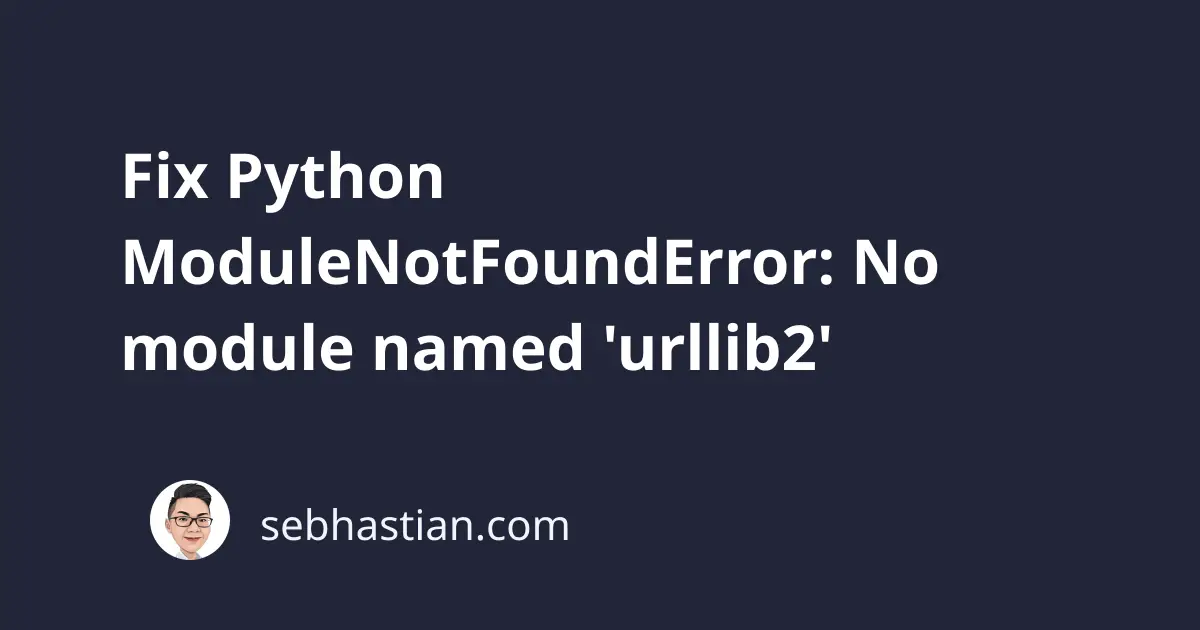
When running Python code that makes use of the urllib2
module, you might see the following error:
ModuleNotFoundError: No module named 'urllib2'
This article explains why the error above occurs and how you can fix it.
Why this error occurs
Python shows ModuleNotFoundError: No module named 'urllib2'
when you try to import the urllib2
module in Python 3.
The urllib2
module was used in Python to open and read Internet contents using the URL identifier.
But in Python 3, the urllib2
module was split into several modules named urllib.request
, urllib.error
, urllib.parse
, and urllib.robotparser
. More modules might be added in the future if needed.
Suppose you run the following code in Python 3:
from urllib2 import urlopen
response = urlopen("https://example.com")
Python will give you the following error message:
Traceback (most recent call last):
File ...
from urllib2 import urlopen
ModuleNotFoundError: No module named 'urllib2'
To fix this error, you need to import urllib.request
instead of urllib2
.
How to fix ModuleNotFoundError: No module named ‘urllib2’
Replace the urllib2
import in your code as shown below:
from urllib.request import urlopen
response = urlopen("https://example.com")
Notice how the import was changed from urllib2
to urllib.request
in the code above.
You can see all available classes and functions in the urllib.request official documentation.
Use try-except block to make your code adaptable
If you still want to support Python 2 interpreter, then you can write a try-except
block that handles the module import.
Consider the code below:
try:
# Try import Python 2 module
import urllib2 as req
except:
# urllib2 not found, import from urllib.request
import urllib.request as req
# You can define the code that uses the module below
response = req.urlopen("https://example.com").read()
print(response)
In the try
block, you are instructing Python to import the urllib2
module and give it an alias name.
When the import fails, Python will run the except
block where it will import from urllib.request
and use the same alias name.
Using an alias will ensure that your code still runs no matter what module gets imported.
Use 2to3 tool to refactor your code automatically
The Python 2to3
module is a program that can rewrite your Python code to make it Python 3 compatible.
This tool automatically converts urllib2
import into the appropriate urllib
module.
This module should already be included when you install Python, but you can install the module using pip
as follows:
pip install 2to3
# or
pip3 install 2to3
Once installed, you can run the module from the terminal.
Suppose you have a file named script.py
, here’s how you convert it:
2to3 -w script.py
The -w
option is added so that the module will save the changes to the file.
Without -w
option, Python only shows the changes you need to make in the terminal.
When it’s done, you’ll see the .py
file modified, and a backup file script.py.bak
will be generated.
Conclusion
This article has shown you that the ModuleNotFoundError: No module named 'urllib2'
occurs when you import the urllib2
module in Python 3.
To fix this error, you can refactor the import statement to use urllib.request
, use a try-except
block to support Python 2 and 3 environments, and use the 2to3
module to convert Python 2 code to Python 3 compatible.
Now you’ve learned how to fix ModuleNotFoundError: No module named 'urllib2'
that occurs in Python 3. Cheers! 🍻