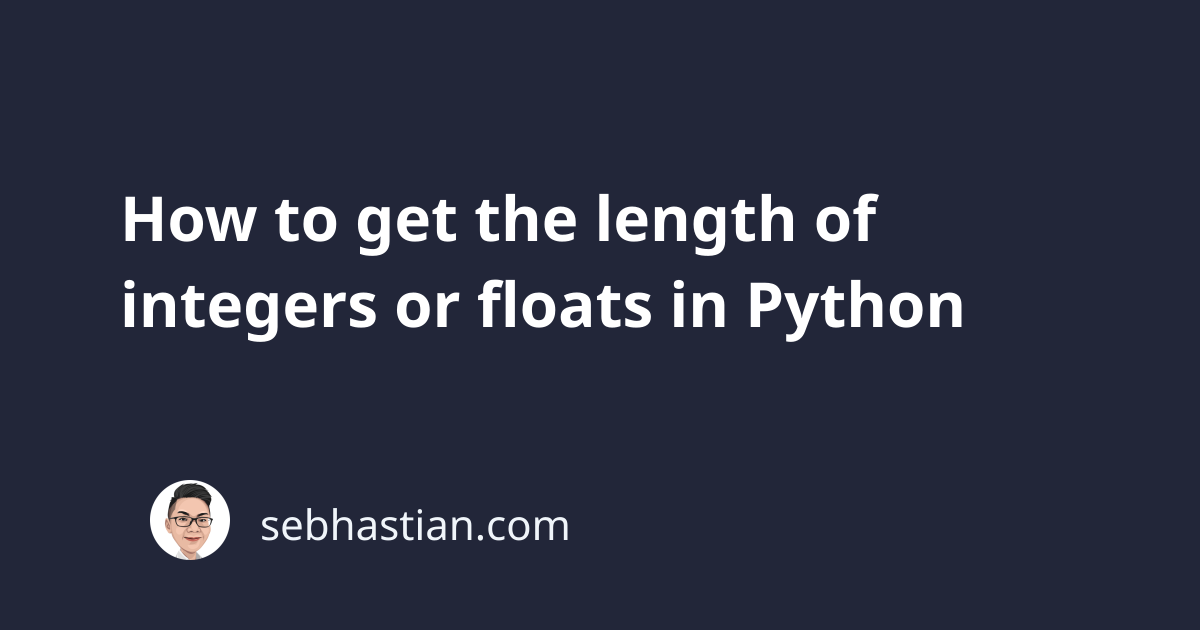
To get the length of integers or floats in Python, you need to follow these steps:
- Convert the value to string with the
str()
function - Count the length with the
len()
function - Adjust the result as you want (remove the decimal point or negative notation)
This tutorial will show you how to do the steps above in practice. Let’s start with getting the length of integer numbers.
Get the length of integer numbers
To get the length of integer numbers, you need to use the str()
and len()
functions like this:
x = 17635
# 1. Convert number to string
x_str = str(x)
# 2. Count the length
x_length = len(x_str)
print(x_length) # 5
If you have a negative number and want to remove the -
notation from the result, then you can reduce the length number by 1 or use the abs()
function to convert the number into a positive number.
See the example below:
x = -9999999999999
# 1. Call abs() and str() to create the positive string
x_str = str(abs(x))
# 2. Count the length
x_length = len(x_str)
print(x_length) # 13
And that’s how you find the length of integer numbers in Python.
You can create a reusable function if you need to find the length of many integers:
def get_int_length(num: int):
return len(str(abs(num)))
print(get_int_length(0)) # 1
print(get_int_length(99)) # 2
print(get_int_length(-9999)) # 4
Next, let’s see how you can count the length of floating numbers in Python
Get the length of float numbers
To count the length of float numbers in Python, you can use the str()
and len()
functions as shown below:
y = 17.635
# 1. Convert number to string
y_str = str(y)
# 2. Count the length
y_length = len(y_str)
print(y_length) # 6
The length of a float will include the decimal separator symbol (.
) in the counting.
If you want to exclude the decimal symbol, you can use str.replace()
to remove the dot as follows:
y = 17.635
# 1. Convert number to string
y_str = str(y)
# 2. Remove the . and count length
y_length = len(y_str.replace(".", ""))
print(y_length) # 5
The next case is that you might also have a negative floating number. If you want to exclude the negative notation, call the abs()
function when converting the float to a string.
Here’s an example:
y = -99.9922
# 1. call abs() and str()
y_str = str(abs(y))
# 2. Remove the . and count length
y_length = len(y_str.replace(".", ""))
print(y_length) # 6
As you can see, the result correctly counts only the digits from the floating number above.
You can create a function to make the code reusable:
def get_float_length(num: float):
num_str = str(abs(num))
return len(num_str.replace(".", ""))
print(get_float_length(0.14)) # 3
print(get_float_length(3.9999)) # 5
print(get_float_length(-990.9999)) # 7
Now anytime you need to count the length of a float, you just call the get_float_length()
function.
Conclusion
This tutorial has shown you how to count the length of integers and floats. The len()
function can’t be called on integers and floats, so you need to convert them to strings first.
You can adjust the result to count only the digits by using the abs()
function to remove the minus symbol from negative numbers and str.replace()
method to remove the decimal separator symbol.
I hope this tutorial is helpful. See you in the next tutorial! 👍