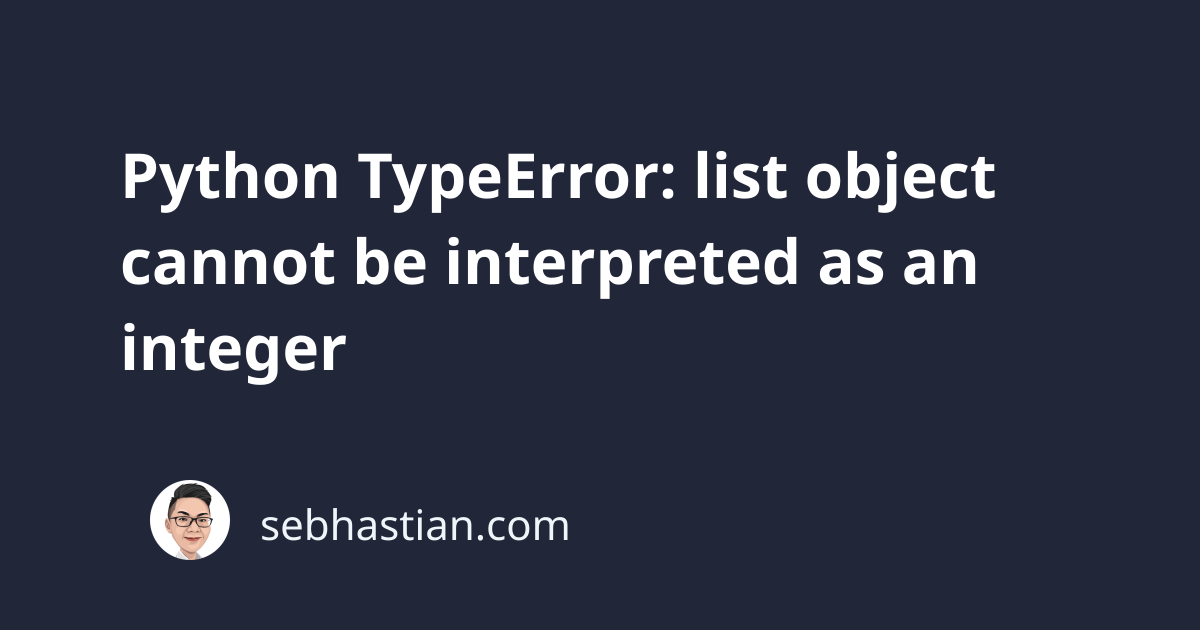
Python shows TypeError: list object cannot be interpreted as an integer
when you use a list where an integer is expected.
This article shows two common cases where this error occurs:
Pass a list to the range function
One common case where this error occurs is when you pass a list to the range
function:
# passing a list to the range function:
my_list = [1, 2, 3]
# 👇️ range expects an integer, but a list is passed
for i in range(my_list):
print(i)
The range
function can only accept an integer argument, but we passed a list in the example above.
This causes Python to respond with the following error:
Traceback (most recent call last):
File ...
for i in range(my_list):
TypeError: 'list' object cannot be interpreted as an integer
To solve this error, you need to pass an integer instead of a list to the function that causes the error.
If you want to iterate over a list with a for
loop, you can pass the list directly as follows:
my_list = [1, 2, 3]
for i in my_list: # ✅
print(i)
# 1
# 2
# 3
If you also need the index value in the for
loop, then you can use the enumerate
function.
The enumerate
function returns the index and value of a list, so you need to define two variables in the for
loop like this:
my_list = ['z', 'x', 'c']
for idx, val in enumerate(my_list): # ✅
print(idx, val)
# 0 z
# 1 x
# 2 c
The my_list
values are changed so that you can see the difference better.
The indices are 0, 1, 2
and the values are z, x, c
. The enumerate
function allows you to use the index values in your for
statement.
Pass a list to the pop function
You can also get this error when you pass a list to the list.pop
function:
my_list = [1, 2, 3]
my_list.pop([1])
Python responds with the following error:
Traceback (most recent call last):
File ...
my_list.pop([1])
TypeError: 'list' object cannot be interpreted as an integer
The pop
function accepts only an integer argument that represents the index of the element you want to pop from the list.
You need to remove the square brackets surrounding the argument as follows:
my_list = [1, 2, 3]
val = my_list.pop(1) # ✅
print(val) # 2
Keep in mind that you need to pass an integer instead of a list, not a list with integer elements.
To conclude, the Python TypeError: list object cannot be interpreted as an integer
means you are passing a list to a function that expects an integer value.
To solve this error, don’t pass a list to the range
function, and use enumerate
if you want to iterate over a list with index value included.
You also need to pass an integer to the pop
function as shown in this article.
Now you’ve learned how to solve another Python error. Nice work!