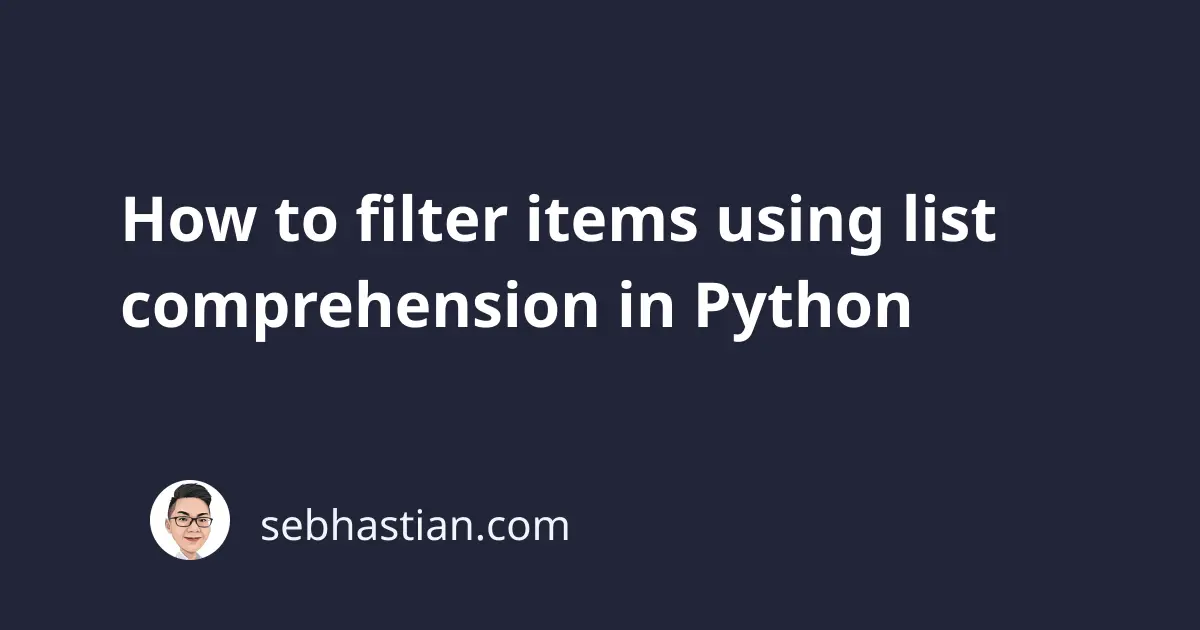
To filter what elements are added to a list, you can add an if
conditional statement when writing a list comprehension.
For example, suppose you want to include only elements that are greater than 10 in your list:
original_list = [5, 17, 12, 2, 14]
new_list = [x for x in original_list if x > 10]
print(new_list)
Output:
[17, 12, 14]
The new_list
is created using a list comprehension that has an if
statement. As a result, only elements that pass the if
test gets added to the list.
You can also add more than one condition by using an and
operator. Suppose you want to filter out even numbers that are greater than 10 from the new list:
original_list = [5, 17, 12, 2, 14]
new_list = [x for x in original_list if x > 10 and x % 2 == 1]
print(new_list) # [17]
By using an if
condition, you can filter the elements from the original_list
to fit your requirements.
I hope this tutorial is useful. Happy coding! 👋