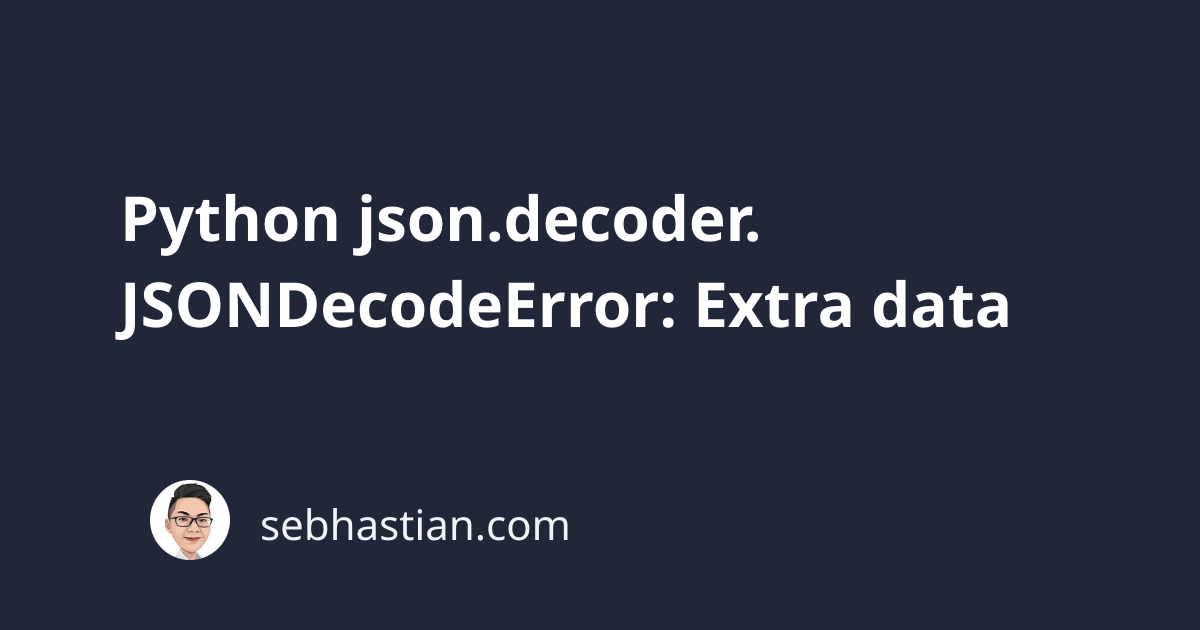
When working with JSON strings with Python, you might encounter the json.decoder.JSONDecodeError: Extra data
error message.
What JSONDecodeError: extra data mean?
A Python JSONDecodeError
means that there’s something wrong with the way your JSON data is formatted.
If the error complains about extra data
, it means that you are trying to load multiple JSON objects that are formatted incorrectly.
For example, suppose you try to load two JSON objects like this:
import json
data = '{"name": "Nathan"}, {"name": "John"}' # ❌
json.loads(data)
The call to json.loads(data)
in the example above will cause the following error:
Traceback (most recent call last):
File ...
json.loads(data)
File ...
return _default_decoder.decode(s)
File ...
raise JSONDecodeError("Extra data", s, end)
json.decoder.JSONDecodeError: Extra data: line 1 column 19 (char 18)
This error happens because the json.load()
method doesn’t decode multiple objects.
The JSON format in the example is also wrong because JSON can’t contain multiple objects as root elements.
You can validate your JSON strings using JSONLint.
How to resolve JSONDecodeError: extra data
To resolve this error, you need to wrap multiple JSON objects in a single list.
A Python list is defined using the square brackets notation ([]
) as follows. Notice how square brackets are added before and after the curly brackets:
import json
data = '[{"name": "Nathan"}, {"name": "John"}]' # ✅
str = json.loads(data)
print(str) # [{'name': 'Nathan'}, {'name': 'John'}]
How to read multiple JSON objects in Python?
If you get the JSON data from a .json
file, then you need to wrap the JSON objects written in that file in a list:
// From this:
{"name": "Nathan"},
{"name": "John"}
// To this:
[
{ "name": "Nathan" },
{ "name": "John" }
]
But keep in mind that you need to have exactly one list in a single .json
file.
If you have multiple lists like this:
[{ "name": "Nathan" }]
[{ "name": "John" }]
Then it will also trigger the JSONDecodeError: Extra data
error.
If you have exactly one JSON object per line, you can also load them using a list comprehension syntax.
Suppose your .json
file looks like this:
{ "name": "Nathan", "age": 29 }
{ "name": "John", "age": 23 }
Then you can use the list comprehension syntax to read each line and parse it as a list.
Once you have the list, you can access the JSON data as shown below:
import json
data = [json.loads(line) for line in open('data.json', 'r')]
print(data) # [{'name': 'Nathan', 'age': 29}, {'name': 'John', 'age': 23}]
print(data[0]['name']) # Nathan
print(data[0]['age']) # 29
print(data[1]['name']) # John
print(data[1]['age']) # 23
Now you’ve learned how to read JSON objects in Python.
Conclusion
Python shows json.decoder.JSONDecodeError: Extra data
error message when you try to load JSON data with an invalid format.
To resolve this error, you need to check your JSON data format. If you have multiple objects, wrap them in a list or read them line by line using a list comprehension syntax.
By following the steps in this tutorial, you can easily fix the JSON extra data error.
I hope this tutorial helps. See you again in other articles!