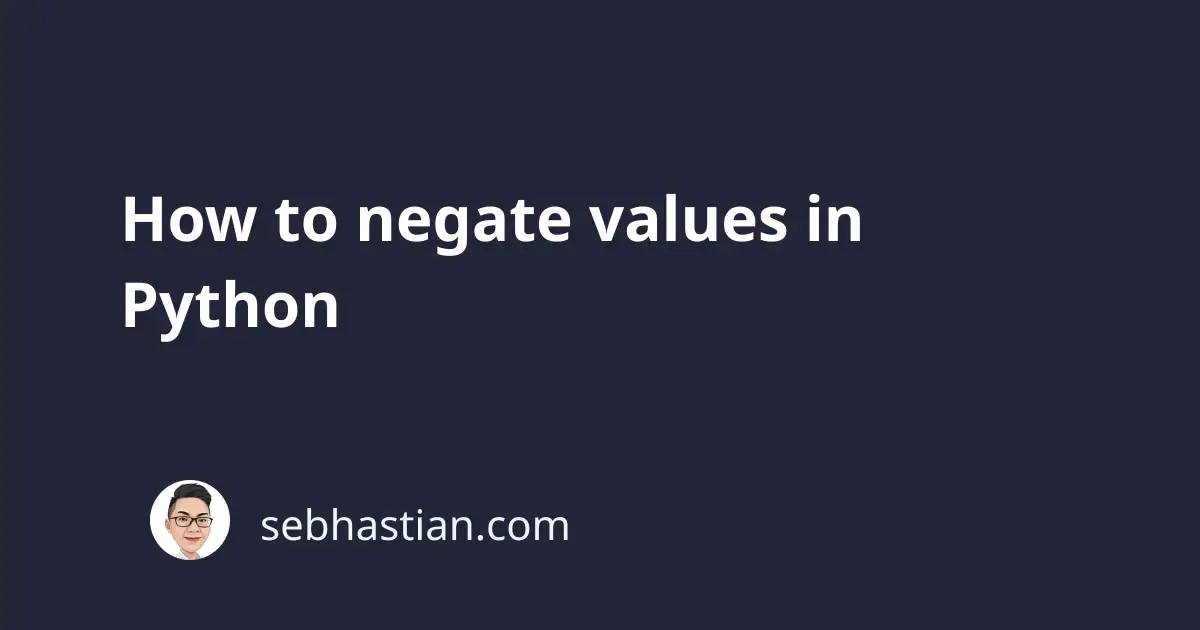
The not
operator is used to negate or reverse values in Python.
The easiest example of using this operator is when you have a boolean value as follows:
val = True
print(not val) # False
The value True
is flipped to False
using the not
operator.
You can use the not
operator to flip a list of boolean values like this:
the_list = [False, True, False, True]
new_list = [not item for item in the_list]
print(new_list) # [True, False, True, False]
The not
operator is commonly used when you need to check for a specific condition in your code.
You can check if a variable is the same as another variable, reverse a value from True
to False
and vice versa, and check if a certain value exists in a collection.
There are two ways you can write a condition using this operator:
x = True
y = False
# 1. Negate positive expression
if not x is y:
pass
# 2. Inline negation of an expression
if x is not y:
pass
Both negations in the example above achieve the same result, but the second way is easier to read and understand than the first one.
This small difference can make a big impact on the readability of your code.
For example, suppose you want to test if a certain value exists in a list.
You can do it in two ways like this:
numbers = [1, 2, 3, 4]
print(not 5 in numbers)
print(5 not in numbers)
Again, the results are the same, but the second way of including not
is easier to read than the former, right?
The not
operator is very useful for checking the truth value of expressions. It can help you to decide the course of action available in your application.