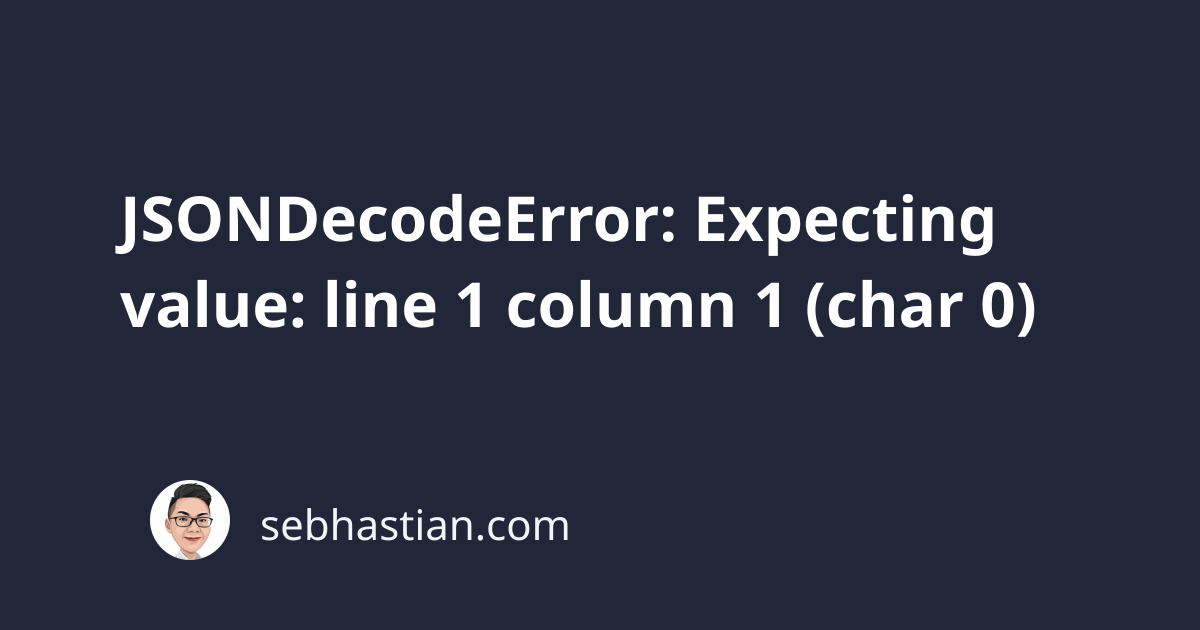
A JSONDecodeError: Expecting value: line 1 column 1 (char 0)
when running Python code means you are trying to decode an invalid JSON string.
This error can happen in three different cases:
Case 1: Decoding invalid JSON content
Case 2: Loading an empty or invalid .json
file
Case 3: A request you made didn’t return a valid JSON
The following article shows how to resolve this error in each case.
1. Decoding invalid JSON content
The Python json
library requires you to pass valid JSON content when calling the load()
or loads()
function.
Suppose you pass a string to the loads()
function as follows:
data = '{"name": Nathan}'
res = json.loads(data)
Because the loads()
function expects a valid JSON string, the code above raises this error:
Traceback (most recent call last):
File ...
res = json.loads(data)
json.decoder.JSONDecodeError: Expecting value: line 1 column 1 (char 0)
To resolve this error, you need to ensure that the JSON string you passed to the loads()
function is valid.
You can use a try-except
block to check if your data is a valid JSON string like this:
data = '{"name": Nathan}'
try:
res = json.loads(data)
print("data is a valid JSON string")
except json.decoder.JSONDecodeError:
print("data is not a valid JSON string")
By using a try-except
block, you will be able to catch when the JSON string is invalid.
You still need to find out why an invalid JSON string is passed to the loads()
function, though.
Most likely, you may have a typo somewhere in your JSON string as in the case above.
Note that the value Nathan
is not enclosed in double quotes:
data = '{"name": Nathan}' # ❌ wrong
data = '{"name": "Nathan"}' # ✅ correct
If you see this in your code, then you need to fix the data to conform to the JSON standards.
2. You’re loading an empty or invalid JSON file
Another case when this error may happen is when you load an empty .json
file.
Suppose you try to load a file named data.json
file with the following code:
with open("data.json", "r") as file:
data = json.loads(file.read())
If the data.json
file is empty, Python will respond with an error:
Traceback (most recent call last):
File ...
data = json.loads(file.read())
json.decoder.JSONDecodeError: Expecting value: line 1 column 1 (char 0)
The same error also occurs if you have invalid JSON content in the file as follows:
To avoid this error, you need to make sure that the .json
file you load is not empty and has valid JSON content.
You can use a try-except
block in this case to catch this error:
try:
with open("data.json", "r") as file:
data = json.loads(file.read())
print("file has valid JSON content")
except json.decoder.JSONDecodeError:
print("file is empty or contain invalid JSON")
If you want to validate the source file, you can use jsonlint.com.
3. A request you made didn’t return a valid JSON
When you send an HTTP request using the requests
library, you may use the .json()
method from the response
object to extract the JSON content:
import requests
response = requests.get('https://api.github.com')
data = response.json()
print(data)
But if the response
object doesn’t contain a valid JSON encoding, then a JSONDecodeError
will be raised:
Traceback (most recent call last):
...
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File ...
data = response.json()
requests.exceptions.JSONDecodeError: Expecting value: line 7 column 1 (char 6)
As you can see, the requests
object also has the JSONDecodeError: Expecting value line 7 column 1
message.
To resolve this error, you need to surround the call to response.json()
with a try-except
block as follows:
import requests
response = requests.get('https://api.github.com')
try:
data = response.json()
print(data)
except:
print("Error from server: " + str(response.content))
When the except
block is triggered, you will get the response content
printed in a string format.
You need to inspect the print output for more insight as to why the response is not a valid JSON format.
Conclusion
In this article, we have seen how to fix the JSONDecodeError: Expecting value
error when using Python.
This error can happen in three different cases: when you decode invalid JSON content, load an empty or invalid .json file, and make an HTTP request that doesn’t return a valid JSON.
By following the steps in this article, you will be able to debug and fix this error when it occurs.
Until next time, happy coding! 🙌