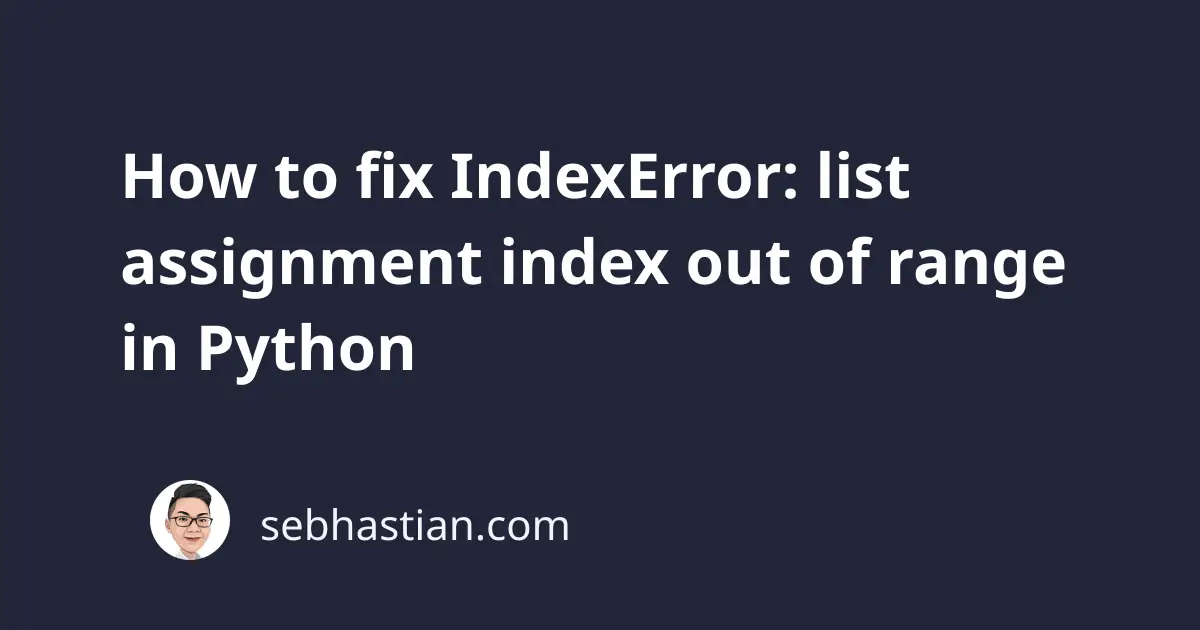
When programming with Python, you might encounter the following error:
IndexError: list assignment index out of range
This error occurs when you attempt to assign a value to a list using an index that doesn’t already exist in the list.
This tutorial will show you an example that causes this error and how to fix it in practice
How to reproduce this error
Suppose you create a list in your code as follows:
animals = ['cat', 'dog']
Next, you assign a new value at index [2]
in the list as follows:
animals[2] = 'bird'
You’ll get this error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
animals[2] = "bird"
IndexError: list assignment index out of range
The error occurs because the index number [2]
doesn’t exist in the animals
list. Index assignment in a list only allows you to change existing items.
Because the list has two items, the index number ranges from 0 to 1. Assigning a value to any other index number will cause this error.
How to fix this error
To resolve this error, you need to use the append()
method to add a new element to the list.
For example, to add the ‘bird’ item:
animals = ['cat', 'dog']
animals.append('bird')
print(animals) # ['cat', 'dog', 'bird']
animals[2] = 'cow'
print(animals) # ['cat', 'dog', 'cow']
As you can see, now the ‘bird’ value is added to the list successfully.
Adding the value using the append()
method increases the list index range, which enables you to modify the item at the new index using the list assignment syntax.
To summarize, use the append()
method when you’re adding a new element and increasing the size of the list, and use the list assignment index when you want to change an existing item in the list.
I hope this tutorial is helpful. Until next time! 👋