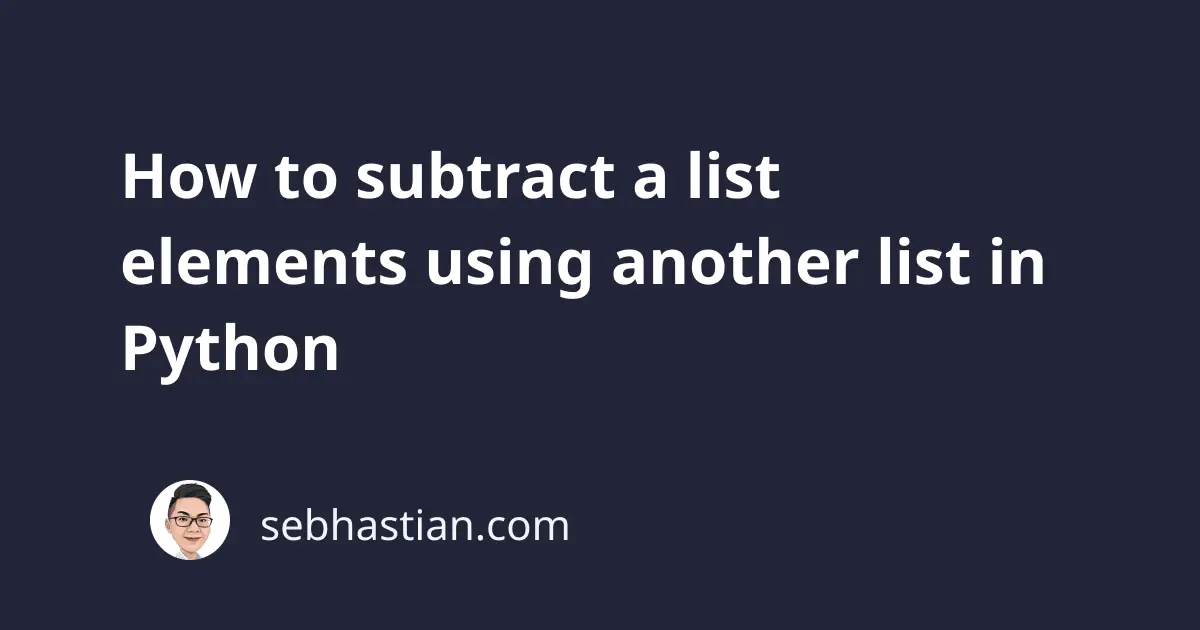
There are two ways you can subtract elements from a list using another list, depending on whether your list has duplicate values or not.
If your list has duplicate values, then you can use a list comprehension with a conditional if
statement
If your list has no duplicate values, you can transform the lists into sets, subtract the elements, and convert the result into a list.
This tutorial will show you how to use both methods in practice.
1. Using a list comprehension and a conditional
To subtract elements from one list using another list, you can use a list comprehension and a conditional if
statement.
For example, suppose you want to subtract elements from list_1
using list_2
as shown below:
list_1 = [1, 2, 3, 3, 5, 6, 7, 7, 9]
list_2 = [2, 3, 9]
# subtract elements from list_1 using list_2
result = [x for x in list_1 if x not in list_2]
print(result)
Output:
[1, 5, 6, 7, 7]
Here, the elements 2, 3, and 9 are subtracted from list_1
successfully.
This method is recommended when you have duplicate values in the original list.
2. Using set difference
The set
object in Python is able to subtract elements from a set by only using the minus -
operator.
If your list has no duplicate values, then you can convert the list to set as follows:
list_1 = [1, 2, 3, 3, 5, 6, 7, 7, 9]
list_2 = [2, 3, 9]
# subtract elements as set objects
result = set(list_1) - set(list_2)
# convert result back to list
result = list(result)
print(result)
Output:
[1, 5, 6, 7]
Notice that duplicate values are removed when you convert a list to a set.
This is because a set can’t contain duplicate values in Python.
Conclusion
This tutorial has shown you two easiest ways to subtract a list elements using another list in Python.
Use a list comprehension when you have duplicate values, or use the set()
function to convert the object and subtract using minus -
operator.
I hope this tutorial helps. See you in other tutorials!