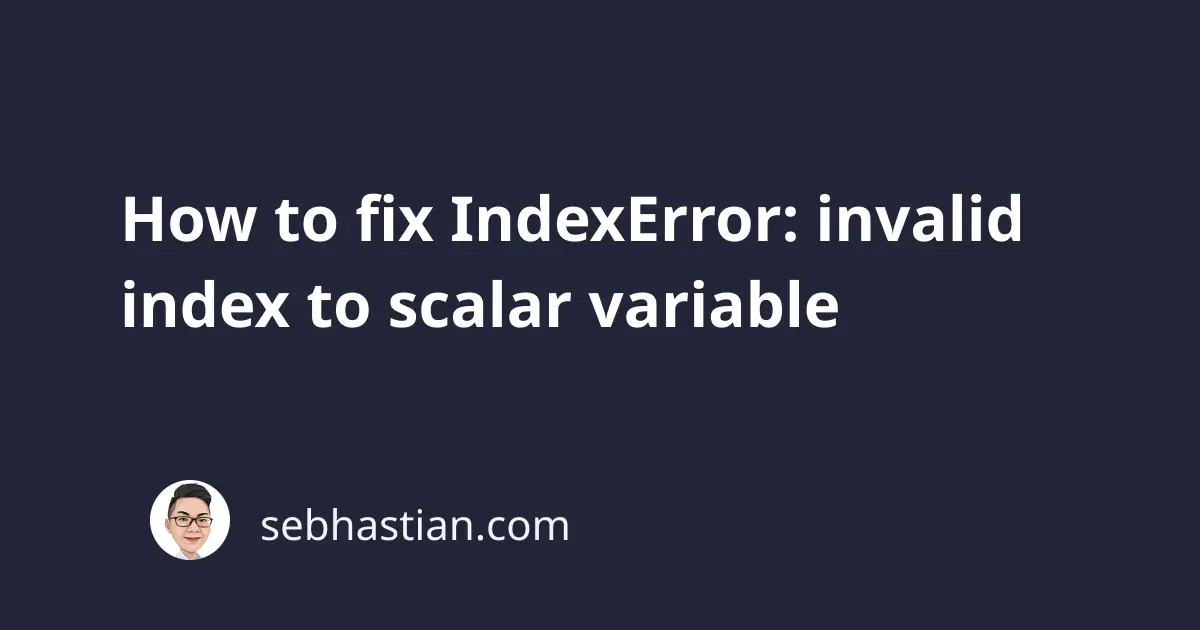
When working with the NumPy library, you might encounter this error:
IndexError: invalid index to scalar variable
This error usually occurs when you used an index to access a scalar variable.
A scalar is a variable that holds one value at a time, which is different from vectors that can hold multiple values.
This tutorial will show you examples that cause this error and how to fix it.
How to reproduce this error
Suppose you used NumPy to create an array as follows:
import numpy as np
numbers = np.array([1, 2, 3, 4])
The numbers
variable is a one-dimensional array containing int
values. It’s a vector variable because it holds multiple values.
Next, let’s say you want to access the first two items in the array, so you use the square brackets like this:
numbers[0][1]
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
print(numbers[0][0])
IndexError: invalid index to scalar variable.
The error above indicates that you’re trying to access a scalar variable, which holds only one value, with an index number.
The syntax numbers[0][1]
has one extra square brackets notation that causes this error.
Here’s another example that’s easier to understand:
import numpy as np
numbers = np.array([1, 2, 3, 4])
value = numbers[0] # 1
item = value[1] # ❌
In the above example, the value
variable is a scalar variable because it holds only one value: the first item in the numbers
variable.
In the last line, we declared the item
variable to hold the second item of the value
variable, and this causes the error.
How to fix this error
To resolve this error, you need to correct the syntax to access the items in your array.
If you want to access the first two items in the array, the correct way is to access one item at a time like this:
import numpy as np
numbers = np.array([1, 2, 3, 4])
print(numbers[0]) # 1
print(numbers[1]) # 2
# or
print(numbers[0], numbers[1]) # 1 2
Using double square brackets notation as in numbers[0][1]
only works when you have a multi-dimensional array.
Here’s an example of a two-dimensional array:
import numpy as np
pairs = np.array([[1, 2], [3, 4]])
## Access the arrays inside pairs
print(pairs[0]) # [1, 2]
print(pairs[1]) # [3, 4]
print(pairs[0][0]) # 1
print(pairs[0][1]) # 2
print(pairs[1][0]) # 3
print(pairs[1][1]) # 4
In the above example, the pairs
array consists of an array that has two child arrays.
You can access the child array’s item using double square brackets.
As long as you’re not trying to access a scalar variable with square brackets notation, this error won’t appear.
Conclusion
The error IndexError: invalid index to scalar variable
occurs when you try to access a scalar variable using the square brackets notation.
The square brackets notation is used to access items in vector variables, which can hold multiple values.
Because scalar variables can only have a single value, the fact that you’re using index numbers to access a scalar variable is what Python is complaining about.
I hope this tutorial is helpful. Happy coding! 🙏