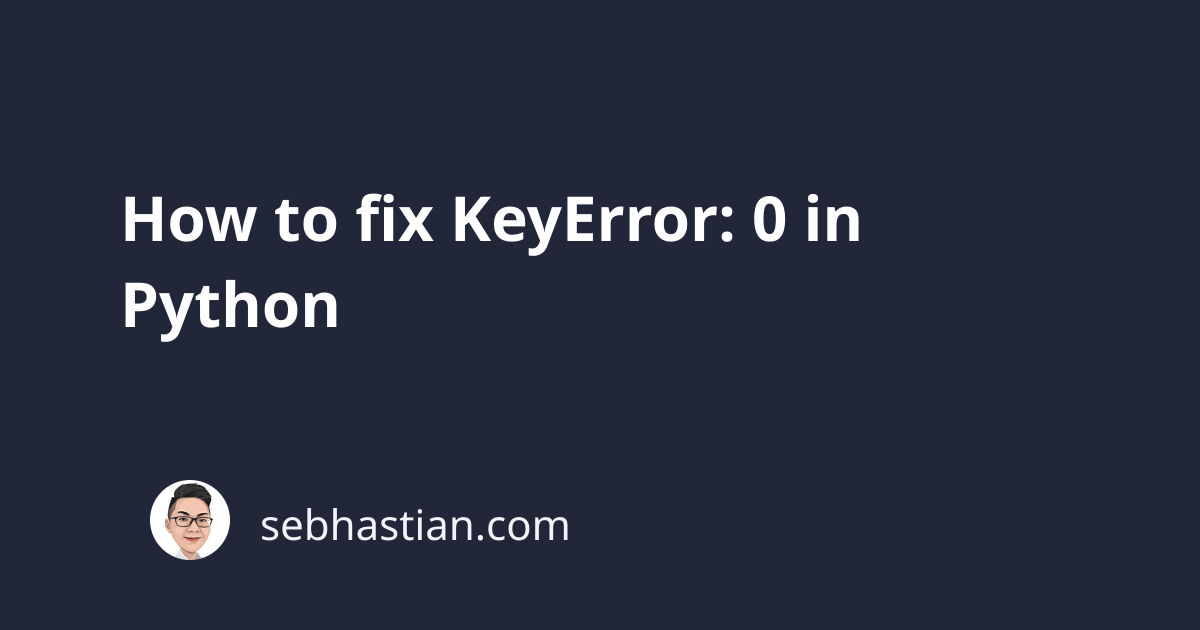
When working with a dictionary in Python, you might encounter the following error:
KeyError: 0
This error usually occurs when the key you used to access a dictionary item doesn’t exist.
There are two possible scenarios when this error might occur:
- The key you specified doesn’t exist
- You have a nested dictionary that doesn’t exist
Let’s see how to fix the error in both scenarios with the following tutorial.
1. The key you specified doesn’t exist
Suppose you create a dictionary that stores the names of fruits as follows:
fruits = {
1: "Apple",
2: "Banana",
3: "Orange"
}
Next, you want to access the item that has the key 0
as follows:
x = fruits[0]
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
x = fruits[0]
KeyError: 0
The KeyError
occurs whenever you specified a key that the dictionary doesn’t have.
The same error occurs when you pass another non-existent key as follows:
x = fruits['z'] # KeyError: z
In this case, the key z
can’t be located inside the dictionary, causing the error.
To resolve this error, you need to avoid specifying a key that doesn’t exist in the dictionary.
There are three possible solutions that could help you avoid this error.
Solution 1: Using the get() method
The dictionary object has the get()
method, which accepts two parameters:
- The
key
you want to access - The
default
value to return when that key isn’t found
This method allows you to specify a default value to return when the key you specified doesn’t exist.
Here’s an example of using the method:
fruits = {
1: "Apple",
2: "Banana",
3: "Orange"
}
x = fruits.get(0, 'Mango')
print(x) # Mango
Since the key 0
isn’t found in the fruits
dictionary, the value Mango
is returned by the get()
method.
This method is useful when you always need to return a value from accessing a dictionary object.
Solution 2: Using the if-in syntax
Another way you can avoid this error is by using the if-in
syntax.
You can check if the key exists in the dictionary like this:
fruits = {
1: "Apple",
2: "Banana",
3: "Orange"
}
if 0 in fruits:
print(fruits[0])
else:
print("Key is not found")
Using the if-in
syntax allows you to check if the key is present in the dictionary before accessing it.
You can change the if
and else
body to fit your requirements.
Solution 3: Using the try-except block
Another way you can avoid this error is by using a try-except
block so that Python doesn’t stop the program when the key isn’t found.
Here’s how you do it:
fruits = {
1: "Apple",
2: "Banana",
3: "Orange"
}
try:
print(fruits[0])
except KeyError as e:
print("Key not found:", e)
print("Code execution finished")
When the try
block fails, Python will execute the except
block and then continue down the line.
The output will be as follows:
Key not found: 0
Code execution finished
If accessing the key isn’t crucial for your program, you can skip that process and continue the code execution.
The next section will show you another scenario that causes this error.
2. You have a nested dictionary that doesn’t exist
This error also occurs when you try to create a nested dictionary using the subscript notation assignment.
For example, suppose you have an items
dictionary as follows:
items = {
'fruits' : {
1: 'Apple',
2: 'Banana'
}
}
Next, you try to add a vegetables
dictionary inside the items
dictionary as follows:
items['vegetables'][0] = 'Potato'
# KeyError: 'vegetables'
You get the same error because the vegetables
key doesn’t exist yet.
Python can’t assign the key 0
and value Potato
to a non-existing dictionary object.
To resolve this error, you need to adjust the assignment syntax and create a dictionary object in the vegetables
key first:
items['vegetables'] = {
0: 'Potato'
}
Once you created a dictionary for the vegetables
key, you can assign key-value pairs for that dictionary in the subsequent lines:
items['vegetables'][1] = 'Onion'
items['vegetables'][2] = 'Turnip'
Notice that there’s no error received when assigning the key-value pairs above. You’ve successfully created a nested dictionary called vegetables
and avoided the error.
Conclusion
The KeyError: 0
occurs in Python when you try to access the key 0
from a dictionary that doesn’t have it.
To resolve this error, you can use the get()
method, the if-in
syntax, or the try-except
block.
If you want to create a nested dictionary, you need to avoid using the subscript notation before initializing the dictionary first.
I hope this tutorial is helpful. Happy coding! 👍