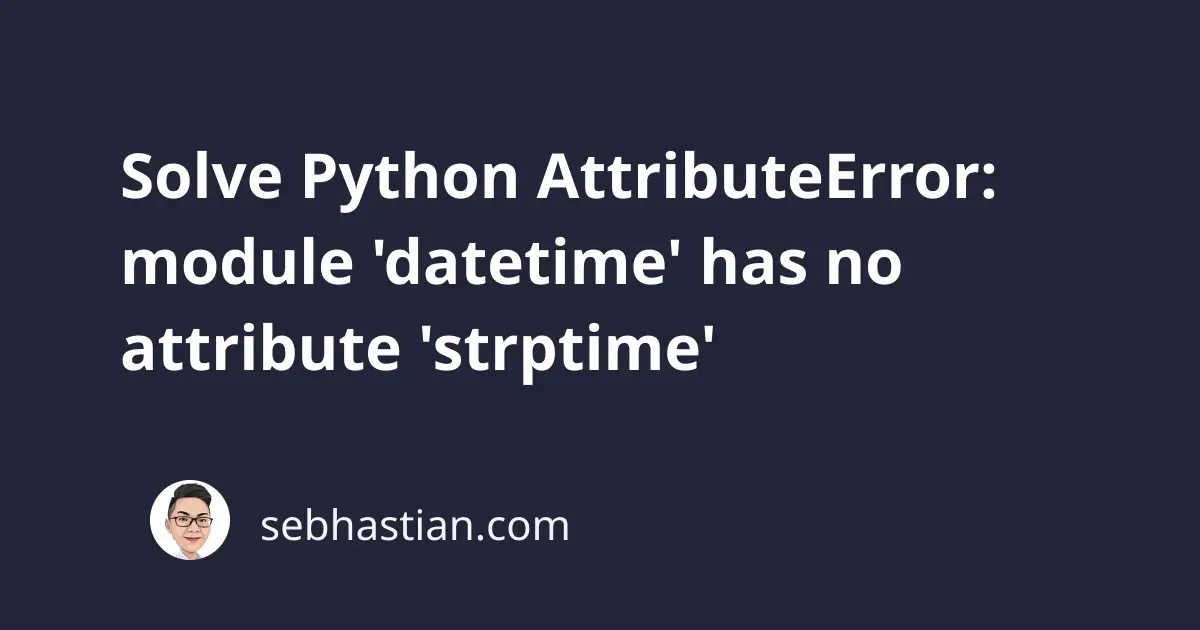
Python shows the AttributeError: module 'datetime' has no attribute 'strptime'
message when you call the strptime()
method directly from the datetime
module.
To solve this error, you need to call the strptime()
method from the datetime
class instead of the datetime
module.
The Python datetime
module has a class that’s (mind-blowingly) also named datetime
.
Suppose you have the following Python code:
import datetime
date_string = "2022-01-10"
date_object = datetime.strptime(date_string, "%Y-%m-%d")
print(date_object)
The strptime()
method is defined in the datetime
class, but the method is called from the datetime
module in the example above.
This causes the following error:
Traceback (most recent call last):
File ...
date_object = datetime.strptime(date_string, "%Y-%m-%d")
AttributeError: module 'datetime' has no attribute 'strptime'
To solve this issue, you need to call the strptime()
method from the datetime
class.
You can import the datetime
class directly from the module like this:
from datetime import datetime
date_string = "2022-01-10"
date_object = datetime.strptime(date_string, "%Y-%m-%d") # ✅
Or you can access the class from the module as follows:
import datetime
date_string = "2022-01-10"
date_object = datetime.datetime.strptime(date_string, "%Y-%m-%d") # ✅
This error confuses a lot of people because the class and the module have the same name.
To clarify your code, you can add an alias to your import
statement as follows:
import datetime as dt
date_object = dt.datetime.strptime("2022-01-10", "%Y-%m-%d")
Aliasing the datetime
module as dt
helps clear things when you are reading the code.
Some people prefer to import the datetime
class by using the from datetime import datetime
syntax.
But this import statement binds the datetime
name to the datetime
class, so you won’t be able to use other classes and functions provided by the module.
Personally, I prefer to alias the datetime
module to dt
and make all import statements identical.
When you are handling Python code written by other people, look at the import statement at the top of the file:
import datetime
? It’s the modulefrom datetime import datetime
? That’s the class.
Knowing what is being imported to the code helps you to debug and fix the error.
And that’s how you solve the AttributeError: module 'datetime' has no attribute 'strptime'
in Python. Good work! 👍