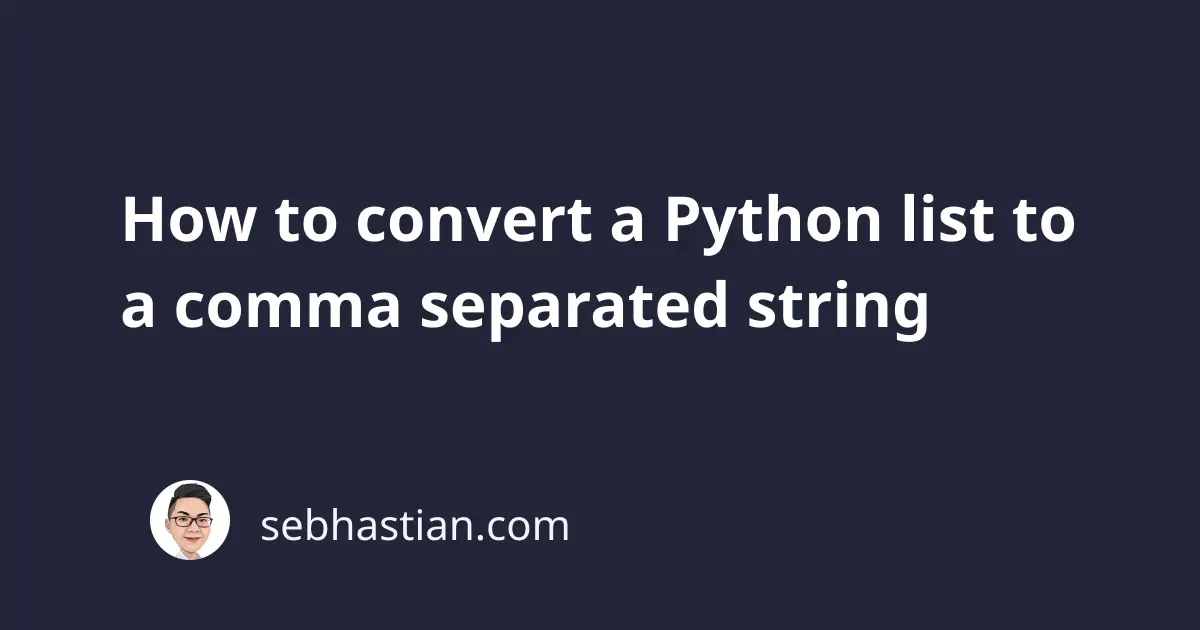
There are three methods you can use to convert a Python list to a comma-separated string:
- Using the
str.join()
method - Using a list comprehension with the
join()
method - Using the
map()
function
This tutorial will show you how to use all three methods in practice.
1. Using the str.join()
method
The str.join()
method is used to concatenate any number of strings into one string.
The string value from which you call this method will be inserted between each given string. See the example below:
my_str = "-".join(["a", "b", "c"])
print(my_str) # a-b-c
As you can see, the dash character -
is inserted between the strings passed as a list into the join()
method.
Knowing this, you can easily convert a list to a comma-separated string. You can also add a white space as follows:
my_str = ", ".join(["a", "b", "c"])
print(my_str) # a, b, c
But keep in mind that this method will fail if you have a non-string value in your list, such as an int
, a NoneType
, or a bool
.
To convert a list of mixed types, you need to use a list comprehension.
2. Using a list comprehension with the join()
method
Because the str.join()
method can’t be used on non-string values, you need to work around the limitation to convert a list of mixed types.
One way to overcome this limitation is to use a list comprehension and call the str()
function for each item to convert them to a string type.
Here’s how you do it:
my_list = ["a", "b", True, 2]
my_str = ", ".join([str(item) for item in my_list])
print(my_str) # a, b, True, 2
Here, a list comprehension is used to create a new list, and the str()
function is called for each item
in my_list
to convert them to string type.
Then, the join()
method converted the list into a comma-separated string.
3. Using the map()
function
You can also use the map()
function as an alternative to a list comprehension.
The map()
function returns an iterator containing the list items after applying a function you specified as its first argument.
You can use this function to call the str()
function for each item in the my_list
variable:
my_list = ["a", "b", True, 2]
my_str = ", ".join(map(str, my_list))
print(my_str) # a, b, True, 2
This solution works the same way as using a list comprehension, so feel free to use the method you liked best.
Conclusion
Now you’ve learned how to convert a Python list into a comma-separated string.
When you have a simple list of strings, you can use the str.join()
method. If you have a list of mixed types, you can use either a list comprehension or the map()
function.
I hope this tutorial is helpful. Happy coding! 👍