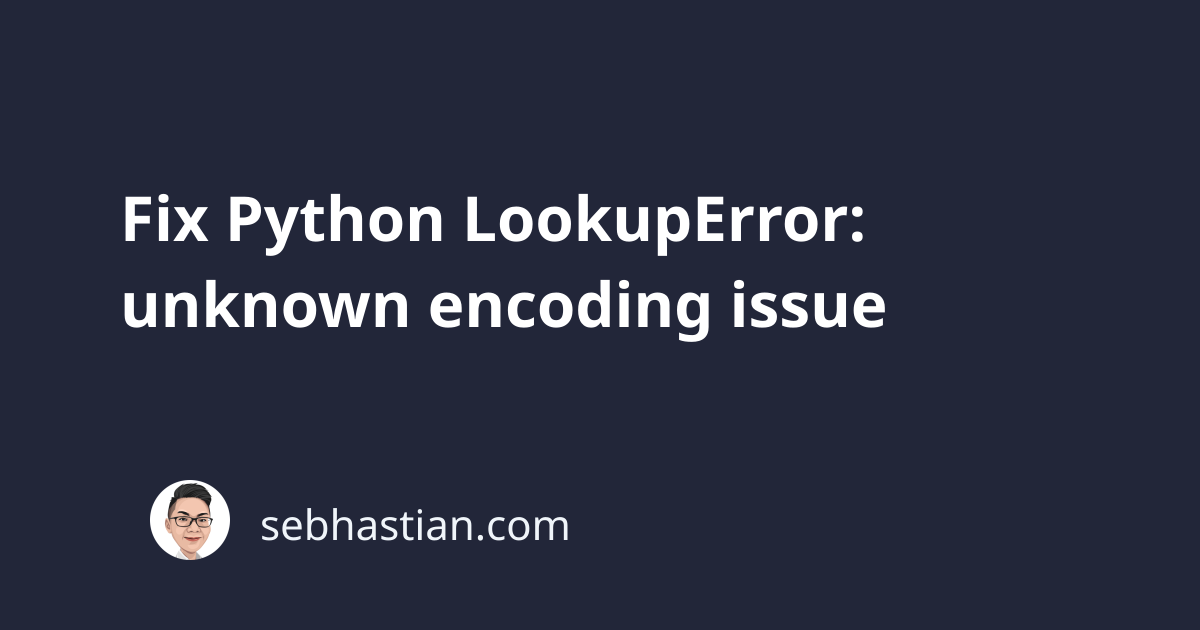
Python shows LookupError: unknown encoding when you try to encode a string using an encoding format that is not supported by Python.
To solve this error, you need to select an encoding format that’s supported by Python.
For example, suppose you try to encode a string using unicode
encoding as follows:
text = "Hello, world!"
# Try to encode to unicode
encoded_text = text.encode("unicode")
Since there’s no unicode
encoding in Python, the code above causes the LookupError as follows:
This error can occur when working with text files, streams, or any other scenario where you need to specify an encoding format.
To solve this error, you need to select an encoding format that’s officially supported by Python.
For example, you can use either utf-8
or utf-16
when encoding a string as shown below:
text = "Hello, world!"
encoded_text = text.encode("utf-16")
Also, make sure there’s no type when specifying the encoding format.
Python has several aliases for some encoding format. You can use utf8
, utf-8
, or utf_8
but you can’t use uft8
as shown below:
text = "Hello, world!"
encoded_text = text.encode("utf8") # ✅
encoded_text = text.encode("utf-8") # ✅
encoded_text = text.encode("utf_8") # ✅
encoded_text = text.encode("uft8") # ❌
Another common cause for this error is when you call the open()
function to open a file stream.
If you are passing an encoding
argument to the function, you need to make sure the format is supported by Python:
with open('output.txt', 'w', encoding='utf8') as file_obj:
file_obj.write('Python is awesome')
In the example above, we pass the named argument encoding='utf8'
to the open()
function. Since the encoding format is supported, the function runs without error.
And that’s how you solve the Python LookupError: unknown encoding issue. Nice work!