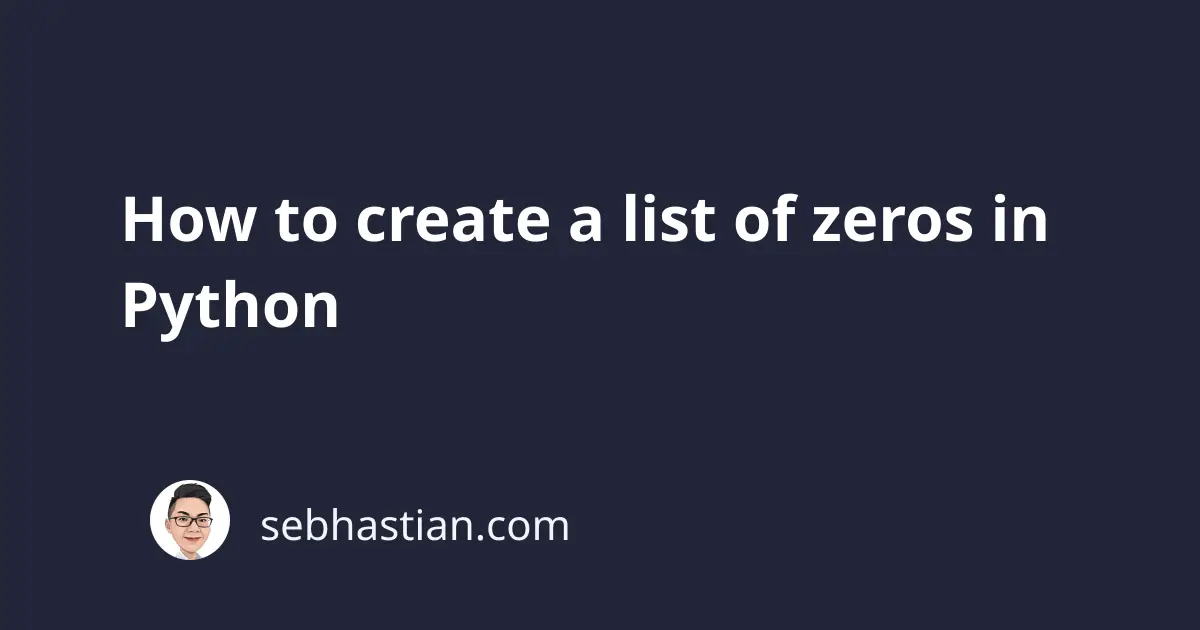
Sometimes, you might need to initialize a list with a specific length in your program.
In Python, this can be done by creating a list of zeros, which is created by multiplying a [0]
list with a number that represents the length of the list.
Here’s an example:
# List of 3
my_list = [0] * 3
print(my_list)
# List of 5
my_list = [0] * 5
print(my_list)
Output:
[0, 0, 0]
[0, 0, 0, 0, 0]
You can also save this syntax as a function so that you can use it as many times as you need:
def listofzeros(length):
return [0] * length
# Call the function and pass the length
x = listofzeros(5)
y = listofzeros(7)
print("x:", x)
print("y:", y)
Output:
x: [0, 0, 0, 0, 0]
y: [0, 0, 0, 0, 0, 0, 0]
If you’re using NumPy, then you can use the zeros()
method to create a matrix of zeros as follows:
import numpy as np
x = np.zeros((3,4), int)
print(x)
Output:
[[0 0 0 0]
[0 0 0 0]
[0 0 0 0]]
And that’s how you create a list of zeros in Python. Happy coding! 🙏