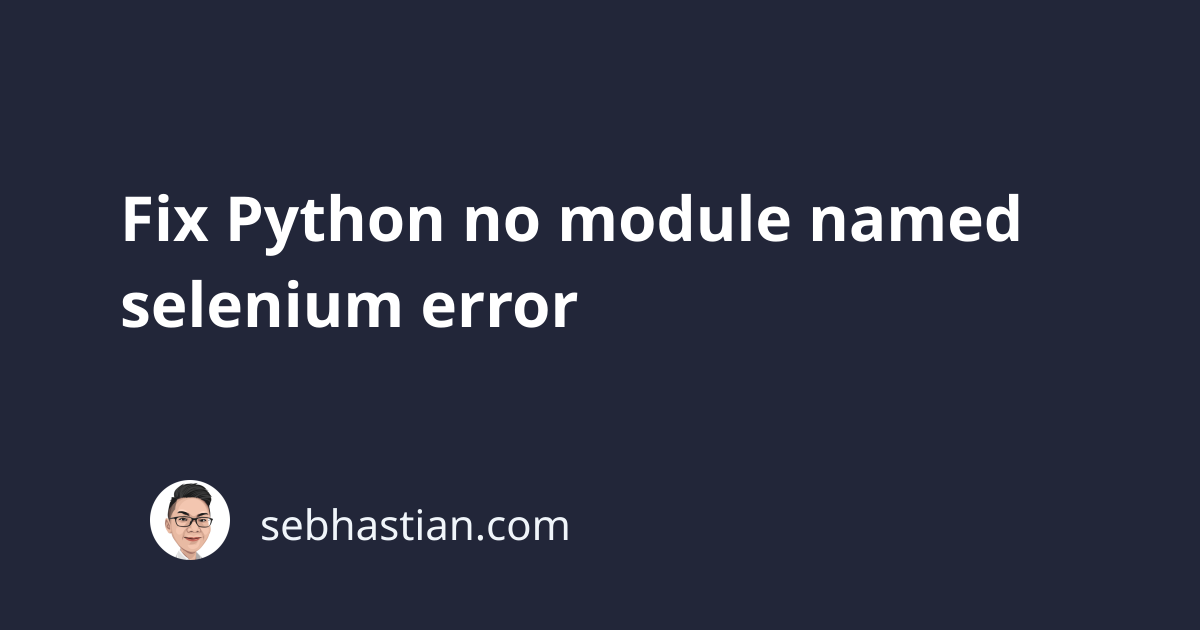
Python shows no module named selenium
error when it can’t find the selenium
library you imported in your code.
This can mean that you don’t have selenium installed, or you installed it for a different version of Python (if you have multiple Python versions)
This article shows simple steps you can do to fix this error.
Why no module named selenium error occurs
This error occurs when you try to import the selenium
library in your code like this:
import selenium
#or
from selenium import webdriver
When Python can’t find the library, it raises the following error:
Traceback (most recent call last):
File ...
import selenium
ModuleNotFoundError: No module named 'selenium'
Because selenium
isn’t a standard Python library, you need to install it using pip
before you can import it.
Here’s the command to install selenium with pip:
pip install selenium
# or if you have Python 3
pip3 install selenium
Once you have selenium
library installed, you should be able to run the code without any errors.
If that doesn’t work, then you may have installed multiple versions of Python on your computer.
One of the following scenarios may happen in your case:
- You have multiple versions of Python installed on your system, and you are using a different version of Python than the one where
selenium
is installed. - You might have
selenium
installed in a virtual environment, and you are not activating the virtual environment before running your code. - Your IDE uses a different version of Python from the one that has
selenium
installed
Let’s see how to fix these errors.
Case#1 - You have multiple versions of Python
If you have multiple versions of Python installed on your system, you need to make sure that you are using the specific version where the selenium
package is installed.
You can test this by running the which -a python
or which -a python3
command from the terminal:
$ which -a python
/opt/homebrew/bin/python
/usr/bin/python
In the example above, there are two versions of Python installed on /opt/homebrew/bin/python
and /usr/bin/python
.
Suppose you run the following steps in your project:
- Install
selenium
withpip
using/usr/bin/
Python version - Install Python using Homebrew, now you have Python in
/opt/homebrew/
- Then add
import selenium
in your code
The steps above will cause the error because selenium
is installed in /usr/bin/
, and your code is probably executed using Python from /opt/homebrew/
path.
To solve this error, you need to run pip install selenium
command again so that the package is installed and accessible by the new Python version.
Finally, keep in mind that you can also have pip
and pip3
available on your computer.
These days, the pip
command is used to install modules for Python 2, while pip3
installs for Python 3. Make sure that you are using the right command for your situation.
Next, you can also have the package installed in a virtual environment.
Case#2 - You are using Python virtual environment
Python venv
package allows you to create a virtual environment where you can install different versions of packages required by your project.
If you are installing selenium
inside a virtual environment, then the module won’t be accessible outside of that environment.
Even when you never run the venv
package, Python IDE like Anaconda and PyCharm usually create their own virtual environment when you create a Python project with them.
You can see if a virtual environment is activated or not by looking at your command prompt.
When a virtual environment is activated, the name of that environment will be shown inside parentheses as shown below:
In the picture above, the name of the virtual environment (base)
appears when the Conda virtual environment is activated.
To solve this, you can either:
- Turn off the virtual environment so that
pip
installs to your computer - Install the
selenium
in the virtual environment withpip
You can choose the solution that works for your project.
When your virtual environment is created by Conda, run the conda deactivate
command. Otherwise, running the deactivate
command should work.
To activate your virtual environment, use one of the following commands:
# For Conda:
conda activate <env_name>
# For venv:
source <env_name>/bin/activate
For Pycharm, you need to follow the Pycharm guide to virtual environment.
Case#3 - IDE using a different Python version
Finally, the IDE from where you run your Python code may use a different Python version when you have multiple versions installed.
If you use VSCode, run the steps below to check available Python interpreters:
- Open the command palette (
CTRL + Shift + P
for Windows and⌘ + Shift + P
for Mac) - Run the
Python: Select Interpreter
command.
You should see all available Python versions listed as follows:
You need to use the same version where you installed selenium
so that the module can be found when you run the code from VSCode.
If you use Pycharm, follow the Pycharm configuring Python interpreter guide.
Once done, the import selenium
statement shouldn’t cause any error.
Conclusion
To conclude, the ModuleNotFoundError: No module named 'selenium'
error occurs when the selenium
package is not available in your Python environment.
To fix this error, you need to install selenium
using pip
.
If you already have the module installed, make sure you are using the correct version of Python, activate the virtual environment if you have one, and check for the Python version used by your IDE.
By following these steps, you should be able to import selenium
modules without any error.